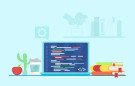
- 1st Nov 2023
- 14:53 pm
- Admin
Python's Object-Oriented Programming (OOP) paradigm stands as a robust approach for structuring and arranging code by modeling real-world entities as objects. This section delves into essential topics that lay the groundwork for comprehending OOP in Python.
- What is Object-Oriented Programming (OOP)?
"Object-oriented programming," a programming paradigm, revolves around the concept of "objects." In OOP, we model real-world entities as objects, each comprising data (referred to as attributes) and behaviors (known as methods).OOP encourages code modularity, reuse, and maintainability by dividing it into smaller, independent components.
- Why does Python employ OOP?
Python makes OOP one of its fundamental paradigms, empowering programmers to write clear, orderly, and simple-to-maintain code. You can represent complicated systems and interactions with OOP, which makes your code more understandable and manageable.
- Important OOP ideas: classes and objects
OOP's core components are "classes" and "objects." A "class" is an outline or model for making things that specifies their properties and operations. examples of classes that serve as "objects" are meant to represent particular examples of the modeled thing. The cornerstones of Python OOP, classes and objects serve as the foundation for more complex ideas within the paradigm.
This introduction lays the groundwork for learning Python's OOP, where you'll discover how to construct classes, create objects, and use encapsulation, inheritance, and polymorphism to create reliable, manageable software.
Creating Classes and Objects
Classes and objects are the basic building elements in the field of object-oriented programming (OOP). The creation of classes and objects in Python will be covered in detail in this part, giving you a solid basis for your OOP adventure.
- Python Class Definition:
A class acts as a blueprint for creating objects endowed with specific attributes and behaviors. In Python, you declare a class using the 'class' keyword, followed by the chosen class name. Within the class, you define attributes and methods to encapsulate the qualities and operations of the object. For example:
```
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return "Woof!"
```
- Creating Objects from Classes:
Once a class is defined, you can create objects or instances of that class. Objects are like tangible entities created from the class's blueprint. To create an object, you call the class like a function. For example:
```
my_dog = Dog("Buddy", "Golden Retriever")
```
- Understanding 'self' and Instance Variables:
In Python, the 'self' argument pertains to the class instance, enabling access to the instance's attributes and methods. Instance variables, like'self.name' and'self.breed' in the above illustration, contain information particular to each object derived from the class. They serve to represent the status and properties of the object.
The foundation of OOP in Python is knowing how to construct classes, generate objects, and interact with instance variables. You'll be able to model complicated systems, write reusable code, and develop software that replicates real-world entities and interactions as you delve further into these ideas.
Attributes and Methods
Python classes must have attributes and methods because they define an object's behavior and attributes. These essential components—methods, instance attributes, class attributes, and the idea of constructors and destructors—will all be covered in this section.
- Class and instance attributes:
Python classes include variables called attributes that hold data. The distinctive qualities of each object are represented by instance attributes. Conversely, all instances within a class share the same class attributes. To illustrate, in a class representing vehicles, the color of a specific car (an instance attribute) might differ from one car to another. However, the manufacturer (a class attribute) remains constant across all cars.
- Methods in Python Classes:
A class's specified functions known as methods provide objects the ability to carry out operations or complete calculations. These methods are able to access and modify properties and create class-specific actions. For example, the 'Person' class may contain a method called 'greet()' to introduce themselves.
- Constructors and Destructors:
Constructors, often denoted by '__init__()', serve as dedicated methods employed to set up an object's attributes when it is created. They enable you to establish the initial state of the object, preparing it for subsequent use. When an object is ready to be destroyed, destructors like '__del__()' are called, offering a way to carry out cleaning operations.
Effective class design and modeling depend on an understanding of attributes, methods, constructors, and destructors. These ideas serve as the foundation for generating objects that encompass both data and behavior, allowing you to write complex and reusable code using Python's OOP paradigm.
Inheritance and Polymorphism
In Python's Object-Oriented Programming (OOP) paradigm, the advanced concepts of inheritance and polymorphism empower developers to construct versatile and intricate class hierarchies. The introduction of these ideas emphasizes their significance for the structure and reuse of code.
- Grasping Inheritance:
Inheritance involves creating a fresh class by inheriting the attributes and features of an existing class. The original class, often termed the base class or superclass, serves as the blueprint for the newly formed class, also recognized as the subclass or derived class. This approach fosters code reusability and the construction of specialized classes without the need to start from scratch.
- Creating Subclasses and Base Classes:
You must create a subclass that derives properties and methods from a base class in order to establish an inheritance connection. The functionality of the base class can be increased by subclasses by adding new properties or methods or by overriding those that already exist. Building complicated systems and simulating real-world connections both require this hierarchical structure.
- Polymorphism and Method Overriding:
Different classes' capacity to respond to the same method call in a fashion that is appropriate for their particular type is known as polymorphism. Subclasses can provide their own implementation of methods inherited from the base class by using method overriding. Flexibility and dynamic behavior are made possible at runtime.
For example, Consider a hierarchy of shapes, where there exists a foundational class named "Shape," along with subclasses like "Circle" and "Rectangle." In this setup, the 'Shape' class acts as the origin for shared attributes and methods inherited by every subclass. However, each subclass retains the freedom to possess its unique characteristics, allowing for individuality within the hierarchy. The interaction with various shapes is uniform thanks to polymorphism, and each shape reacts correctly thanks to method overriding.
It is important to understand inheritance and polymorphism in Python's OOP paradigm because they facilitate code reuse, maintainability, and a more understandable representation of complicated connections.
Encapsulation and Abstraction
Developers may create reliable and maintainable code by using the core OOP ideas of encapsulation and abstraction. In this section, we delve into these concepts within the realm of Python classes, emphasizing their significance in shaping code structure and enhancing data security.
Encapsulation entails the consolidation of data (attributes) and the methods (functions) responsible for manipulating that data into a unified entity, which is the class itself. In Python, you achieve data encapsulation by defining class properties and methods as integral components of the class. Access modifiers (like private or protected attributes) can be used to limit direct access from outside the class in order to regulate access to certain properties. This encourages data integrity and captures the inner workings of the class.
- Abstraction and Hiding Implementation Details:
Abstraction is the presentation of an object's key characteristics while hiding its extraneous or complicated implementation details. This is accomplished with Python classes by hiding the internal intricacies and offering a clear and accessible interface for interacting with the object. Class users have the straightforward task of knowing how to employ the methods and attributes provided; they don't need to concern themselves with the underlying implementation details.
For example, consider the 'BankAccount' class, which might offer methods such as 'deposit()' and 'withdraw()' alongside properties like the account balance. Users can easily interact with these elements without needing to understand the intricate workings behind the scenes. Users of the class can interact with it through a simple and straightforward interface by abstracting away the inner workings of interest computations or data storage.
In Python's OOP paradigm, encapsulation and abstraction support the development of modular, safe, and user-friendly classes. You may create software that is more reliable and manageable by exposing only what is essential and concealing implementation specifics.
Working with OOP in Real-World Scenarios
To fully understand the capabilities of Object-Oriented Programming (OOP) in Python, it's crucial to delve into practical, real-world situations where OOP principles can be effectively employed. This section is dedicated to showcasing real-world applications that illustrate how custom classes can be harnessed to accomplish specific tasks.
- Useful Examples of OOP in Python:
Object-Oriented Programming (OOP) is more than just an abstract concept; it's a practical technique with a multitude of real-world applications. It allows you to construct objects and classes that mirror tangible entities, such as a "Car" class for automobiles, a "Person" class for individuals, or even a "BankAccount" class for managing financial transactions. OOP transforms abstract ideas into tangible, functional code structures. These unique classes include properties and operations pertinent to the particular domains they represent.
- Building and Using Custom Classes for Specific Tasks:
To handle specific duties and difficulties, custom classes can be created. To manage product data in a retail system, for instance, a unique 'Inventory' class can be built. Methods for adding, updating, and obtaining product information can be defined. By utilizing OOP, you may create a structured, orderly, and user-friendly solution that is simpler to maintain and modify as the project progresses.
Understanding OOP through real-world applications not only shows its usefulness but also empowers you to use its benefits in your own projects. Python's OOP features provide a potent approach to structure and manage your code, whether you are creating software, managing data, or simulating actual systems.
Advanced OOP Concepts
Once you've mastered the foundations of Python's Object-Oriented Programming (OOP), you may go on to more complex ideas that improve the expressivity and flexibility of your code. These advanced OOP ideas are covered in this section:
- Decorators for methods and classes:
Python's decorators are an effective feature that may be used with either methods or classes. By employing method decorators, you can modify a method's behavior without needing to make alterations directly in its code. Conversely, class decorators offer the means to enhance or alter the behavior of entire classes. These decorators provide a flexible and efficient way to tailor the functionality of methods and classes to suit specific requirements. For responsibilities like logging, access control, and memoization, decorators are frequently employed.
- Unique Techniques (Dunder Techniques) for Changing Class Behavior:
You can modify the behavior of your classes by using special methods, which are sometimes referred to as "dunder" methods (short for double underscore). When performing activities like object creation, string representation, and arithmetic, these techniques are used. You may modify how instances of your class behave in different scenarios by creating these methods in your classes.
Python enables multiple inheritance, allowing a class to derive from many parent classes. This is known as method resolution order (MRO). Conflicts in method naming may result, though. When a method is called on an instance, the Method Resolution Order (MRO) specifies the order in which base classes are looked up. Understanding MRO is essential to resolve ambiguity and control method resolution in complex inheritance hierarchies.
These more complex OOP ideas provide you with the ability to design class structures that are more dynamic and adaptable, which is very useful when working on complicated or large-scale projects. You may create highly customized and feature-rich classes that satisfy the particular requirements of your applications by making use of decorators, special methods, multiple inheritance, and MRO.
Best Practices and Design Patterns
The key to writing effective and maintainable Python Object-Oriented Programming (OOP) code is to follow best practices and comprehend design patterns. We will examine several essential facets of OOP development in this part.
- Writing Clear and Maintainable OOP Code:
Clear code is not only easier to read and comprehend, but also to maintain and extend. The Single Responsibility Principle (which states that a class should have a single cause to change) and good code encapsulation are among the OOP best practices that are encouraged. Coding documentation and readability are further improved by using docstrings and informative comments.
- Design Patterns in Python OOP:
Design patterns are tried-and-true answers to frequent problems in programming. Design patterns in OOP provide models for resolving common design issues. The Singleton pattern, Factory pattern, Observer pattern, and other patterns are examples of design patterns. Understanding the appropriate times and methods for applying these patterns can significantly expedite your development efforts, elevate the code's overall quality, and facilitate collaboration among developers.
By incorporating best practices and design patterns, you can ensure that your Python OOP code is not only functional but also well-organized and adaptable, making it easier to manage and scale as your project evolves. Your OOP projects will be more robust and long-lasting if you follow these guidelines for developing code that is less prone to errors, simpler to test, and more easily understandable.
Use Cases and Real-world Applications
Exploring the uses of Python's Object-Oriented Programming (OOP) ideas in diverse fields is crucial to understanding their true capabilities. We'll go into real-world use cases and situations where Python OOP is frequently used in this part.
- Software Development:
OOP is the cornerstone for creating modular, reusable, and manageable code in Software development. Class-based software components may be created using OOP, which promotes code structure, encapsulation, and concern separation. OOP concepts are frequently utilized in frameworks like Django for web programming and PyQt for graphical user interfaces. - Data Analysis and Modeling: To build data models and structures, data scientists and analysts use OOP. Classes can represent data entities, and methods can provide procedures for data analysis and modification. OOP principles are applied by libraries like Pandas, which are often used for data analysis.
- Game development:
OOP offers a natural approach to representing game components like characters, objects, and environments in the field of game production. OOP concepts are the foundation of game engines like Unity and Unreal Engine, which allow developers to make intricate and engaging games.
- Robotics and automation:
OOP is essential for programming automation and robotics systems. Classes can describe the actions of sensors, actuators, and robot behaviors, enabling modular and adaptable control systems.
- Applications in Finance and Science: OOP is crucial for creating intricate financial and scientific simulations and models. It makes it possible to create classes that contain mathematical models and algorithms.
These practical uses highlight Python OOP's versatility and efficiency in tackling challenging issues as well as how it makes it easier to create software and systems that accurately reflect the complexities of the real world.