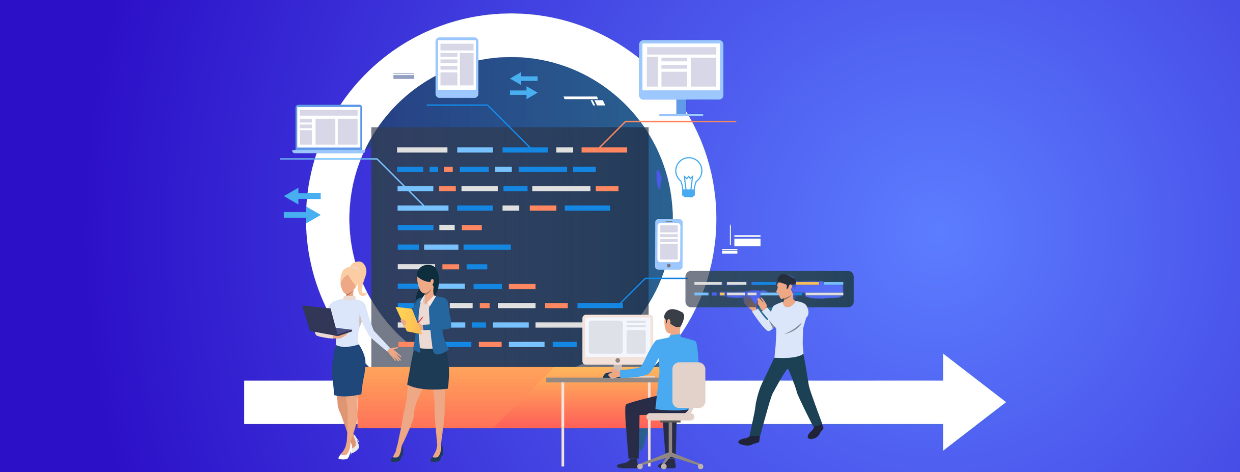
- 15th Feb 2024
- 17:00 pm
- Admin
In the world of programming, numbers don't always behave how we might expect. Imagine you're calculating your budget: you add up expenses, but the total ends with pesky decimals. Rounding down comes to the rescue, ensuring financial clarity by snapping that number to the nearest whole. But rounding down isn't just for pennies and cents; it finds applications in diverse fields like science, data analysis, and engineering.
Think of rounding down as taking a metaphorical elevator ride: you pick a floor (your desired whole number), and the number "descends" to the closest one below. This simple concept has profound implications across various domains:
- Precision in Science: When analyzing sensor data or measurements, rounding down can maintain accuracy while simplifying complex numbers. Imagine studying reaction rates - rounding down reaction times to whole seconds keeps the focus on key trends without getting bogged down in minute decimal variations.
- Clarity in Data Analysis: Working with large datasets often involves intermediate calculations that can generate unwieldy decimals. Rounding down strategic values can enhance clarity and highlight overarching patterns without sacrificing essential information.
- Reliability in Engineering: From construction dimensions to safety tolerances, rounding down plays a crucial role in ensuring precision and adherence to standards. Think of rounding down material quantities to avoid overages or rounding down error margins for added safety in critical designs.
This guide empowers you to master rounding down in Python. We'll delve into built-in functions like math.floor() and round(), explore powerful third-party libraries like numpy and pandas, and even craft custom solutions for specific needs. By the end, you'll be able to confidently navigate the world of "rounded down" numbers, tackling real-world challenges with clarity and precision.
Built-in Methods for Rounding Down
When it comes to numerical calculations, precision is often crucial, but sometimes simplification takes center stage. Rounding down offers a clean way to eliminate unwanted decimals, improving readability and focusing on the core information. In Python, several built-in methods empower you to tackle this task effectively. Let's explore three powerful tools:
- math.floor():
This dedicated function serves as your go-to choice for rounding down. Its syntax is straightforward:
import math
rounded_down_value = math.floor(number)
Remember, number here can be either an integer or a floating-point number. Watch the examples:
print(math.floor(10.75)) # Output: 10
print(math.floor(-5.2)) # Output: -6
print(math.floor(12)) # Output: 12 (no change for integers)
As you can see, math.floor() always returns the largest integer less than or equal to the input. However, keep in mind its limitations:
a. It only works with numbers, not strings or other data types.
b. For extremely large numbers, precision issues might arise due to internal computations.
- round() with Negative Precision
The round() function offers versatility, and rounding down is no exception. By using negative precision, you can achieve the desired effect:
rounded_down_value = round(number, -1)
Here's how it works in practice:
print(round(10.75, -1)) # Output: 10
print(round(-5.2, -1)) # Output: -10
print(round(12, -1)) # Output: 10 (rounds to nearest multiple of 10)
While convenient, this approach has a readability drawback. Using round() for rounding down might not be immediately clear compared to dedicated methods like math.floor().
- operator.floordiv() for Precise Integer Division
This operator comes in handy when dealing with integer division and rounding down:
import operator
rounded_down_quotient = operator.floordiv(numerator, denominator)
Remember, both numerator and denominator should be integers. Here are some examples:
print(operator.floordiv(10, 3)) # Output: 3
print(operator.floordiv(-5, 2)) # Output: -3
print(operator.floordiv(12, 4)) # Output: 3
operator.floordiv() excels in maintaining precision for integer calculations. However, be aware of potential overflow issues for very large numbers that exceed the limits of your system's integer type.
Choosing the Right Tool:
Each method has its strengths and limitations. When integers and straightforward rounding down are involved, math.floor() reigns supreme. For quick rounding with other data types, round() with negative precision can be convenient. However, for precise integer division and calculations, operator.floordiv() stands out.
Remember, understanding these built-in methods empowers you to effectively round down in Python, opening doors to cleaner data analysis, financial calculations, and other numerical tasks. By exploring their nuances and choosing the right tool for the job, you'll conquer the world of rounded numbers with confidence.
Third-Party Libraries for Advanced Rounding
While built-in methods offer solid foundations for rounding down, complex projects often demand more advanced solutions. Luckily, Python's vibrant ecosystem provides powerful libraries like NumPy and pandas, empowering you to tackle sophisticated rounding tasks with ease.
- NumPy
NumPy shines when dealing with large datasets represented as NumPy arrays. Its floor() function operates element-wise, efficiently rounding down each value in the array:
import numpy as np
data = np.array([10.75, -5.2, 12.0])
rounded_data = np.floor(data)
print(rounded_data) # Output: [ 10. -6. 12.]
But NumPy's magic doesn't stop there. Vectorized operations allow you to round entire arrays in a single line, significantly boosting performance compared to looping through elements:
large_array = np.random.rand(100000) # Create a large random array
rounded_array = np.floor(large_array)
# Vectorized vs. loop performance comparison (example)
import timeit
loop_time = timeit.timeit(lambda: [math.floor(x) for x in large_array], number=1000)
vectorized_time = timeit.timeit(lambda: np.floor(large_array), number=1000)
print("Loop time:", loop_time, "Vectorized time:", vectorized_time)
This demonstrates the significant speed advantage of NumPy's vectorized approach for large datasets.
- pandas:
pandas reigns supreme when working with tabular data. Its Series.floor() and DataFrame.floor() methods seamlessly round entire columns or DataFrames. Like NumPy, these methods handle missing values gracefully, replacing them with NaN by default:
import pandas as pd
data = pd.DataFrame({'col1': [10.5, -3.8, 12.2], 'col2': [2.7, 4.1, None]})
rounded_df = data.floor()
print(rounded_df)
Output:
col1 col2
0 10.0 NaN
1 -4.0 NaN
2 12.0 NaN
pandas also allows you to apply custom rounding functions using the apply() method, offering unparalleled flexibility for specific rounding needs.
Exploring Other Options:
While NumPy and pandas often cover most rounding needs, specialized libraries like decimal exist for high-precision rounding requirements. Remember, the best choice depends on your specific project and data characteristics. Don't hesitate to explore different options to find the perfect fit.
Custom Functions for Tailored Rounding
While built-in methods and libraries offer a robust rounding arsenal, specific scenarios often call for customized solutions. That's where custom functions come in, empowering you to define your own rounding rules and tailor them to your unique needs.
When Do Custom Functions Shine?
Imagine you're calculating taxes, where rounding down might not always be ideal. Perhaps you need to round down to the nearest multiple of 5 cents, or maybe certain items have specific rounding rules. Built-in methods don't offer this level of granularity. This is where custom functions provide the answer.
Building Your First Custom Rounder:
Let's craft a basic function that rounds down to the nearest multiple of a user-defined value:
def custom_round_down(number, multiple):
"""Rounds down to the nearest multiple of a specific value.
Args:
number: The number to be rounded.
multiple: The value to which the number should be rounded.
Returns:
The rounded down number.
"""
remainder = number % multiple
if remainder == 0:
return number
else:
return number - remainder
print(custom_round_down(10.75, 3)) # Output: 9
print(custom_round_down(15.2, 0.5)) # Output: 15
This function uses modular division and conditional statements to achieve the desired rounding behavior. By accepting arguments, it becomes adaptable to various scenarios.
Beyond the Basics:
Custom functions unlock the potential for complex rounding logic. Here are some examples:
- Round down to different values based on number ranges (e.g., round down to tens for large numbers, but units for smaller ones).
- Implement specific rounding rules for negative numbers.
- Incorporate error handling to gracefully handle invalid input.
Modularity and Reusability:
Remember, good code is reusable and maintainable. When defining custom functions, prioritize:
- Clear function names and docstrings: Explain the purpose and usage of your function.
- Meaningful variable names: Enhance code readability.
- Modularity: Break down complex logic into smaller, modular functions.
- Testing: Ensure your function behaves as expected with various inputs.
By following these principles, you'll create well-structured and reusable custom rounding functions, empowering you to tackle even the most intricate rounding challenges in your Python projects.
Error Handling and Considerations:
While rounding down simplifies calculations, neglecting potential pitfalls can lead to unexpected results. Here's how to navigate common errors and performance aspects:
Error Checkpoints:
- Data type mismatches: Ensure your functions accept compatible data types (e.g., no rounding strings!). Implement checks to prevent issues and provide informative error messages.
- Precision limitations: Remember that floating-point numbers have inherent limitations. Be aware of potential rounding errors, especially when dealing with very small or large numbers.
- Invalid input: Validate user input or function arguments to catch errors early and avoid unexpected behavior.
Graceful Error Handling:
- try-except blocks: Employ these to gracefully handle potential errors, providing meaningful messages and preventing program crashes. This enhances debugging and user experience.
Performance Considerations:
- Built-in methods: Generally optimized for performance, especially when dealing with basic rounding tasks.
- NumPy's vectorized operations: Offer superior performance for large datasets, leveraging efficient array-wise calculations.
- Custom functions: Can introduce potential performance overhead. Profile your code to identify bottlenecks and optimize if necessary.
Applications of Rounding Down
Rounding down isn't just an academic exercise - it plays a crucial role in various real-world applications across diverse fields:
Financial Calculations:
- Taxes: Rounding down taxable income or sales amounts ensures accurate tax calculations and avoids overpayments.
- Budgeting: Simplifying expenses by rounding down helps create realistic budget plans and track spending effectively.
- Price Adjustments: Businesses use rounding down to set clear and attractive price points, influencing consumer perception.
Data Analysis and Science:
- Data Cleaning: Rounding down irrelevant decimals in large datasets can improve storage efficiency and reduce processing time without compromising key insights.
- Error Propagation: When analyzing measurements with inherent error margins, rounding down can maintain accuracy while presenting results in a clear and concise manner.
- Statistical Analysis: Rounding down specific values can sometimes simplify statistical calculations or visualizations without significantly affecting the overall interpretation.
Engineering and Construction:
- Safety Margins: Rounding down tolerances or safety factors ensures adherence to critical standards and minimizes potential risks.
- Material Quantities: Estimating and ordering materials using rounded down values helps avoid excessive material costs and waste.
- Design Specifications: Rounding down dimensions in technical drawings simplifies communication and ensures practical implementation.
Other Domains:
- Inventory Management: Rounding down stock levels can facilitate efficient ordering and avoid unnecessary inventory accumulation.
- Sports and Games: Scoring systems in various sports often involve rounding down points or time measurements for clarity and fairness.
- Time Tracking: Rounding down clocked hours for billing purposes can streamline administrative processes.