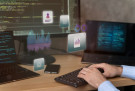
- 1st Nov 2023
- 21:24 pm
Recursion stands as a captivating and influential concept within the realm of programming, effectively employed by Python. It empowers a function to invoke itself, effectively forming a repetitive loop that adheres to specific conditions. This recursive strategy greatly simplifies intricate problems, rendering them more structured and aesthetically refined.
What is Python Recursion?
Python recursion is a sophisticated and elegant programming concept that empowers functions to call themselves, simplifying complex problems into manageable components. At its core, recursion breaks down a problem by dividing it into smaller, similar sub-problems. In Python, this technique involves a function calling itself with each successive call working on a reduced portion of the original problem. The process continues until a base case is met, at which point the function starts returning results, eventually solving the entire problem.
Recursion is a powerful tool when handling problems that can be divided into smaller, identical sub-problems. It promotes code reusability, readability, and simplification. In Python, it shines in scenarios like tree traversal, complex mathematical calculations, and pathfinding algorithms. Understanding and effectively using Python recursion is essential for solving intricate problems efficiently and elegantly.
Why Use Recursion in Python?
Recursion in Python is a valuable technique that finds its application in various problem-solving scenarios. Its utility stems from several key advantages:
- Simplifying Complex Problems: Python recursion allows you to break down complex problems into smaller, more manageable sub-problems. By tackling these sub-problems recursively, you can address the larger problem effectively.
- Code Readability: Recursive solutions are often more readable and intuitive. They mirror the natural divide-and-conquer approach that humans use to solve problems.
- Code Reusability: Recursive functions can be applied to similar sub-problems within a broader context, promoting code reusability and reducing redundancy.
- Elegance: Python recursion can lead to elegant solutions, especially in situations where iterative approaches might involve extensive loops and conditional statements.
- Applications in Data Structures: Recursion plays a vital role in tree traversal, graph algorithms, and various Data Structure operations, making it indispensable for handling advanced data structures.
Recursive Function in Python
Python leverages recursion effectively by allowing a function to call itself, breaking down intricate problems into smaller sub-problems. It comprises two fundamental elements: the base case (determining when the recursion ends) and the general case (solving complex issues by subdividing them). Python's recursive approach simplifies problem-solving, enhancing efficiency.
- Base Case: The base case acts as the recursion's ending assumption. It specifies when to end the recursive calls. The recursive function would keep calling itself endlessly without a base case. The base case represents the simplest scenario or input that the function can directly handle. Once the base case is reached, the recursion unwinds, and the function returns a result.
- General (Recursive) Case: The general case is where the recursive function calls itself with modified arguments, moving closer to the base case with each iteration. This part of the function addresses more complex scenarios or inputs, breaking them down into simpler sub-problems. The general case encapsulates the logic for dividing the problem into smaller parts and invoking the function recursively.
Types of Recursion in Python
Python supports different types of recursion:
- Direct Recursion: Direct recursion is further categorized into two subtypes, each with its unique characteristics:
- Tail Recursion: In this form of direct recursion, the recursive call is the last operation within the function. Tail recursion is well-known for its efficiency and reduced memory consumption. It doesn't need to keep track of multiple pending recursive calls, making it more memory-efficient. This type is frequently employed in functional programming paradigms and is excellent for solving problems that lend themselves naturally to a recursive approach, such as calculating factorials.
- Head Recursion: In head recursion, the recursive call takes place at the beginning of the function. Unlike tail recursion, it is less memory-efficient because each recursive call is added to the call stack, creating a stack of calls that need to be resolved. Head recursion is useful when certain operations need to be executed before the recursive call, such as updating a counter or printing results. Although it may consume more memory, head recursion is applicable when the problem-solving process requires a specific order of execution.
- Indirect Recursion: Indirect recursion entails multiple functions calling one another in a circular manner. One function starts the call, and another continues it, which can become complex. To avoid infinite loops, precise control mechanisms are needed. Indirect recursion is valuable for solving intricate problems with mutual dependencies, offering an effective approach to breaking them into smaller, interrelated subproblems.
Recursion in Python with Numbers
Recursion in Python extends its utility to a wide array of numerical problems. From testing divisibility by 7 to computing factorials, generating Fibonacci numbers, and reversing integers, Python's recursive capabilities empower programmers with elegant and efficient solutions.
- Divisibility by 7: Recursive functions can help determine if a number is divisible by 7, a task that may seem daunting, but recursion simplifies the process.
- Factorial of a Number: The process of determining the factorial of a given number entails the iterative operation of multiplication. By utilizing recursion, the execution of this task gets more simplified as it involves dividing it down into smaller subproblems.
- Nth Fibonacci Number: Generating the Fibonacci sequence can be accomplished using recursive functions. The sequence's recursive nature is apparent as each number relies on the sum of the two preceding numbers.
- Integer Reversal: Reversing an integer involves manipulating its digits. Recursive functions enable programmers to tackle this task in an organized manner, providing an effective solution for integer reversal.
Recursion in Python with Strings and Arrays
Recursion in Python is a versatile technique that goes beyond numerical problem-solving; it extends its reach into handling strings and arrays as well. The inherent recursive nature of many string and array operations lends itself naturally to recursion.
- Ascending or Not: Determining whether an array is sorted in ascending order is a classic example of a recursive function. By comparing adjacent elements, recursion can efficiently solve this problem.
- Number of Vowels: Counting vowels in a string can also be elegantly solved with recursion. The function can traverse the string character by character, identifying vowels, and incrementing the count.
- Palindrome: Recursion can check whether a given string is a palindrome, which means it reads the same forwards and backward. By comparing characters symmetrically, recursion can verify this property.
- Reversal of String: Reversing a string, much like reversing an integer, is another task made manageable through recursion. By breaking the string into smaller parts and progressively reversing them, a reversed string can be constructed.
Recursion in Python with Data Structures
Recursion in Python extends its applications beyond numbers, strings, and arrays to data structures. Python's ability to create and manipulate data structures makes it a powerful tool for solving complex problems. Two notable examples of recursion with data structures are:
- Inserting a Node at the End and Traversing a Linked List Using Recursion: Linked lists can be effectively managed using recursion. You can create recursive functions to insert nodes at the end or traverse the entire list. By defining base cases and recursive steps, you can navigate and modify linked lists with ease.
- Inorder Traversal of Binary Tree: Recursion plays a fundamental role in binary tree traversals. For example, the inorder traversal visits nodes in ascending order, making it particularly useful for sorting. By recursively traversing the left subtree, visiting the current node, and then the right subtree, you can achieve this essential operation.
Advantages and Disadvantages of Recursion in Python
Recursion is a powerful tool with clear advantages in terms of elegance, readability, and problem-solving. However, it should be used judiciously, considering performance and potential pitfalls like stack overflows. Careful design, proper base cases, and efficient termination conditions are essential for successful recursive implementations.
Advantages:
- Simplicity: Recursion can offer elegant and concise solutions to problems. It often reflects the mathematical or problem-solving logic directly.
- Divide and Conquer: Recursion divides complex problems into smaller, more manageable subproblems. This technique is notably efficacious for jobs that necessitate the division of an issue into analogous, yet smaller, components.
- Readability: Recursive code can be highly readable and close to human thought processes, making it accessible to other programmers.
- Versatility: Recursion can be applied to various domains, from mathematics to data structures, offering a universal problem-solving tool.
Disadvantages:
- Stack Overflows: The inclusion of recursive calls contributes to the accumulation of function calls on the call stack, potentially resulting in stack overflow issues if not appropriately handled, especially in scenarios involving extensive or infinite recursion.
- Performance: Recursive methods may exhibit worse efficiency compared to iterative approaches in some problem scenarios, particularly in terms of memory utilization.
- Debugging Complexity: Debugging recursive code can be challenging, as you must trace through multiple recursive calls, which can be difficult to visualize.
- Limited Tail Recursion Optimization: While Python supports tail recursion, it doesn't optimize it as some other languages do. This can result in stack overflows in functions that utilize tail recursion.