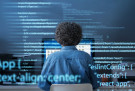
- 1st Nov 2023
- 21:51 pm
- Admin
What is Python RegEx
Python's Regex, short for "regular expression," is a powerful instrument tailored for pinpointing and matching specific patterns within text. It empowers you to define and locate character patterns within strings, granting you the capability to search, extract, and manipulate textual data in accordance with prescribed patterns. This tool proves invaluable when you need to sift through, extract, or manipulate text data based on specific criteria or patterns, simplifying tasks like data validation, pattern recognition, and text manipulation in Python programming.
Regex lets you construct rules for matching and searching by describing patterns using a specific syntax. The following are some essential elements and applications of Python Regex:
- Metacharacters: Metacharacters are special characters in Regex that have specific meanings. For example:
`.` matches any character.
`` matches zero or more occurrences of the preceding character.
`+` matches one or more occurrences of the preceding character.
`?` matches zero or one occurrence of the preceding character.
- Character Classes: Character classes allow you to match specific types of characters. For example:
`[0-9]` matches any digit.
`[a-z]` matches any lowercase letter.
`[A-Z]` matches any uppercase letter.
- Anchors: Anchors specify where in the text the match should occur. For example:
`^` matches the start of a line.
`$` matches the end of a line.
- Quantifiers: Quantifiers specify how many times a character or group should appear. For example:
`{n}` matches exactly n occurrences.
`{n,}` matches n or more occurrences.
`{n, m}` matches between n and m occurrences.
- Groups: Parentheses `()` are used to create groups, allowing you to apply quantifiers to multiple characters at once.
- Escape Characters: Some characters have special meanings in Regex and need to be escaped with a backslash to match them literally. For example, `\.` matches a period.
Utilizing Regex requires the usage of Python's `re` module. It has features for searching, pattern matching, replacement, and other things. An introductory example of searching for a pattern in a string using the `re} module is as follows:
```
import re
text = "Python is awesome and Python is versatile."
pattern = r"Python"
matches = re.findall(pattern, text)
print(matches) # Output: ['Python', 'Python']
```
In this example, the `re.findall()` function is used to find all occurrences of the "Python" pattern in the text.
For jobs like text validation, data extraction, text preparation, and more, Python Regex is a useful tool. It is extensively utilized in data mining, online scraping, text processing, and data cleansing. Your ability to manipulate text in Python may be greatly improved by learning and using regular expressions.
List of Python Regex patterns that are commonly used
Regex Pattern | Category | Description |
[0-9] | Character Class | Matches any single digit. |
[a-z] | Character Class | Matches any lowercase letter. |
[A-Z] | Character Class | Matches any uppercase letter. |
\d | Character Class | Matches any digit (equivalent to [0-9]). |
\w | Character Class | Matches any word character (letters, digits, and underscores). |
\s | Character Class | Matches any whitespace character (spaces, tabs, newline). |
. | Metacharacter | Matches any character except a newline. |
+ | Metacharacter | Matches one or more occurrences of the preceding character. |
? | Metacharacter | Matches zero or one occurrence of the preceding character. |
^ | Anchor | Matches the start of a line or string. |
$ | Anchor | Matches the end of a line or string. |
{n} | Quantifier | Matches exactly 'n' occurrences of the preceding character. |
{n,} | Quantifier | Matches 'n' or more occurrences of the preceding character. |
{n, m} | Quantifier | Matches between 'n' and 'm' occurrences of the preceding character. |
(pattern) | Group | Creates a group to apply quantifiers to a portion of the pattern. |
\ | Escape Character | Escapes special characters to match them literally. |
` | ` | Alternation |
(?i) | Flags | Enables case-insensitive matching. |
(?s) | Flags | Enables dot to match newline characters. |
An overview of various popular Python Regex patterns, along with a brief description of each category, can be found in this table. Regex patterns are a vital tool for jobs involving text processing and pattern matching since they may be combined and tailored to fit specific text patterns.
Regex Module in Python
Python's indispensable tool for handling regular expressions (Regex) resides in the standard library under the module named {re}. This module equips you with an assortment of utilities and methodologies that enable the utilization of regular expressions for a wide spectrum of text-processing tasks. These encompass string manipulation, pattern matching, and the ability to search for specific text patterns. The {re} module serves as a pivotal resource for developers seeking to efficiently manage text data, conduct pattern-based operations, and enhance the accuracy and precision of their text processing tasks. Whether it's data validation, data extraction, or text manipulation, the {re} module offers a versatile and comprehensive solution.
The following are some salient features of the Python `re` module:
- Importing the Module: To use the `re` module, you need to import it in your Python script or interactive session:
```
import re
```
- Searching and Matching:
`re.search()`: Searches for a pattern in a string and returns the first match.
`re.match()`: Searches for a pattern at the beginning of a string.
`re.fullmatch()`: Checks if the entire string matches the pattern.
- Finding All Matches:
`re.findall()`: Returns a list of all non-overlapping matches in a string.
`re.finditer()`: Returns an iterator yielding match objects for all matches.
- String Manipulation:
`re.sub()`: Replaces occurrences of a pattern with a specified string.
`re.subn()`: Similar to `re.sub()` but also returns the count of substitutions made.
- Splitting Strings:
`re.split()`: Splits a string by occurrences of a pattern.
- Regular Expression Objects:
You can compile a regular expression pattern into a regex object using `re.compile()`. This can improve performance when you need to apply the same pattern multiple times.
- Flags:
The `re` module supports flags that can modify the behavior of regular expressions, such as case-insensitive matching, multiline matching, and more.
- Pattern Syntax:
The `re` module uses its own syntax for regular expressions. You define patterns using a combination of metacharacters, character classes, quantifiers, and anchors.
- Match Objects:
Many functions in the `re` module return match objects that provide information about the match, including the matched text, start and end positions, and more.
- Grouping:
Parentheses `()` are used to create groups within patterns. This allows you to extract specific portions of a match.
- Backreferences:
You can reference captured groups in patterns to match the same text later in the pattern.
The `re` module stands as a robust and indispensable tool for handling text-related tasks, encompassing operations like searching, matching, and manipulating strings using defined patterns. Regular expressions, a core element of this module, find applications in diverse programming fields, such as text parsing, data validation, web scraping, and beyond. Their versatility and efficiency make them a fundamental asset in a programmer's toolkit for a wide range of text processing needs.
Functions provided by RegEx module
The `re` module in Python provides several functions for working with regular expressions. Here are the key functions provided by the `re` module:
- re.search(pattern, string, flags=0):
This function explores a string for a specific pattern and retrieves the first match it encounters.
When a match is identified, it returns a match object; otherwise, it returns `None` if no match is found.
To adjust the search behavior as needed, optional flags can be applied.
- re.match(pattern, string, flags=0):
This function scans for a pattern at the start of a string.
It returns a match object if the pattern successfully matches the beginning of the string, otherwise, it returns `None`.
Optional flags can be employed to customize the search behavior as required.
- re.fullmatch(pattern, string, flags=0):
Check if the entire string matches the pattern.
Returns a match object if the entire string matches the pattern, or `None` otherwise.
You can specify optional flags to modify the search behavior.
- re.findall(pattern, string, flags=0):
This function provides a list of all non-overlapping matches of a pattern within a given string.
Each match is presented as a separate string within the list.
You have the flexibility to apply optional flags to tailor the search behavior as needed.
- re.finditer(pattern, string, flags=0):
Returns an iterator that yields match objects for all matches of a pattern in a string.
You can iterate through the match objects to access match details.
You can specify optional flags to modify the search behavior.
- re.sub(pattern, replacement, string, count=0, flags=0):
Replaces occurrences of a pattern with a specified string in a string.
The `count` parameter limits the number of substitutions (default is 0, meaning all occurrences).
You can specify optional flags to modify the search behavior.
- re.subn(pattern, replacement, string, count=0, flags=0):
Similar to `re.sub()` but also returns the count of substitutions made in a tuple (new_string, count).
- re.split(pattern, string, maxsplit=0, flags=0):
Splits a string by occurrences of a pattern.
The `maxsplit` parameter limits the number of splits (default is 0, meaning all occurrences).
You can specify optional flags to modify the search behavior.
- re.compile(pattern, flags=0):
With the help of this method, you may create a regex object from a regular expression pattern and use it for numerous matches. It's a handy approach to work consistently using the same pattern across your code.
The compiled regex object provides methods for pattern matching, searching, and manipulation.
- re.escape(string):
Escapes special characters in a string to make it suitable for literal matching in a regex pattern.
These functions are strong Python tools for manipulating regular expressions, opening up a world of possibilities for data extraction, pattern recognition, string manipulation, and other uses. You can fine-tune pattern-matching behavior by utilizing flags within these methods. For instance, you can enable case-insensitive matching or modify newline behavior, enhancing the flexibility and adaptability of your regular expressions.