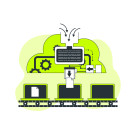
- 3rd Nov 2023
- 14:58 pm
- Admin
Flow control in Python is an essential concept that holds a significant role in programming. It deals with the sequence in which statements are executed within a program, offering you the ability to direct your code's course. Python, being a versatile programming language, equips you with multiple tools and mechanisms to establish and manage flow control.
What is Flow Control in Python?
Flow control in Python plays a pivotal role in programming, giving developers the tools to orchestrate the sequence of statements, make informed decisions, and navigate the flow of data. Python offers essential flow control structures that help sculpt the behavior of your code:
- Conditional Statements: In Python, programmers have access to conditional constructs such as "if," "elif," and "else" that serve as essential tools for making decisions within their code. For instance, the "if" statement enables the execution of a designated code block solely when a specified condition holds true. These constructs enhance code flexibility and control.
- Loops: Loops are the workhorses of repetitive tasks in Python. There are two primary types of loops – "for" and "while." "For" loops excel in iterating through sequences, such as lists or ranges, and running specific code for each element. In contrast, "while" loops persist as long as a designated condition remains true, making them suitable for situations where you need to repeat an action until a particular criterion is satisfied.
- Functions: Functions in Python act as a form of flow control by allowing you to encapsulate blocks of code that execute when the function is called. They promote code modularity, reusability, and structural organization. Through function definitions, you can structure your code logically, making it more manageable and comprehensible. Functions enhance program efficiency and maintainability.
- Exception Handling: Exception handling mechanisms like try, except, finally, and raise are employed to manage errors or exceptions that may occur during program execution. This is a critical aspect of flow control to ensure that the program handles errors gracefully.
Importance of Flow Control in Python
Flow control is integral to programming, and in Python, it serves several important purposes:
- Decision-Making: Conditional statements allow developers to make decisions in their code. This is vital for implementing different behaviors based on specific conditions, enhancing the adaptability and intelligence of your programs.
- Repetition: Loops provide the means to repeat actions or processes. This is invaluable for iterating over data structures, processing large volumes of data, and automating repetitive tasks.
- Modularity: Functions are a significant part of Python's flow control as they promote modularity. By breaking down code into smaller, reusable functions, developers can better organize and maintain their codebase.
- Error Handling: Exception handling ensures that a program doesn't abruptly terminate when it encounters errors. It enables the graceful handling of issues, improving the reliability and robustness of Python programs.
- Control and Efficiency: Flow control gives you control over the program's execution path, enabling you to design efficient algorithms and ensure that your code behaves as intended.
Types of Flow Control in Python
Flow control in Python is the heart of programming. It's what allows you to manage the execution of your code, making decisions based on conditions and repeating actions as needed. In Python, flow control comes in two primary categories: Conditional and Loop structures.
Conditional Flow Control
Conditional flow control helps you make decisions in your code, directing the program's behavior based on specified conditions. It's akin to constructing paths for your program to follow, depending on particular scenarios.
Python offers various conditional statements:
- if Statement:
The simplest form of conditional control, the if statement checks whether a certain condition is met and executes a block of code if it's true. For example:
# if Statement
x = 10
if x > 5:
print("x is greater than 5")
- if-else Statement:
The "if-else" statement presents a binary decision mechanism. It executes one action when a condition is true and an alternative action when false, enhancing code adaptability.
# if-else Statement
y = 3
if y > 5:
print("y is greater than 5")
else:
print("y is not greater than 5")
- if-elif-else Statement:
The if-elif-else statement is an extension of the if-else construct. This is employed when you need to assess multiple conditions in sequence. The if-elif-else statement permits you to examine a series of conditions one by one until one of them is true. Then it executes the corresponding code block:
# if-elif-else Statement
z = 5
if z > 5:
print("z is greater than 5")
elif z == 5:
print("z is equal to 5")
else:
print("z is less than 5")
Loop Flow Control
Loop flow control allows you to repeat a block of code multiple times, simplifying repetitive tasks in your program. Python offers two primary types of loops:
- For Loop:
The for loop is a versatile construct for iterating over a sequence (e.g., a list, tuple, or string). It repeats a block of code for each element in the sequence:
# For Loop
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
- While Loop:
A while loop repeats a block of code as long as a specified condition is true. This loop is particularly useful when you don't know beforehand how many times you need to execute the code:
# While Loop
count = 0
while count < 5:
print("Count is:", count)
count += 1
Breaks & Continues:
Python offers the "break" and "continue" statements as additional mechanisms to enhance the control flow of loops.
- Breaks:
The "break" statement is employed to prematurely terminate a loop.. When a specific condition is met within the loop, the "break" statement stops the loop and continues with the next section of your code. Here's an example:
for item in range(10):
if item == 5:
break # When item is 5, exit the loop prematurely
print(item)
In this example, the loop will stop when "item" equals 5, and the number 5 won't be printed. It will continue executing the code outside the loop.
- Continue:
The "continue" statement in Python functions as a method to exclude a certain portion of loop code based on specific conditions. For illustration:
for item in range(10):
if item % 2 == 0:
continue # Skip even numbers
print(item)
In this instance, when the condition "item % 2 == 0" is met, implying that "item" represents an even number, the "continue" statement activates. As a result, it skips the following code lines and proceeds to the next loop iteration. Consequently, the output consists of odd numbers exclusively.