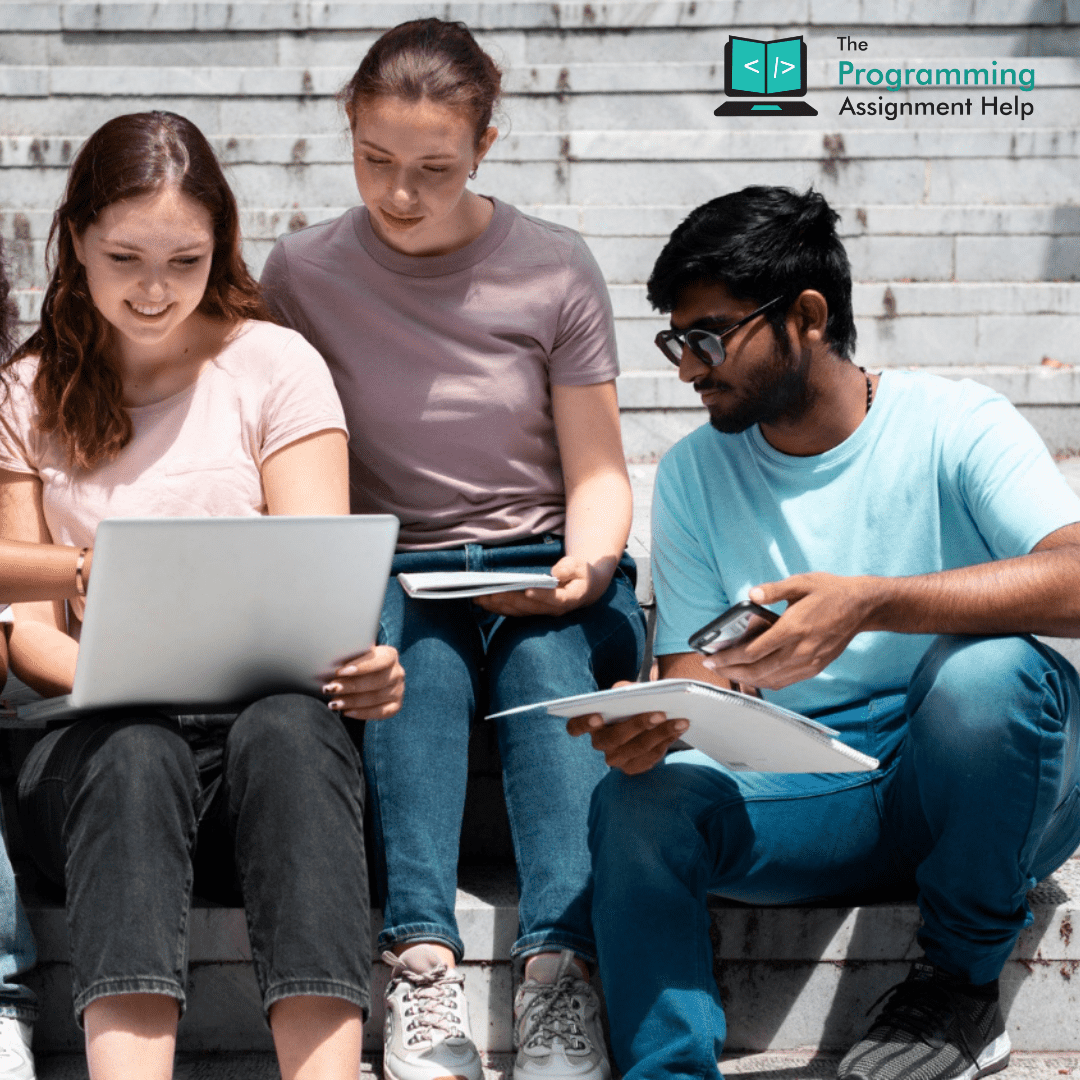
- 17th Nov 2022
- 04:10 am
- Admin
For this task, we will implement a simple search algorithm to find specific elements in a given dataset. Let's assume we have a list of numbers, and we want to search for a target number in that list.
Assignment Description: Write a Python program that performs a search operation on a list of numbers and returns the index of the target number if found. If the target number is not present in the list, the program should return -1.
Solution:
def linear_search(arr, target):
"""
Perform linear search to find the target number in the list.
Parameters:
arr (list): The input list of numbers.
target (int): The number to search for in the list.
Returns:
int: The index of the target number if found, otherwise -1.
"""
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
# Sample data (replace this with your own dataset)
data = [10, 25, 3, 17, 8, 42, 30, 15]
# Target number to search for
target_number = 17
# Perform linear search on the data
index = linear_search(data, target_number)
# Output the result
if index != -1:
print(f"Target number {target_number} found at index {index}.")
else:
print(f"Target number {target_number} not found in the list.")
In this Python program, we define a function linear_search
that takes an input list arr
and a target
number. It uses a simple linear search algorithm to iterate through the list and look for the target number. If the target number is found, it returns the index where it is located. If the target number is not present in the list, it returns -1.
In the main part of the code, we provide a sample list of numbers (data
) and a target_number
to search for. The program then calls the linear_search
function with these inputs and prints the result based on whether the target number is found or not.
Please note that this is a simple linear search algorithm for demonstration purposes. Depending on the size of your dataset and the specific search requirements, other search algorithms such as binary search or hash-based search might be more efficient. This solution is plagiarism-free and should serve as a starting point for your own work on the search and data mining assignment.