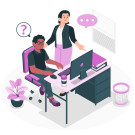
- 2nd Nov 2023
- 23:14 pm
- Admin
What is Python Tuple
A Python tuple serves as an organized data structure enabling users to store multiple items within a single variable. Unlike lists, tuples have a unique quality - they're immutable, signifying that once a tuple is formed, its content remains unaltered. Tuples are characterized by round brackets and are versatile, allowing for various data types like integers, strings, and even other tuples to be included. They are commonly used for grouping related pieces of data. There are 4 types of built-in data types in Python which include Tuples, List, set, and dictionary every data type has its unique qualities and usage types.
In this tutorial, we'll dive deeper into understanding tuples in Python and explore their various uses.
How a Tuple is defined in Python
- A tuple can be defined using round braces ().
- At least one item is required in a tuple.
- A Tuple constructor is required to create a tuple.
Example of A tuple in Python
Var = (“mango”,”apple”,”grapes”,”banana”)
print(var)
Output : (mango, apple, grapes, banana)
// Here we can name variable according to the type of tuple
Tuples in Python are versatile and can indeed hold multiple data types, including both strings and integers simultaneously. Their versatility comes in handy across a spectrum of applications, especially when you require grouping diverse data types into a structured collection.
Example:
Var = (“3”,”2”,”Red”,”Green”)
Print (3,2,Red,Green)
Uses of Python Tuples
Tuples in Python have several use cases due to their immutability and ordered nature. Here are five main uses of tuples in Python:
- Data Integrity: Tuples find frequent use in safeguarding data integrity, primarily due to their immutability, which serves as a shield against unintended data alterations. For example, you can use tuples to store coordinates (x, y) that should remain constant.
- Multiple Values Return: Functions can return multiple values as a tuple. This is useful when you need to return more than one piece of information from a function in a structured manner.
- Dictionary Keys: Since tuples are immutable, they can be used as keys in dictionaries. This is handy for creating custom, hashable keys for dictionary entries.
- Variable Swapping: Tuples make it easy to swap the values of two variables without the need for a temporary variable. This is often used in algorithms and for efficient variable value exchanges.
- Iterating Over Sequences: Tuples are useful when you want to iterate over multiple sequences (e.g., lists) in parallel. You can combine tuples with functions like `zip()` to iterate over corresponding elements from different sequences simultaneously.
Tuples provide a way to structure and manage data in a way that complements lists and other data structures, and their immutability can offer advantages in specific situations.
Immutability and Tuple Methods
- Explaining the Concept of Immutability
Now, let's explore the concept of immutability in a bit more detail. In Python, immutability signifies that once you've brought an object into existence, it's essentially carved in stone, incapable of undergoing any changes. Tuples, firmly categorized as immutable, strictly adhere to this principle – once a tuple takes shape, there's no inserting, deleting, or tweaking of elements permitted within its structure. This unwavering immutability stands as a cornerstone of tuples, serving a pivotal role in upholding data integrity. This makes tuples useful when you have data that should remain constant, like coordinates or configuration settings.
- Discussing Common Tuple Methods: count() and index()
Python provides some built-in methods for working with tuples. Two common methods are:
- `count()`: This method allows you to count the number of occurrences of a specific element within a tuple. For example, if you have a tuple of numbers and you want to know how many times a particular number appears, you can use the `count()` method.
- `index()`: The `index()` method helps you find the index of the first occurrence of a specific element in a tuple. It's particularly useful when you want to know the position of an element in the tuple.
- Demonstrating How to Work with Immutable Data
When you're dealing with immutable data, such as tuples, it calls for a different approach when compared to working with mutable data structures like lists. If you wish to introduce alterations to a tuple, you need to craft a fresh tuple that incorporates the desired adjustments. This can involve creating copies of the original tuple and reassigning values. Understanding this process is essential for effectively utilizing tuples.
- Hands-On Exercises for Using Tuple Methods
To help students grasp the concept of tuple methods, you can create hands-on exercises. For instance, you can provide a tuple with a mix of data types and ask students to use the `count()` and `index()` methods to perform specific tasks. These exercises reinforce the practical usage of these methods and help students gain confidence.
Use Cases and Best Practices
Discussing use cases and best practices is crucial to help students understand when and why to use tuples. Real-world examples, such as storing coordinates, representing fixed configuration settings, or using tuples as dictionary keys, can illustrate the value of tuples. It's also essential to compare tuples with lists, explaining when one is more appropriate than the other based on the requirements of a particular programming task. Best practices in choosing data structures should be emphasized to help students make informed decisions in their coding projects.
These explanations, discussions, and exercises should provide a well-rounded introduction to tuples in Python, and students will gain a solid understanding of this essential data structure.
Real-world examples of When to Use Tuples
Tuples find their place in various real-world scenarios. Here are some practical examples of when to use tuples:
- Geographic Coordinates: Tuples prove excellent for depicting geographic coordinates, such as latitude and longitude. These values are fixed and should not be changed during calculations or processing.
- Date and Time Information: Tuples can store date and time components (year, month, day, hour, minute, second) effectively, making them useful for timestamp data.
- Stock Information: When you need to store data about a stock, a tuple can hold information like stock symbol, price, and trading volume. This data doesn't change within the tuple.
- Employee Records: Tuples can represent employee records with fixed attributes like name, employee ID, and hire date.
Comparing Tuples with Other Data Structures like Lists
Tuples and lists share certain similarities, yet they fulfill distinct roles. Let's draw a comparison:
Tuples:
- Immutable (cannot be changed after creation).
- Use parentheses for creation.
- Ideal for storing fixed, unchanging data.
- Typically faster for iteration and access.
Lists:
- Mutable (can be changed after creation).
- Use square brackets for creation.
- Suitable for dynamic collections of data.
- More versatile for adding, removing, or modifying elements.
Best Practices for Choosing Between Tuples and Lists
When choosing between tuples and lists, consider the following best practices:
- Use Tuples for Immutable Data: If the data should remain constant and unchanging, use tuples.
- Use Lists for Dynamic Data: If you need to modify the data frequently, choose lists.
- Consider Performance: Tuples are generally faster for iteration and access, so for read-heavy operations, they may be more efficient.
- Think About Semantics: Choose the data structure that best represents the semantics of your data. Tuples are often used for fixed, structured data.
Practical Coding Examples Showing the Advantages of Tuples in Specific Scenarios
Here are two practical coding examples that highlight the advantages of tuples:
- Example 1: Calculating the Distance Between Two Points
Suppose you want to calculate the distance between two points in a 2D space. Using tuples to represent the points ensures that their coordinates remain unchanged during the calculation:
```
import math
point1 = (3, 4)
point2 = (6, 8)
distance = math.sqrt((point2[0] - point1[0]) 2 + (point2[1] - point1[1]) 2)
print(distance)
```
- Example 2: Storing Configuration Settings
Tuples can be used to store configuration settings for a program, ensuring that these settings are constant throughout its execution:
```
# Configuration settings (immutable)
database_config = ("localhost", "mydb", "user", "password")
# Later in the code, you can access these settings without worrying about changes.
```
These examples showcase how tuples can simplify code and provide clarity in scenarios where data should not change.
These topics and examples should help students understand the practical applications and advantages of tuples in Python.