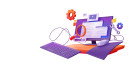
- 14th Nov 2023
- 15:00 pm
XOR, or Exclusive OR, is a fundamental logical operation in Python and many other programming languages. XOR allows you to manipulate and analyze binary data, perform bitwise operations, and solve problems in various domains.
What is Python XOR?
XOR, or Exclusive OR, is a fundamental logical operation in Python and many other programming languages. XOR allows you to manipulate and analyze binary data, perform bitwise operations, and solve problems in various domains.
- XOR Operator
Essentially, XOR, or Exclusive OR, is a logical operator used in computing. XOR works with binary values, usually represented as 0 and 1, and generates results based on a predefined rule. When the input values differ, XOR yields a result of 1, and when they are identical, it produces 0. This behavior makes XOR a key element in computer science and digital electronics.
- Performing XOR Operations in Python
Python provides a straightforward way to perform XOR operations using the caret symbol (^). The XOR operation in Python can be conducted on integers, booleans, and binary representations of data.
- How XOR Works
To comprehend the functioning of XOR, take two binary values, A and B, as an example. XOR examines each pair of bits in A and B. If the bits at a given position differ, the result will contain a 1 in that position. Conversely, if the bits are the same, the result will contain a 0.
- XOR in Bitwise Operations
XOR plays a fundamental role in Python's bitwise operations, enabling the manipulation of individual bits within integers. In addition to XOR, other essential bitwise operations like AND, OR, and NOT empower developers to perform intricate bit-level manipulations. These operators are useful for tasks like setting and clearing specific bits in binary numbers.
Built-in XOR Function
Python provides a convenient built-in XOR function, simplifying the XOR operation. This function accepts two arguments and computes the XOR result between them. This method removes the necessity for complex bit manipulation, presenting a simple way to execute XOR operations. The XOR operation returns True if the number of True operands is odd, which makes it a valuable tool for evaluating boolean conditions. Let's explore how the XOR operator functions:
- XOR Syntax and Implementation in Python
The XOR operator is denoted by the caret symbol (^). It accepts two operands, and its syntax is as follows::
result = operand1 ^ operand2
The XOR operator returns True if only one of the operands is True, and it returns False if both operands have the same boolean value (either both are True or both are False).
- Using the Built-in XOR Function
Python includes a built-in function, bool(), that inherently performs XOR operations. When given two arguments, if one evaluates to True and the other to False, bool() returns True. Let's explore this built-in XOR functionality with some illustrative examples:
XOR with Boolean Values
result = bool(True) ^ bool(False)
# Evaluates to True
In this example, the XOR operator ^ combines the boolean values True and False. Since one is True and the other is False, the result is True.
XOR with Integer Values
result = bool(1) ^ bool(0)
# Evaluates to True
Here, we have integer values, 1 and 0. By converting them to booleans using bool(), the XOR operation yields True.
XOR with Complex Conditions
condition1 = True
condition2 = False
condition3 = True
result = bool(condition1) ^ bool(condition2) ^ bool(condition3)
# Evaluates to False
This example demonstrates XOR applied to complex conditions. The XOR operator can chain together multiple conditions. However, it returns False because there is an even number of True values (2), which doesn't meet the XOR criterion.
Performing XOR Operations with NumPy
NumPy, a fundamental library for numerical computations in Python, provides a robust framework for performing element-wise XOR operations on arrays. Leveraging the power of NumPy, you can apply XOR on arrays, which is incredibly useful in data manipulation, data masking, and various other applications.
- Using NumPy to XOR Arrays
To XOR two arrays, you can utilize NumPy's bitwise XOR function, often represented as ^. This function allows you to apply element-wise XOR to corresponding elements of two arrays of the same shape. The result is a new array with the XOR results.
Here's how to perform a basic XOR operation on two arrays using NumPy:
import numpy as np
array1 = np.array([1, 0, 1, 0])
array2 = np.array([1, 1, 0, 0])
result = np.bitwise_xor(array1, array2)
- XOR on Multidimensional Arrays
NumPy further simplifies XOR operations on multidimensional arrays. You can perform the XOR operation on entire arrays or specific rows and columns, which proves beneficial when working with image processing, data transformation, and more.
import numpy as np
matrix1 = np.array([[1, 0, 1], [0, 1, 0], [1, 0, 1]])
matrix2 = np.array([[0, 1, 0], [1, 0, 1], [0, 1, 0]])
result = np.bitwise_xor(matrix1, matrix2)
In this case, result will be a NumPy array representing the element-wise XOR of matrix1 and matrix2.
XOR for Efficient Variable Swapping
The XOR operator provides an efficient method to swap the values of two variables without requiring additional storage. This XOR-based variable swapping operation is both concise and elegant.
The XOR-Based Variable Swapping Technique
Traditional variable swapping often involves the use of a third temporary variable, but XOR simplifies this process. Here's how it works:
- Swapping Variables Using XOR:
Swapping values of two variables, "a" and "b," without the need for a third variable can be accomplished using the XOR operator as illustrated below:
a = 7
b = 5
a = a ^ b
b = a ^ b
a = a ^ b
Upon completing these operations, variable "a" will contain the original value of variable "b," while variable "b" will hold the initial value of "a." The XOR-based variable swapping technique leverages XOR's unique properties and is a space-efficient solution.
- A Space-Efficient Alternative
The XOR-based variable swapping technique is particularly useful in scenarios where memory resources are limited, or you want to optimize your code for efficiency. By eliminating the need for an extra storage location, it streamlines variable swapping.
- An Application Beyond Simple Swapping
Beyond its role in variable swapping, XOR has applications in diverse fields, including cryptography, network security, and data manipulation. Understanding and utilizing XOR's unique characteristics can enhance your problem-solving capabilities in various programming scenarios.
Error Handling in Python XOR
While XOR is a valuable operator, it's important to be aware of potential errors that may arise during its use.
- Error Handling for Data Type Compatibility
XOR operations should ideally involve boolean or integer values. When using XOR with incompatible data types, you might encounter errors. To ensure the smooth execution of XOR operations, it's advisable to validate input data types and handle exceptions where necessary.
- Handling XOR-Related Exceptions
In Python, invalid XOR operations may raise exceptions. These exceptions are essential to catch and handle appropriately, preventing unexpected program crashes and ensuring the reliability of your code.