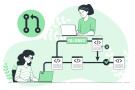
- 2nd Nov 2023
- 23:58 pm
- Admin
TheProgrammingAssignmentHelp stands out as a reliable source for top-notch assistance with Python data types. Our expert team provides comprehensive guidance on Python's diverse data types, including integers, floats, strings, lists, tuples, sets, dictionaries, and more. We cover topics like data type conversion, data type-specific methods, and the nuances of working with each type. Whether it's data validation, manipulation, or understanding the differences between mutable and immutable types, their support ensures you have a solid grasp of Python's data structures. With their assistance, you can make informed choices on the appropriate data type for your programming tasks, enhancing code efficiency and reliability.
Python Data Types
Python is a dynamically typed language. It means variable types are automatically set at runtime based on value assignment. It has a comprehensive set of built-in data types that are used to hold binary data.
There are mainly four data types in Python:
- Numeric: Numeric data types have integers (int) for whole numbers and floating-point numbers (float) for decimals. Python supports complex numbers (complex) too. These data types are crucial for performing mathematical and numerical operations.
- Boolean: Boolean data types, represented as True and False, are essential for logical operations and control flow. They help in decision-making and conditional statements, allowing the program to execute different code blocks based on conditions.
- String: Strings (str) are used to store text data. They are versatile and enable text processing, manipulation, and formatting. Python provides extensive string methods for string handling.
- Sequence: Sequence data types encompass lists, tuples, and ranges. Lists are mutable, ordered collections of items, while tuples are immutable. Ranges represent a sequence of numbers, often used in loops.
Understanding and effectively using these data types is fundamental in Python programming for various tasks and applications.
Integers can be used for counting and sequence indexing. Floats represent decimal numbers and are useful for measurements and fractional values. Complex numbers contain real and imaginary parts and are written as x + yi.
For text processing, Python provides the str type to represent strings as sequences of Unicode characters. Strings are immutable and can be enclosed in single or double quotes.
Python has three main sequence-type data structures - lists, tuples, and range objects. Lists are ordered, mutable collections that can contain mixed data types like strings, ints, etc. Tuples are similar to lists but are immutable once created. Ranges represent integer sequences and are often used for iterating.
Data Types | Classes | Description |
Numeric | int, float, complex | holds numeric values |
String | str | holds sequence of characters |
Sequence | list, tuple, range | holds collection of items |
Mapping | dict | holds data in key-value pair form |
Boolean | bool | holds either True or False |
Set | set, frozeenset | hold collection of unique items |
Dictionaries are Python's built-in mapping data type. They contain unordered collections of key-value pairs and provide a fast lookup of values based on keys. Dictionaries are dynamic and support any hashable object as a key.
Sets and frozensets represent unordered collections of unique objects in Python. Sets are mutable while frozensets are immutable. Sets implement mathematical set operations like unions, intersections, differences, etc.
Choosing the right data type leads to efficient memory usage and faster code execution. For example, tuples consume less space than lists and sets provide fast membership testing compared to sequential searches in lists.
Python makes type conversion easy through constructor functions like str(), int(), list(), set(), etc. This allows seamless conversion between compatible data types. Automated memory management in Python through garbage collection prevents leakage and other issues faced in languages like C, C++.
FAQs
Q: List data types in Python?
A: Python has different built-in data types including numeric types like:
- Integers, floats, complex numbers,
- String type for text,
- Boolean type for True/False,
- Sequence types like lists, tuples, ranges;
- Sets and dictionaries for unordered collections
Q: Explain what tuples are in Python?
A: Tuples are immutable ordered sequences of elements in Python. They are enclosed within parentheses and once created, their values cannot be modified. Tuples are useful for grouping related but different items together.
Q: What is the main difference between List, tuples and arrays in Python?
A: Lists are mutable Python objects that can contain mixed data types and variable lengths. Arrays are fixed-size mutable collections focused on numeric data and computations. Tuples are immutable ordered sequences that can store mixed data types.
Feature | List | Array | Tuple |
Ordered Collection | Yes | Yes | Yes |
Mutable | Yes | Yes | No |
Heterogeneous data types | Yes | No, single type | Yes |
Accessed by index | Yes | Yes | Yes |
Fixed size | No, dynamic | Yes | Yes |
Syntax | [] | np.array() | () |
Use cases | General purpose, storage | Numeric computing | Immutable storage |