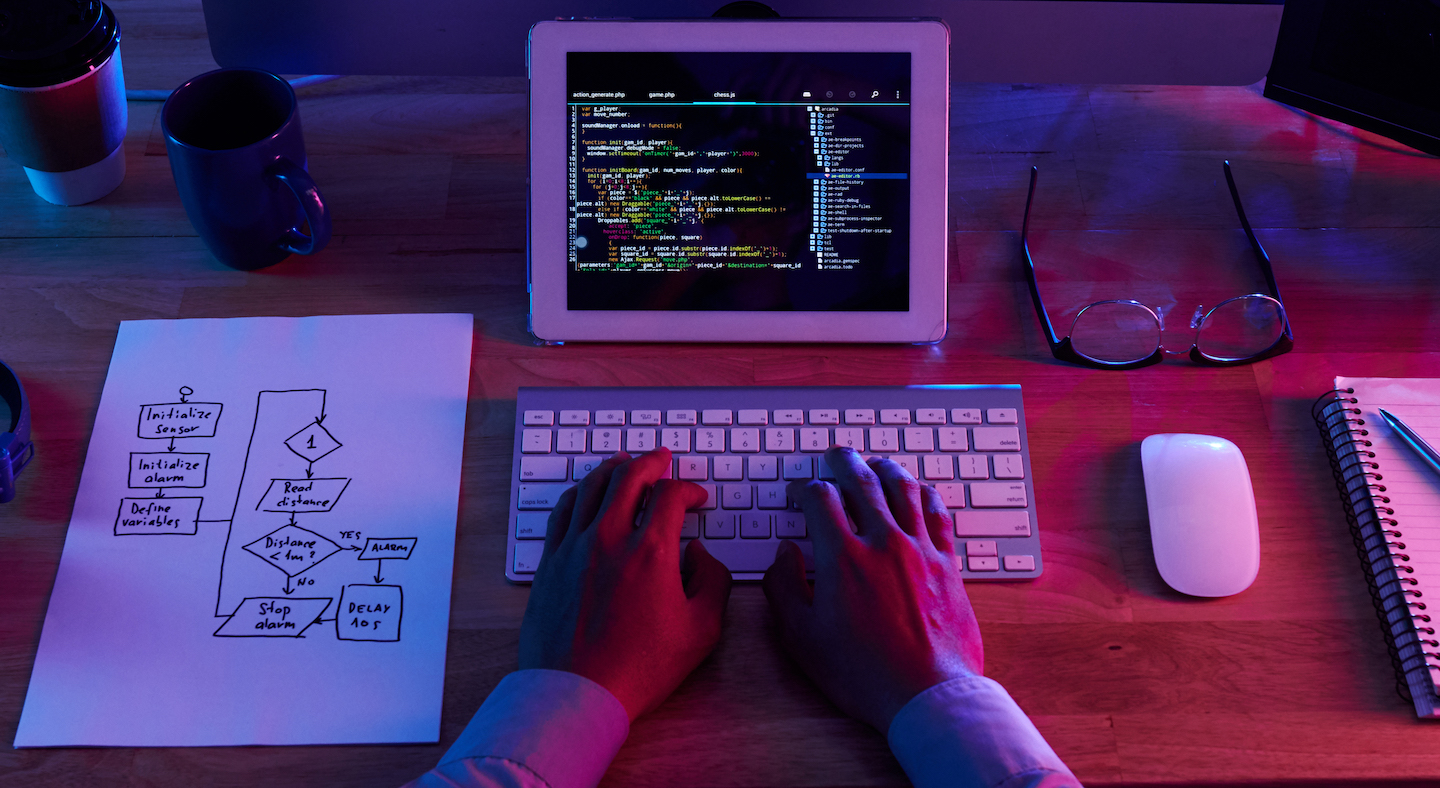
- 18th Dec 2023
- 16:42 pm
- Admin
A 'Queue' in Java is like a line where things get added at one end and taken out from the other. It follows the rule of First-In-First-Out (FIFO), meaning the one that's been there the longest is the first to go.
The 'Queue' is part of the Java Collections Framework and extends the 'Collection' interface. It helps organize data in a specific order. It defines methods for adding, deleting, and examining queue entries. The following are some of the important methods defined in the 'Queue' interface:
- 'add(E e)' and 'offer(E e)': Adds an element to the queue's back end. If the operation fails, the 'add' function throws an exception, whereas 'offer' returns a special result ('true' or 'false').
- 'remove()' and 'poll()': Removes and returns the first member in the queue. If the queue is empty, the'remove' function produces an error, whereas 'poll' returns 'null'.
- 'element()' and 'peek()': Retrieves the element at the front of the queue but does not delete it. If the queue is empty, the 'element' method throws an error, whereas 'peek' returns 'null'.
The Java standard library includes multiple implementations of the 'Queue' interface, including:
- 'LinkedList': This interface implements both the 'List' and the 'Queue' interfaces. It implements the 'Queue' using a doubly-linked list.
- 'PriorityQueue': A priority queue based on a priority heap is implemented. Elements are arranged either by their natural order or by a given comparator.
- 'ArrayDeque': An array-based implementation of the 'Deque' interface that additionally extends the 'Queue' interface. It enables for quick insertion and removal from both ends.
Queues are frequently utilized in a variety of circumstances, including work scheduling, breadth-first search methods, and concurrent programming job management.
Operations on Queue
Queues in Java provide numerous core operations that help with element management using the First-In-First-Out (FIFO) principle. The following are the fundamental Queue operations:
- Enqueue (Add):
Enqueue is the process of adding an element to the back of the queue. This process is carried out in Java utilizing methods such as 'add(E e)' or 'offer(E e)'.
```
Queue queue = new LinkedList<>();
queue.add("Element 1");
queue.offer("Element 2");
```
- Dequeue (Remove):
Dequeue is the process of removing and returning an element from the front of a queue. This action is carried out in Java utilizing methods such as'remove()' and 'poll()'.
```
Queue queue = new LinkedList<>();
queue.add("Element 1");
String removedElement = queue.poll();
```
- Peek (Inspect):
Peeking is the act of inspecting the element at the front of the queue without deleting it. This procedure is carried out in Java utilizing methods such as 'element()' or 'peek()'.
```
Queue queue = new LinkedList<>();
queue.add("Element 1");
String frontElement = queue.peek();
```
Note: It's essential to check whether the queue is empty before calling `element()` or `peek()` to avoid exceptions.
- Size:
Obtaining the number of elements in the queue is a common operation. In Java, the `size()` method is used for this purpose.
```
Queue queue = new LinkedList<>();
int size = queue.size();
```
- Check if Empty:
Checking whether the queue is empty is crucial before performing certain operations. In Java, the `isEmpty()` method can be used.
```
Queue queue = new LinkedList<>();
boolean isEmpty = queue.isEmpty();
```
These actions offer the fundamental capability for handling Queue components in Java. Different implementations of the Queue interface (e.g., 'LinkedList', 'PriorityQueue', 'ArrayDeque') may have subtle differences in functionality or new functions.
Characteristics of Queue
Queues in Java have various properties that determine their behavior and usage as part of the Java Collections Framework. The following are the major features of a Queue:
- FIFO (First-In-First-Out) Sequence: A queue's primary feature is that entries are processed in the order in which they are added. Following the FIFO principle, the element that has been in the queue the longest is the first to be eliminated.
- Linear Data Structure: A Queue is a linear data structure with components ordered in a sequential order. Existing elements are removed from one end (rear/tail) and new elements are introduced to the other (front/head).
- Addition at Rear, Removal at Front: Elements are added to the queue's back (or tail), and elements are removed from the front (or head). This guarantees that the oldest piece gets deleted first.
- Dynamic Size: Queues can be dynamically resized to accommodate components that are added or withdrawn. The size of a queue might change depending on the actions executed.
- Restricted Access: Queues often allow restricted access to components. Elements may be added to the back and taken away from the front. Elements in the center of the queue have limited or no direct access.
- Implemented in the Collections Framework: The Queue interface in Java is part of the Java Collections Framework. It enhances the Collection interface by adding queue-specific functions.
- Commonly Used actions: Enqueue (adding of items to the rear), dequeue (removal of elements from the front), peek (viewing the element at the front without removal), and testing if the queue is empty are all common actions on queues.
- Different Types: Java offers various ways to use the Queue, like LinkedList, PriorityQueue, and ArrayDeque. Each type has its own features and best uses.
- Concurrency Suppor: In the 'java.util.concurrent' package, there are special versions of queues, like 'LinkedBlockingQueue' and 'ArrayBlockingQueue'. They're made to deal with many tasks simultaneously, especially in situations where multiple actions are happening at the same time.
Understanding these characteristics helps in the selection of the suitable type of queue and its efficient use in various contexts. Queues are often employed in a variety of applications, such as work scheduling, order processing, and breadth-first search methods.
Advantages of Queues in Java
- FIFO Order: Ensures orderly processing.
- Efficient for Waiting Lines: Useful in scenarios where elements wait for processing.
- Task Scheduling: Ideal for managing tasks in a sequential manner.
- Breadth-First Search (BFS): Facilitates BFS traversal in graphs.
- Efficient for Multithreading: Concurrent implementations provide thread-safe access.
- Dynamic Size: Can dynamically adjust size based on operations.
- Multiple Implementations: Various implementations offer flexibility.
Disadvantages of Queues in Java
- Limited Access: Limited direct access to elements in the middle.
- No Random Access: Elements can only be added at the rear and removed from the front.
- Fixed Capacity: Some implementations have a fixed capacity, limiting dynamic growth.
- Inefficient for Random Access: Accessing elements in the middle is inefficient.
- Overhead: Implementations may have additional overhead compared to simpler data structures.
- Limited Use Cases: Not suitable for scenarios where random access is critical.
- Complexity: Certain operations may have higher time complexity, especially in some scenarios.
It is critical to select a queue solution depending on the application's unique requirements. Depending on criteria such as access patterns, concurrency requirements, and the nature of the activities being done, different implementations may have significant advantages and drawbacks.
Java program that uses a queue to simulate a basic task scheduler
```
import java.util.LinkedList;
import java.util.Queue;
public class TaskScheduler {
public static void main(String[] args) {
// Create a queue to simulate a task queue
Queue taskQueue = new LinkedList<>();
// Enqueue tasks
taskQueue.add("Task 1");
taskQueue.add("Task 2");
taskQueue.add("Task 3");
// Dequeue and process tasks
while (!taskQueue.isEmpty()) {
String currentTask = taskQueue.poll();
processTask(currentTask);
}
}
private static void processTask(String task) {
System.out.println("Processing: " + task);
// Simulate task processing (e.g., by printing the task)
}
}
```
Explanation:
- Queue Initialization:
We create a `Queue` using the `LinkedList` implementation. This queue (`taskQueue`) will simulate a task queue.
```
Queue taskQueue = new LinkedList<>();
```
- Enqueue Tasks:
We enqueue (add) tasks to the queue using the `add` method.
```java
taskQueue.add("Task 1");
taskQueue.add("Task 2");
taskQueue.add("Task 3");
```
- Dequeue and Process Tasks:
We use a `while` loop to dequeue (remove) tasks from the front of the queue and process them until the queue is empty.
```
while (!taskQueue.isEmpty()) {
String currentTask = taskQueue.poll();
processTask(currentTask);
}
```
- Process Task Method:
- The `processTask` method simulates the processing of a task. In this example, it just prints the task, but in a real application, this method could perform more complex operations.
```
private static void processTask(String task) {
System.out.println("Processing: " + task);
}
```
This program demonstrates using a queue in Java to handle jobs one after the other. It follows the rule of "First-In-First-Out" (FIFO), so jobs are managed in the same order they were added to the queue.