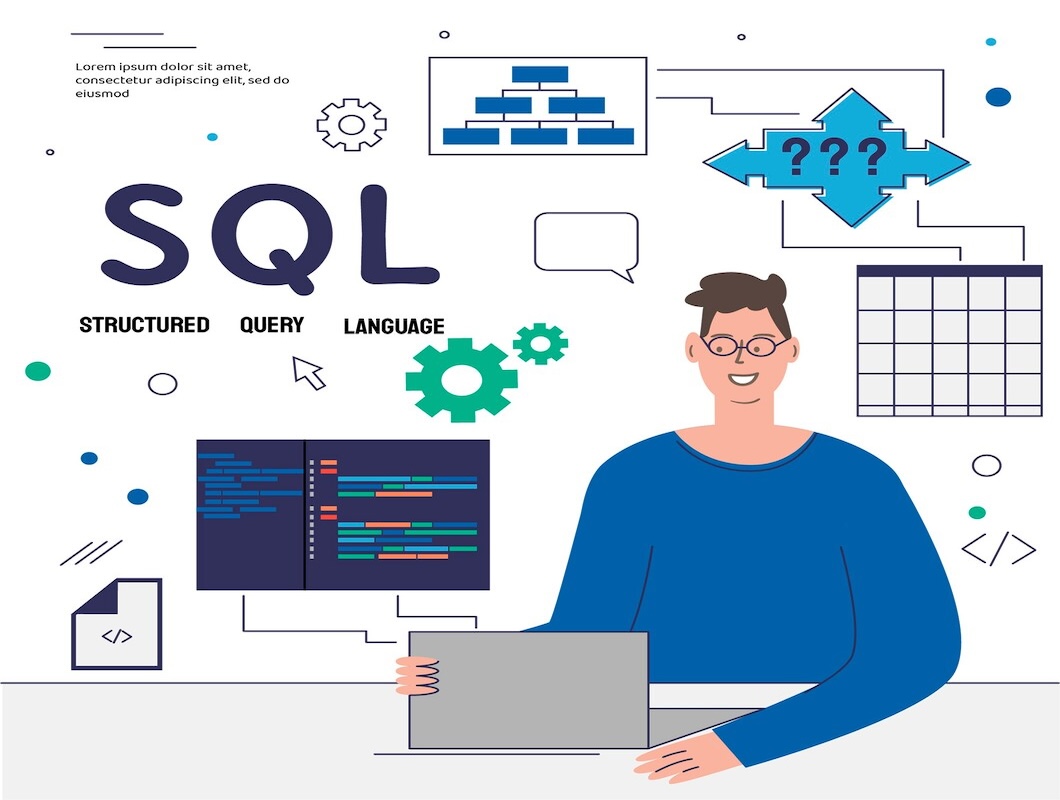
- 12th Dec 2023
- 00:22 am
- Admin
SQL statistical functions are a collection of functions that are meant to execute various statistical calculations on data stored in relational databases. These features are useful for Data Analysis, reporting, and gaining insights from enormous datasets.
SQL's statistical functions improve relational databases' ability to execute thorough statistical studies. These functions enable users to draw significant insights from within the database environment, whether they are computing averages, spotting outliers, or analysing data distributions.
Use cases of Statistical functions in SQL
SQL statistical functions are critical in data analysis and decision-making processes. These functions provide useful information on the features and distribution of data within a relational database. Here are some examples of frequent uses for statistical functions in SQL:
- Descriptive Statistics: For simple descriptive statistics, statistical functions such as AVG(), SUM(), COUNT(), MIN(), and MAX() are widely employed. They provide a fast overview of a dataset's central tendency, total sum, number of observations, and range of values.
- Data Exploration: Statistical functions are used by analysts to examine and comprehend datasets. Means, medians, and standard deviations may be calculated to uncover patterns, trends, and potential outliers.
- Performance Metrics: Statistical functions in business intelligence and reporting applications aid in the measurement of performance measures such as average sales, total revenue, and order volume. This data is essential for determining the success and efficiency of corporate operations.
- Quality Control: Statistical functions are used to monitor and control product or process quality. Calculating standard deviations or variances aids in identifying measurement discrepancies and ensuring that items satisfy set quality standards.
- Forecasting and Prediction: In predictive modelling, the correlation and covariance functions (CORR() and COVAR()) are employed to understand relationships between variables. This is essential for anticipating future trends or forecasting outcomes based on historical data.
- Random Sampling: The RAND() function generates random values, allowing the generation of random samples for testing or analysis. This is especially beneficial in cases where representative subsets of data are required.
- Percentile Analysis: Percentile methods such as PERCENTILE_CONT() and PERCENTILE_DISC() aid in the analysis of data distribution. This is useful for determining key thresholds in datasets and understanding the dispersion of values.
- A/B Testing: Statistical functions are used in A/B testing scenarios to compare changes of a process or product. AVG() and STDDEV() functions are used to calculate the average and variability of performance measures for several groups.
- Risk Evaluation: Statistical functions aid in the assessment and quantification of risks in finance and risk management. The volatility and dependency of financial instruments are measured using the variance, standard deviation, and correlation functions.
- Data Quality Assurance: Statistical functions aid in data quality assurance by detecting abnormalities, outliers, and inconsistencies in datasets. This ensures the accuracy and dependability of the data utilised for analysis and reporting.
SQL statistical functions are powerful tools with applications in a variety of disciplines. They aid in data exploration, decision-making processes, and the overall understanding of patterns and trends in datasets. Statistical functions are critical for realising the full potential of relational databases, whether in finance, marketing, operations, or research.
Function | Description |
AVG() | Calculates the average value of a numeric column, providing a measure of central tendency for the dataset. |
SUM() | Computes the sum of values in a numeric column, useful for aggregating total amounts. |
COUNT() | Counts the number of rows or non-null values in a column, providing a measure of the dataset's size. |
MIN() | Identifies the minimum value in a column, indicating the smallest value in the dataset. |
MAX() | Finds the maximum value in a column, indicating the largest value in the dataset. |
STDDEV() | Calculates the standard deviation, providing a measure of the amount of variation or dispersion in a dataset. |
VARIANCE() | Computes the variance, which is the average of the squared differences from the mean. |
CORR() | Measures the correlation between two numeric columns, indicating the strength and direction of their linear relationship. |
COVAR() | Calculates the covariance between two numeric columns, providing a measure of their joint variability. |
PERCENTILE_CONT() | Computes a specified percentile (continuous), providing insights into the distribution of values within a dataset. |
These statistical functions are essential in SQL for analyzing and summarising data. They provide insights into the central tendency, variability, and linkages within datasets, allowing users to make informed decisions based on their data's features.
Create a basic SQL program that analyses a hypothetical dataset using various statistical functions. We'll use a table called'sales' with columns for 'product_id' and'sales_amount' in this example. We'll figure out the average sales, total sales, and number of items sold.
```
-- Create a sample table
CREATE TABLE sales (
product_id INT,
sales_amount DECIMAL(10, 2)
);
-- Insert sample data
INSERT INTO sales (product_id, sales_amount) VALUES
(1, 100.50),
(2, 150.75),
(3, 80.25),
(1, 120.00),
(2, 90.50);
-- Analyze the dataset using statistical functions
SELECT
AVG(sales_amount) AS average_sales,
SUM(sales_amount) AS total_sales,
COUNT(DISTINCT product_id) AS unique_products
FROM sales;
```
Explanation:
- Setting the Table: We begin by creating a table called'sales' with columns for 'product_id' (the product identification) and'sales_amount' (the sales amount for each product).
- Inserting Sample Data: To replicate a dataset with sales records for various products, we insert some sample data into the sales table.
- Statistical Analysis: The SELECT statement employs the following statistical functions:
- AVG(sales_amount): Calculates the average sales amount, providing a measure of the dataset's central tendency.
- SUM(sales_amount): Computes the total amount of sales, resulting in the total income generated.
- COUNT(DISTINCT product_id): Counts the number of distinct goods sold, providing information on the product diversity in the dataset.
This program explains how to use statistical functions in SQL to analyze and summarise data. Additional statistical functions or filtering conditions can be integrated into the query to gain more thorough insights, depending on the specific requirements and features of the dataset. Statistical functions are useful tools for quickly analyzing key parameters within a dataset, facilitating quantitative decision-making processes.