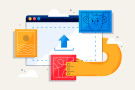
- 31st Oct 2023
- 23:49 pm
- Admin
Introduction to Python Files
Files are the main method used in programming to store and organize data. The computer language Python, which is flexible and popular, offers effective capabilities for working with files. But what are Python files precisely, and why are they so important?
Python files are incredibly flexible for managing and interacting with data. Files provide you with the capability to save, fetch, and modify a diverse array of data, spanning text, numbers, images, and a multitude of other data types. Python's versatility extends to a spectrum of file formats, encompassing text files, binary files, and specialized ones such as CSV and JSON. This diverse file handling capability enables efficient management of a wide array of data. These files act as a link between a program and outside data sources or storage, making them an essential component of any software application.
Importance of Working with Files in Programming:
Learning how to work with files holds significant importance for various reasons. Firstly, files empower programs to retain data between runs, ensuring that your data remains intact when the program ends. Secondly, files are essential for handling user input and generating output. Finally, files assume a critical role in practical situations, facilitating interactions with external databases, configuration files, and data storage.
Throughout this tutorial, we'll embark on a deeper exploration of Python files. We'll delve into the nuances of opening, reading, writing, and manipulating files. This journey will furnish you with the expertise needed to harness the complete potential of files for storing and retrieving data.
Opening and Closing Files
To harness the full potential of Python files, it's essential to grasp the proper techniques for opening and closing them. This section delves into the foundational aspects of file handling, encompassing topics such as initiating file access with the 'open()' function, understanding various opening modes, and emphasizing the significance of closing files correctly.
Python File Opening Instructions Making use of the 'open()' Function
In Python, you employ the 'open()' method to initiate file access. This method necessitates only the file name as its parameter and yields a file object, which becomes your gateway to reading from, writing to, or engaging with the file in various ways.
# Opening a file in read mode
file = open("example.txt", "r")
# Opening a file in write mode
file = open("new_file.txt", "w")
# Always close the file when done
file.close()
```
Remember to close the file using the `close()` method to release system resources and prevent potential issues.
Different Modes for Opening Files (Read, Write, Append, etc.):
The `open()` function allows you to specify different modes for opening files, depending on your intended file operations. Common modes include:
- `"r"`: Read mode (default), for reading data from the file.
- `"w"`: Write mode, for creating a new file or overwriting an existing one.
- `"a"`: Append mode, for adding data to an existing file.
- `"b"`: Binary mode, for working with binary data.
- `"x"`: Exclusive creation mode, to create a new file but raise an error if it already exists.
Securing File Closure to Prevent Resource Leaks:
Resource leaks resulting from improper file closure can significantly affect your software's performance. It's advisable to promptly close files once you've completed your tasks with them. A commonly embraced approach involves making use of the 'with' statement. This statement guarantees that the file is closed promptly, even if an exception occurs, as soon as you've completed your operations with it.
```
with open("example.txt", "r") as file:
data = file.read()
# The file is automatically closed outside the 'with' block.
Effective file handling in Python depends on knowing the right way to open and shut files and choosing the suitable mode. This understanding is a crucial building component for many real-world applications, whether you're reading, writing, or adding data to files.
Reading from Files
These use cases demonstrate the adaptability and importance of Python files in a variety of fields. Python files are essential resources for handling and organizing data properly, whether of your work in data, web development, software, system administration, or finance.
- File Reading Techniques: Python provides a number of file reading techniques, including:
- `read()`: This method reads the entire contents of the file as a single string. For example:
```
with open("example.txt", "r") as file:
content = file.read()
```
- `readline()`: `readline()` reads a single line from the file each time it's called, moving the file pointer to the next line. For instance:
```
with open("example.txt", "r") as file:
line1 = file.readline() # Reads the first line
line2 = file.readline() # Reads the second line
```
- `readlines()`: This method reads all lines from the file and returns them as a list of strings. Each string represents a line. For example:
```python
with open("example.txt", "r") as file:
lines = file.readlines()
```
Examples and Exercises:
To master the art of effective file reading, practice is key. Here are some hands-on exercises and examples to reinforce your understanding:
- Reading a Text File: Craft a program that reads the contents of a text file and displays them on the console.
- Line Counting: Develop a program that tallies and presents the number of lines in a given file.
- Data Extraction: Create a program that extracts and processes specific information from a file with data structured in a particular format, like CSV.
- Word Frequency Analysis: Design a program that scans a text file and computes the frequency of each word, unveiling the most commonly used terms. Students can enhance their proficiency in reading text and data from files by engaging in these exercises and experimenting with various reading techniques. The ability to extract and manipulate data from files is a fundamental skill applicable in data analysis, text processing, and numerous real-world scenarios.
- Writing to Files: Within the Python domain, your capabilities extend beyond reading data from files; you can also inscribe data into them and effect modifications. This section delves into the art of crafting and editing text and data files using Python. It introduces techniques for writing to files, including the "write()" and "writelines()" methods.
Methods for Writing to Files:
Python provides methods for writing data to files:
- `write()`: This method empowers you to inscribe a single string or text into a file. It's useful for both creating new files and overwriting the content of existing ones.
- `writelines()`: The "writelines()" method is employed for writing a list of strings into a file, such as a list of lines in a text file.
- Creating and Modifying Text and Data Files Using Python: Python provides the versatility to generate new files, append data to existing ones, or completely rewrite their content as required. It serves as a multifaceted tool for managing an array of file formats, spanning text, CSV, JSON, and more. For example:
```
with open("new_file.txt", "w") as file:
file.write("This is a new text file.")
```
This code creates a new text file and writes a line of text into it.
Understanding how to write to files is essential for storing data, generating reports, and interacting with various file formats in Python. Whether you're saving text or data, these methods empower you to manipulate and manage files efficiently.
Working with File Paths
Managing file paths is an essential Python skill for effectively working with files and directories. This chapter examines the significance of knowing where files are located and how to browse directories and manage file paths in Python.
Comprehending File Paths and File Locations:
A file or directory's position inside the file system is specified by its file path. They can start from the root directory or the current working directory and be either absolute or relative. For proper file access and discovery, understanding the differences is crucial.
How to Navigate Directories and Handle File Paths in Python:
Python comes with a potent library called 'os' that enables you to interact with file paths, whether you're creating them, verifying that files exist, or modifying directory structures. Assuring that your code runs consistently on various operating systems is the 'os.path' module, which offers methods for generating, joining, and altering file paths.
Working with files and directories in Python requires a solid understanding of file paths. It lets you to create adaptive, portable code that can handle various file locations and structures with ease, making your file-handling activities more reliable and versatile.
File Handling Best Practices
Writing code that functions and following best practices for reliable and maintainable solutions are both essential for effective file handling in Python. This section explores the best programming methods for interacting with files and offers advice on how to handle errors like file not found and permission problems.
Good Programming Practices for Working with Files:
- Utilize Context Managers ('with' Statement): When working with files, utilize context managers (the 'with' statement) at all times. When you're finished working with a file, it makes sure that it is correctly closed, reducing resource leaks and enhancing code readability.
- Select Meaningful Variable Names: Meaningful variable names help maintainability and code readability. Use 'file_path' as opposed to 'f', for instance, to denote the file path.
- Do not use magic numbers or strings: Do not explicitly hardcode file paths, file mode, or other file-related information into your code. Use configuration files or constants to store such settings as an alternative.
Advice on Handling Errors:
Handling File Not Found Errors: Be ready for a file not found error when opening a file. To gracefully handle errors, use 'try' and 'except' blocks. For example:
```
try:
with open("file.txt", "r") as file:
content = file.read()
except FileNotFoundError:
print("The file does not exist.")
```
Handling Permission Issues: Take note of file permission problems, particularly when writing to files. Your software can run into a permission problem if a file is read-only or situated in a directory with limited access. When errors are handled correctly, the user can get instructive notifications or the error can be logged for troubleshooting.
The reliability and maintainability of your file-handling code are improved by these recommended practices and error-handling pointers. By adhering to these recommendations, you not only create code that functions, but code that is easier to comprehend and less likely to have problems or defects when working with files.
Working with CSV Files
A popular format for storing and transferring tabular data is Comma-Separated Values (CSV) files. We'll look at CSV files in this part, which are made up of rows and columns with values separated by commas. For data modification and data exchange across multiple software systems, an understanding of CSV files is crucial.
The 'csv' module in Python makes working with CSV files simpler. This module offers tools for effectively reading data from CSV files, writing data to them, and manipulating CSV data. Python's 'csv' package is an essential tool for data processing and analysis since it makes it simple to examine datasets, produce reports, or move data across applications.
Working with JSON Files
JSON (JavaScript Object Notation) is a user-friendly and adaptable data interchange format that simplifies data organization for humans. It finds widespread application in storing structured data, facilitating communication between servers and clients, and configuring settings. In this section, we'll introduce the 'json' module in Python, which becomes instrumental in handling JSON files.
The Python 'json' module streamlines the management of JSON data by providing methods to process it and encode Python data structures into JSON format. Proficiency in reading and writing JSON files is essential for effective handling of structured data, configuration setups, and data exchange with external systems. Python's `json` module ensures a seamless and standardized approach to interacting with JSON files, enhancing its significance as a valuable asset in data-driven applications.
Hands-On Exercises
To reinforce the concepts learned in this tutorial and enhance their practical skills, students will benefit from engaging in hands-on exercises that focus on working with files. These interactive coding exercises are designed to provide real-world scenarios and challenges related to file handling in Python. Here are a few exercises to get students started:
- File Copy Utility: Create a program that replicates the contents of one text file to another. This is known as a "file copy utility." Permit user input for the source and destination file paths.
- CSV Data Analysis: Create a program that take data from a CSV file and do simple analyses like averaging, adding, or determining the highest and lowest values of a certain column.
- JSON Data Transformation: Create a program that receives information from a JSON file, modifies it, and then writes the updated information to a fresh JSON file. The 'json' module of Python is highlighted in this experiment.
- File Search and Reporting: Create a tool that searches a directory of text files for a certain term or phrase. Create a report once the keyword has been located that lists the file names and line numbers where it may be found.
- Log File Analysis: Examine log files generated by a web server or application to extract and compile crucial data, like the quantity of error messages, the number of successful requests, and more.
Students get the chance to put their understanding of file management to use in real-world scenarios thanks to these practical tasks. Students can improve their comprehension and acquire assurance in their capacity to manage files in Python properly by working through these difficulties.
Use Cases and Applications
Python files are a flexible tool for data storage, modification, and interaction since they are essential in many applications and sectors. The following list contains the top five use cases and applications for Python files:
- Data Science and Analysis: Python files are used to read, write, and process data for activities including data cleansing, Data Analysis, and visualization in the discipline of Data Science. The ability of Python to handle files is essential for dealing with datasets in CSV, Excel, and JSON formats.
- Web Development: Web developers utilize Python files as a back-end database for web applications, to manage configuration files, and to store user data. This covers processing form input, managing user sessions, and handling HTML templates.
- Software Development: In software development, files are used to manage program settings, create configuration files, and store log information. This enables software developers to alter and improve apps.
- System Administration and Automation: For operations like log file analysis, automatic backups, and configuration management, system administrators use Python files. The ability of Python to read and manipulate files is crucial for automating system-related tasks.
- Financial Analysis and Reporting: Python files are used by the financial sector to manage and process financial data, produce reports, and do data analysis. Python is used to communicate with file types including financial data files and Excel spreadsheets.
These use cases demonstrate the adaptability and importance of Python files in a variety of fields. Python files are essential resources for handling and organizing data properly, whether of your work in data, web development, software, system administration, or finance.