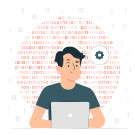
- 3rd Nov 2023
- 21:12 pm
- Admin
Python boasts versatile support for numeric data, covering integers, floating-point numbers, and complex numbers. This guide explores Python numbers, their types, methods of conversion, and essential numeric modules. Whether handling precise integers, versatile floating-point numbers, or intricate complex numbers, Python delivers the tools for efficient numerical data management. Additionally, Python offers modules for randomization and advanced mathematical operations, enhancing its utility in numeric computations.
What is Python Numbers?
In Python, numbers are essential components for performing mathematical operations, storing data, and making computations in various applications. Python provides built-in support for different types of numbers to accommodate the broad spectrum of numerical requirements.
Python numbers encompass a versatile array of numeric data types that facilitate computations with both simplicity and precision. These data types can represent integers, real numbers, and complex numbers. They are foundational to Python's ability to handle numerical tasks, from basic arithmetic to advanced mathematical calculations.
Python Number Types:
Python offers a diverse range of numeric types to accommodate a variety of numerical needs, granting programmers the precision to execute arithmetic and mathematical operations. The fundamental numeric types in Python encompass integers (int), floating-point numbers (float), and complex numbers.
- Integers (int): Integers portray whole numbers, devoid of decimal points, encompassing positive, negative, or zero values. Python supports integers of unlimited size, enabling unrestricted calculations, and making them indispensable for discrete tasks like counting, indexing, and various mathematical operations.
- Floating-Point Numbers (float): Floating-point numbers serve to represent real numbers involving decimal points. They excel in tasks that require fractional values or continuous data, offering high precision that is advantageous for scientific and engineering applications, where accuracy holds utmost significance.
- Complex Numbers: Complex numbers feature both real and imaginary components, expressed in the form a + bj, where 'a' represents the real part and 'b' the imaginary part. Complex numbers prove invaluable in resolving intricate mathematical challenges, notably in scientific and engineering domains.
# Integers (int)
x = 5
print(type(x))
# Output:
# Floating Point Numbers (float)
y = 3.14
print(type(y))
# Output:
# Complex Numbers
z = 2 + 3j
print(type(z))
# Output:
Type Conversion in Python:
In Python, type conversion is a pivotal concept that grants you the ability to transform the data type of a variable from one form to another. This flexibility proves immensely valuable when dealing with diverse data types and carrying out operations that demand compatible data types.
Python offers two primary approaches to type conversion:
- Using Arithmetic Operations:
Python permits the execution of arithmetic operations involving distinct data types. For example, the addition of an integer and a floating-point number triggers implicit type conversion, yielding a floating-point result. However, this method may not consistently produce the intended data type, hence, a need for caution.
x = 5
y = 2.0
z = x + y
print(z)
# Output: 7.0
- Using Built-in Functions:
Python boasts built-in functions explicitly designed for converting one data type to another. For instance, the int(), float(), and complex() functions are employed for converting data into integers, floating-point numbers, and complex numbers, respectively. Leveraging these functions provides precise control over type conversion, ensuring the desired data type is achieved.
a = 42.5
b = "100"
c = int(a)
d = float(b)
print(c)
# Output: 42
print(d)
# Output: 100.0
Python Random Module:
The Python Random Module emerges as an invaluable asset for generating random numbers and facilitating operations that demand randomness in Python. It encompasses an array of functions designed to produce random integers, floating-point numbers, and sequences of random choices.
Within the random module, you'll find functions like randint(), random(), and uniform() that cater to generating random numbers. These functions allow you to define specific ranges or boundaries, ensuring the retrieval of random values tailored to your exact specifications.
Additionally, the random module supports the generation of random choices from sequences or lists using the choice() function. This is particularly useful in scenarios like shuffling a deck of cards or picking a random item from a list.
Randomness is essential in various applications, including simulations, games, and cryptographic operations. The Python Random Module ensures that the random numbers generated are sufficiently unpredictable and can be used with confidence in your Python programs.
import random
# Generate a random integer between 1 and 10
rand_num = random.randint(1, 10)
print(rand_num)
# Generate a random floating-point number between 0 and 1
rand_float = random.random()
print(rand_float)
Python Mathematics:
Python boasts a formidable collection of mathematical functions and libraries that elevate it into a formidable instrument for numerical computation and data analysis. The Python Mathematics section navigates through a spectrum of mathematical operations and accessible libraries within the language.
Within Python, the math library reigns supreme by providing a comprehensive array of mathematical functions. Its offerings extend from fundamental arithmetic operations to the more intricate, encompassing calculations such as trigonometric, logarithmic, and exponential functions. This library is the preferred choice for a diverse array of mathematical tasks, particularly within scientific and engineering domains.
- Using the math library for exponentiation:
import math
result = math.pow(2, 3)
print(result)
# Output: 8.0
For more advanced mathematical work, the numpy library is widely used. It introduces support for arrays and matrices, enabling efficient numerical operations and complex mathematical manipulations. numpy is often used for data analysis, scientific computing, and machine learning tasks.
- Using the numpy library for array operations:
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
result = array1 + array2
print(result)
# Output: [5 7 9]
The sympy library focuses on symbolic mathematics, allowing you to perform algebraic operations symbolically, which is essential in mathematical research and symbolic computation.
The scipy library builds upon numpy and offers additional functionality for optimization, integration, interpolation, and more, making it a valuable resource for scientific and engineering applications.