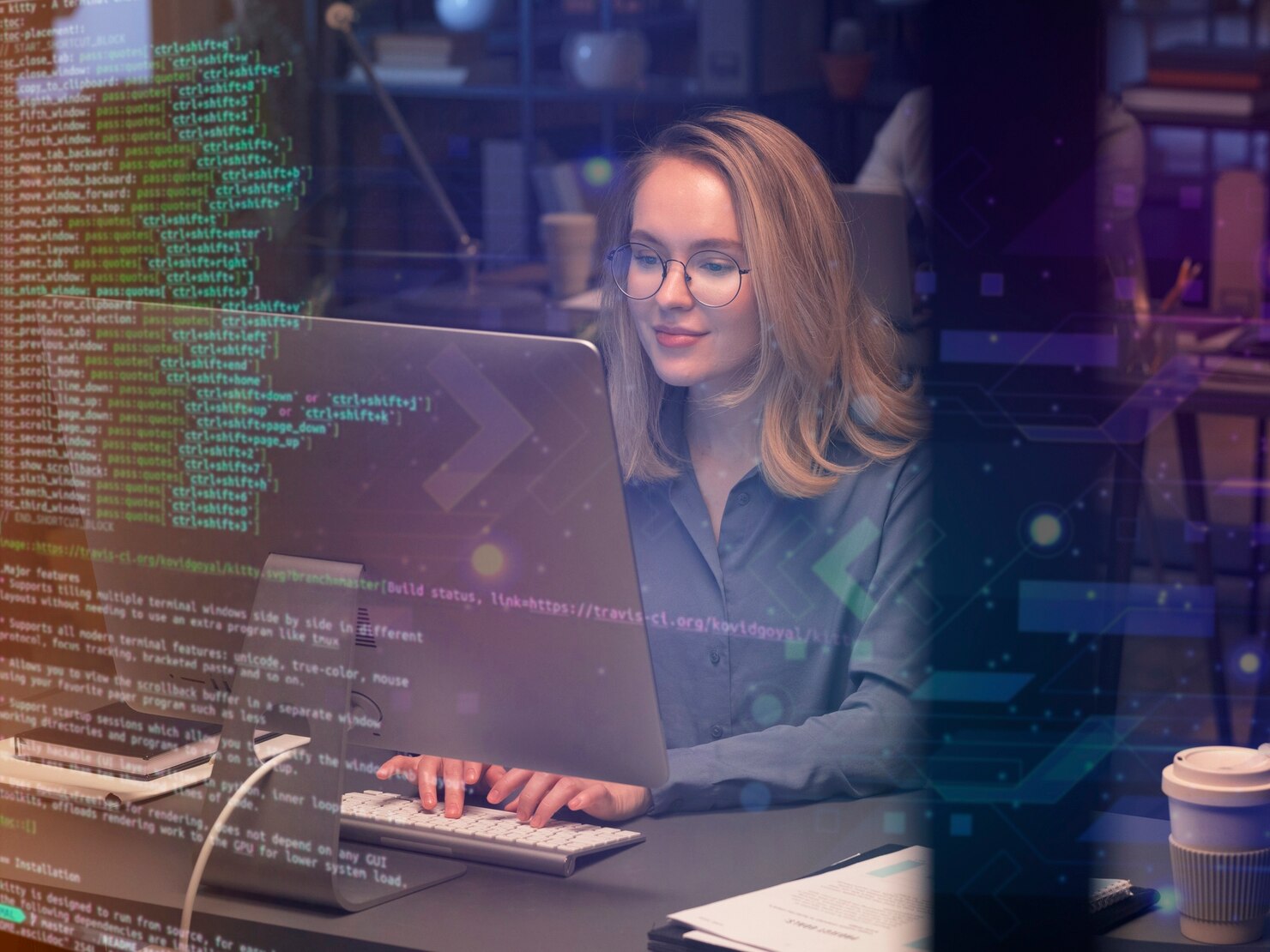
- 27th Dec 2023
- 14:48 pm
Converting a list into a string is a frequently encountered operation in Python, crucial for effectively handling data in various situations. Whether the goal is to present information, log data, or prepare it for transmission, the ability to seamlessly convert a list into a string is a fundamental skill.
Why Convert Python Lists to Strings?
In the realm of Python programming, understanding the rationale behind converting a list into a string is pivotal. This procedure offers notable benefits, enhancing the functionality of our code and contributing to a more organized and visually appealing appearance.
- Improved Readability: When we change a Python list into a string, it makes the data much easier to understand. This is especially helpful when dealing with lists that have different types of information or are a bit complicated.
- Clear Output: Converting lists to strings helps us create neater and more organized output. Whether we're showing information, keeping a log, or sending data somewhere, having it in string form often makes more sense.
- Better Performance: Using smart methods like .join() makes our code run faster, especially when we're working with a lot of data. This makes our programs use resources more efficiently.
- Easier Data Work: Turning lists into strings makes it simpler to handle data, fitting better with various tasks. This is really handy when we need our data in a specific format for our programs or applications.
Program to Convert a List to a String in Python
Converting a list into a string is a fundamental operation in Python, widely employed in programming tasks. Python offers various methods to achieve this, each with distinct advantages and suitable applications.
- Using for Loop:
While for loops can handle list-to-string concatenation, they might not be the most efficient choice for bigger lists. Consider exploring more streamlined approaches when working with larger datasets.
my_list = ['Hello', 'World', '!']
result_string = ''
for element in my_list:
result_string += element + ' '
- Using .join() Method:
The .join() method presents a more Pythonic and efficient approach for converting a list to a string. By employing a specified separator, this method effortlessly merges the elements of a list, resulting in code that is both neat and succinct.
my_list = ['Hello', 'World', '!']
result_string = ' '.join(my_list)
- Using List Comprehension:
List comprehension provides a concise and readable solution. It elegantly packs both the list iteration and string concatenation tasks into a single, expressive line of code, promoting both readability and efficiency.
my_list = ['Hello', 'World', '!']
result_string = ' '.join([element for element in my_list])
- Using map() Function:
The map() function serves to apply a designated function to all items in a given input list. List comprehension frequently demonstrates its versatility in seamlessly transforming individual list elements into strings and then gracefully merging them into a cohesive whole.
my_list = ['Hello', 'World', '!']
result_string = ' '.join(map(str, my_list))
- Using Enumerate Function:
The enumerate() function pairs each element of the list with its index, allowing for more complex string constructions. This is useful when the position of each element is significant.
my_list = ['Hello', 'World', '!']
result_string = ' '.join([f'{index}:{element}' for index, element in enumerate(my_list)])
- Using “in” Operator:
The "in" operator can be utilized to check if an element exists in the list before appending it to the result string. This ensures that the string contains distinct elements.
my_list = ['Hello', 'World', 'World', '!']
result_string = ''
for element in my_list:
if element not in result_string:
result_string += element + ' '
- Using functools.reduce Method:
The functools.reduce() method systematically applies a function to the elements of an iterable, progressively combining them. This method is particularly effective for handling more intricate concatenation operations.
from functools import reduce
my_list = ['Hello', 'World', '!']
result_string = reduce(lambda x, y: x + ' ' + y, my_list)
- Using str.format Method:
The str.format() method provides a versatile way to format and concatenate strings. Notably, list comprehension empowers you to weave variables or expressions directly into the final string, offering remarkable adaptability in crafting tailored output.
my_list = ['Hello', 'World', '!']
result_string = '{} {} {}'.format(*my_list)
- Using Recursion:
For individuals favoring a recursive approach, it's possible to design a function to manage the conversion. This method is well-suited for scenarios demanding a more dynamic or customized concatenation process.
def list_to_string(my_list):
if not my_list:
return ''
return str(my_list[0]) + ' ' + list_to_string(my_list[1:])
my_list = ['Hello', 'World', '!']
result_string = list_to_string(my_list)
About the Author - Jane Austin
Jane Austin is a 24-year-old programmer specializing in Java and Python. With a strong foundation in these programming languages, her experience includes working on diverse projects that demonstrate her adaptability and proficiency in creating robust and scalable software systems. Jane is passionate about leveraging technology to address complex challenges and is continuously expanding her knowledge to stay updated with the latest advancements in the field of programming and software development.