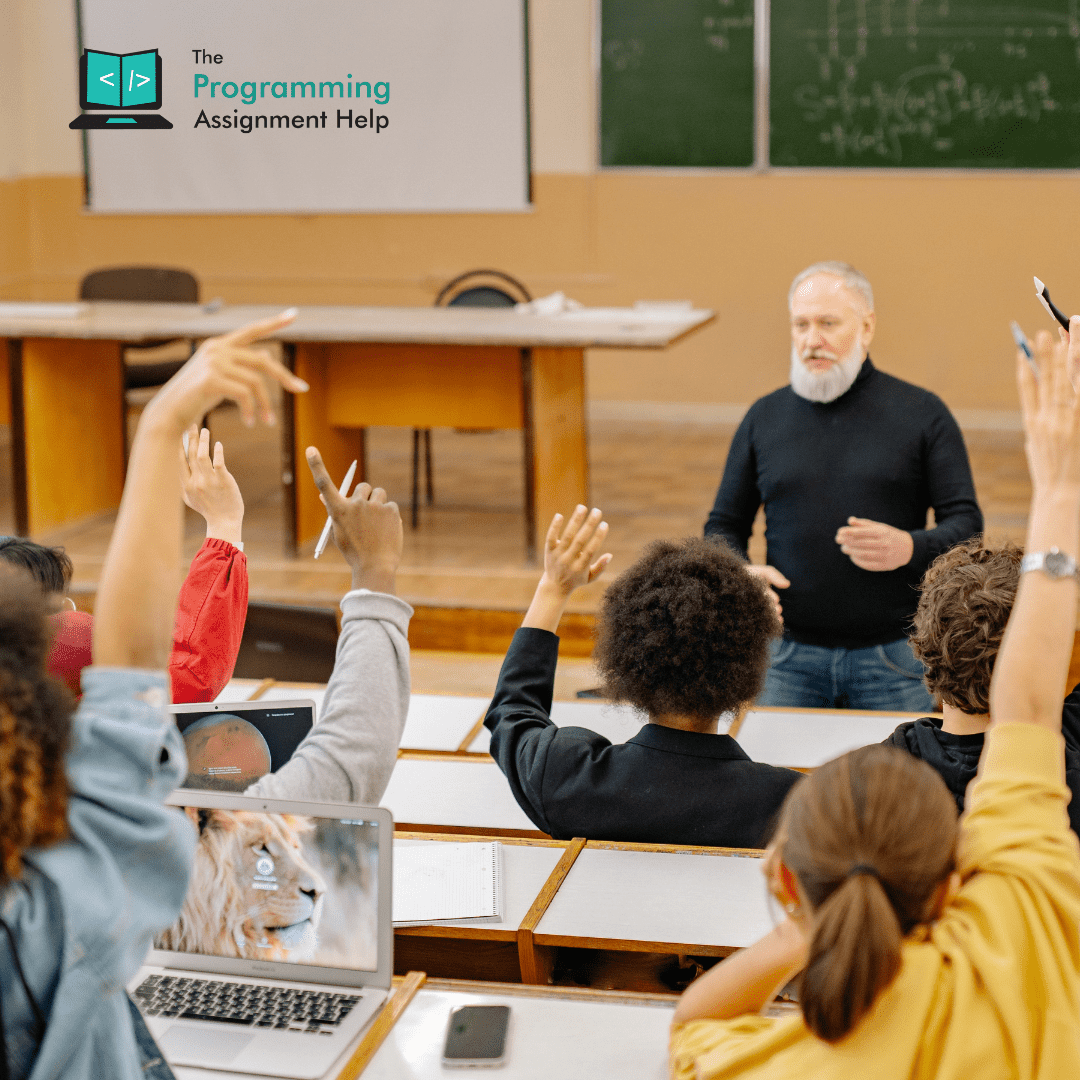
- 4th Nov 2022
- 04:01 am
Python Assignment Solution on Hash Table
from linearProbingHash import LinearProbingHash
from quadraticProbingHash import QuadraticProbingHash
from doubleHashingProbingHash import DoubleHashingProbingHash
class Driver:
def __init__(self):
self.tableSize = 191
self.doubleFactor = 181
self.fout = None
self.data = None
def testKeyValue(self, description, hashTable, key, value):
previousCollisions = hashTable.getCollisions()
hashTable.put(key, value)
retrievedValue = hashTable.get(key)
if retrievedValue == None:
retrievedValue = "null"
self.fout.write("{} : {} -> {}, collisions {}\n".format(key, value, retrievedValue, hashTable.getCollisions()-previousCollisions))
if (retrievedValue == "null") or (retrievedValue != value):
print("ERROR !!! value mismatch ")
def testInputKey(self, key, lph, qph, dhph):
value = key * 2
self.testKeyValue("Linear ", lph, key, value)
self.testKeyValue("Quadratic", qph, key, value)
self.testKeyValue("Double ", dhph, key, value)
self.fout.write("\n")
def testData(self, description):
self.fout.write("*** {} Start *** \n\n".format(description))
lph = LinearProbingHash(self.tableSize)
qph = QuadraticProbingHash(self.tableSize)
dhph = DoubleHashingProbingHash(self.tableSize, self.doubleFactor)
for key in self.data:
self.testInputKey(key, lph, qph, dhph)
self.fout.write("Linear {} collisions\n".format(lph.getCollisions()))
self.fout.write("Quadratic {} collisions\n".format(qph.getCollisions()))
self.fout.write("Double {} collisions\n".format(dhph.getCollisions()))
self.fout.write("\n*** {} End *** \n\n".format(description))
def readData(self, inputFile):
with open(inputFile, "r") as f:
lines = f.readlines()
self.data = []
for line in lines:
self.data += [int(i) for i in line.strip().split()]
def testFile(self, inputFilename, outputFilename):
print("Input file : {}, Output file : {}".format(inputFilename, outputFilename))
self.readData(inputFilename)
self.fout = open(outputFilename, "w")
self.testData("Random Order")
self.data.sort()
self.testData("Ascending Order")
self.data = self.data[::-1]
self.testData("Descending Order")
self.fout.close()
print("Done ... ")
def main(self):
self.testFile("in150.txt", "out150.txt")
self.testFile("in160.txt", "out160.txt")
self.testFile("in170.txt", "out170.txt")
if __name__ == "__main__":
d = Driver()
d.main()
Get the best Python Programming Homework Help online from our qualified programmers.