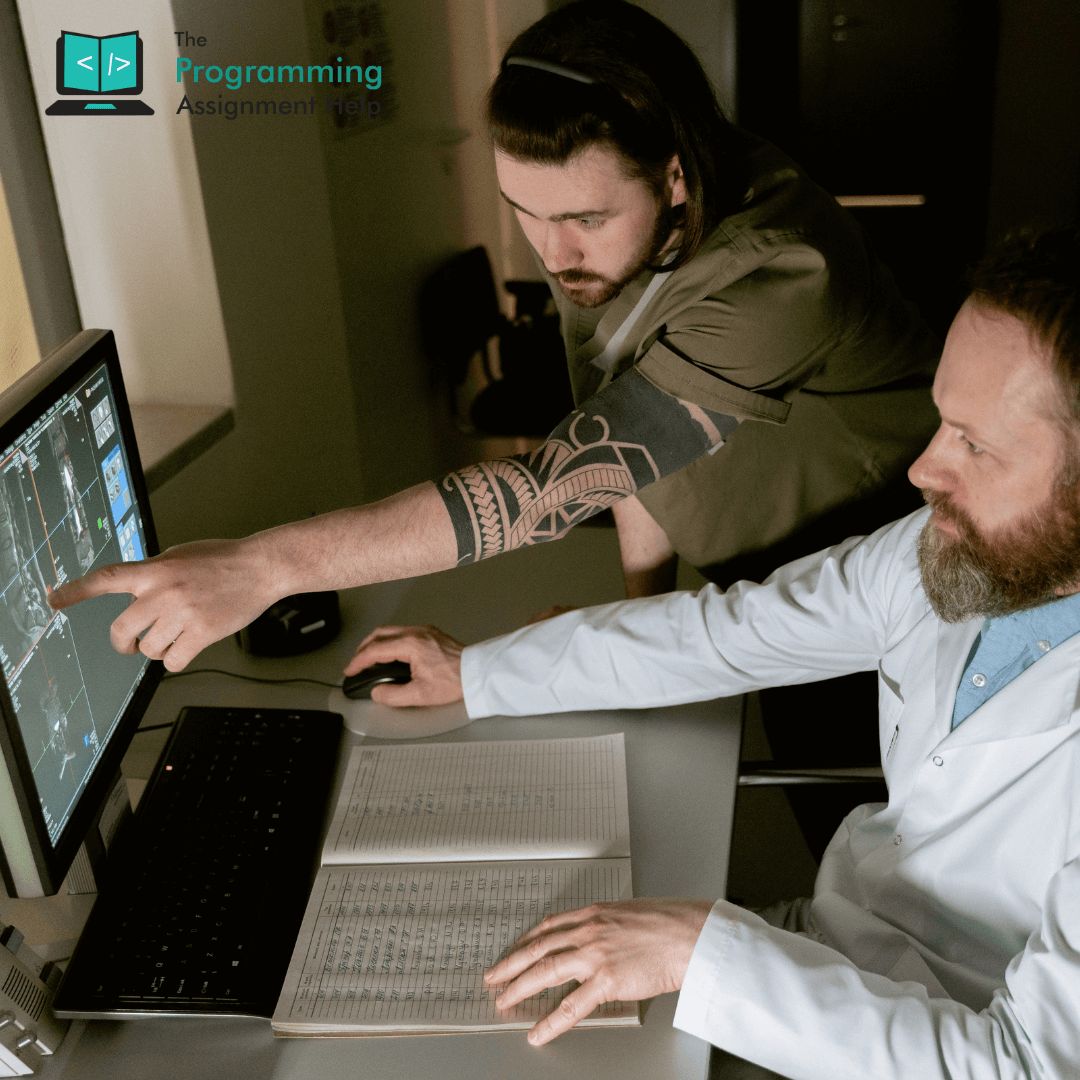
- 11th Feb 2022
- 05:04 am
- Admin
Question: We often find queues within programming and Computer Science. Using Google Scholar or a Resource Finder, find an example where a queue has been used to solve a problem.
Guidance- Write a short discussion post below discussing the example of queues you researched, outlining why a queue is the correct data structure for the problem.
Solution:
A queue is an organized group of elements where the introduction of new components takes place, at last, termed as "rear" and the deletion of old processes takes place at the far end, usually referred to as the "front" As an object reaches the queue it begins at the rear and heads towards the front, having to wait until that time when it is the succeeding item to be erased. At the later part of the compilation, the most newly acquired product in the queue must hang tight. At the front is the object which was the greatest in the compilation. This ordering principle is also known as FIFO, first-in-first-out. It is also called 1st-come-1st-served. The Lee algorithm is one feasible solution to the situation with the maze configuration. It also provides an optimal response, if there is a unique solution, but is sluggish and needs broad storage for a compact layout. It will provide the shortest path to the maze problem. The Lee algorithm is an algorithm based on broadness-first, which utilizes queues to hold the stages. Lee algorithms follow the following steps:
Step1: First we need to pinpoint or identify a starting point for the maze. After this, the initial point is added to the queue.
queue X, Y; // the queues will initialize in the matrix X.push(start_x); // initialize the queues with the start position Y.push(start_y);
Step2: In the second step, we will add all the valid neighbouring digits to the queue.
void lee() { int x, y, xx, yy; while(!X.empty()) // while there are still positions in the queue { x = X.front(); // set the current position y = Y.front();
Step3: we will remove our existing position in the queue and move to the next digit.
for(int i = 0; i < 4; i++) { xx = x + dl[i]; // travel in an adiacent cell from the current position yy = y + dc[i]; if('position is valid') //here you should insert whatever conditions should apply for your position (xx, yy) { X.push(xx); // add the position to the queue Y.push(yy); mat[xx][yy] = -1; // you usually mark that you have been to this position in the matrix } }
Step 4: Then we will repeat steps 2 and 3 until we get the whole queue gets empty.