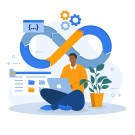
- 31st Oct 2023
- 21:29 pm
- Admin
The Programming Assignment Help excels in providing outstanding assistance with Python control flow. Their expert team offers comprehensive guidance on Python's control structures, including conditionals, loops, and functions. They cover if statements, for and while loops, and the intricacies of decision-making and repetitive operations. With their support, you can master the art of creating well-structured, efficient code that responds to various conditions and iterations.
Moreover, they provide in-depth insights into functions, helping you create reusable code blocks and modular programs. Their expertise includes parameter passing, return values, and understanding scope. They ensure you comprehend how to design and implement functions for better code organization and maintainability.
Their assistance empowers you to navigate control flow seamlessly, making your Python programs more versatile and powerful. Whether you're a beginner or an experienced developer, TheProgrammingAssignmentHelp's guidance ensures you have a strong foundation in Python's control flow mechanisms, enabling you to write code that meets your specific requirements efficiently.
Python Control Flow
Control flow in Python refers to the order in which statements, instructions or function calls are executed or evaluated. It helps in handling complex logic and decision-making. Let’s look into different types of Python control flow.
Type | Statement | Description |
Conditional | if | If a specified condition evaluates to True, a block of code will be executed. |
Conditional | if-else | Executes one block of code if a condition is true, and another block of code if the condition is false. |
Conditional | if-elif-else | Executes a block of code based on the value of a variable. |
Conditional | nested if-else | An if statement inside another if statement. |
Transfer | break | Exits a loop or function. |
Transfer | continue | Skips the remaining code in the current loop iteration and starts the next iteration. |
Transfer | pass | Does nothing. |
Iterative | for | A block of code is executed repeatedly for a specified number of iterations. |
Iterative | while | A block of code is executed repetitively as long as a given condition remains true. The execution stops when the condition evaluates to false. |
Comparison Operators
Comparison operators allows you to compare values and evaluate conditions. Eg., ==, !=, >, <, >=, and <=.
Boolean Operators
The boolean operators and, or, and not allow you to combine comparison expressions and evaluate more complex boolean logic.
Mixing Operators
You can mix comparison, boolean, and other operators together in complex expressions to create advanced logic.
if Statements
The if statement executes code only when a certain condition is met. You can also add an else block.
Flowchart of Python if statement
Flowchart
Flowchart of Python if-else statement
Flowchart
Python if-elif-else Ladder
Syntax:
if (condition):
statement
elif (condition):
statement
.
.
else:
statement
Flowchart of Python if-elif-else ladder
Example of Python nested if else statement
grade = 85
if grade >= 90:
letter_grade = 'A'
else:
if grade >= 80:
letter_grade = 'B'
else:
if grade >= 70:
letter_grade = 'C'
else:
if grade >= 60:
letter_grade = 'D'
else:
letter_grade = 'F'
print(f"Your letter grade is: {letter_grade}")
Output
Your letter grade is: B
Ternary Conditional Operator
The ternary operator provides a shortcut for if/else statements in a single line.
Syntax:
if condition: statement
# Python program to illustrate Ternary Conditional Operator
i = 100
if i < 150: print("i is less than 15")
Output:
i is less than 15
Switch-Case Statement
Some languages have a switch or case statement, but Python does not. However, you can replicate this behavior with if/elif/else.
Matching with the or Pattern
Python has an or pattern matching syntax that provides an alternative to switch case statements.
Matching by the length of an Iterable
A common pattern-matching technique is checking the length of an iterable object.
Matching Builtin Classes
You can match objects by their built-in Python class.
Guarding Match-Case Statements
You can use if statements inside match cases to add additional logic.
While Loop Statements
A while loop executes as long as a certain condition is True.
break Statements
The break statement exits the current loop early.
continue Statements
Continue skips the rest of the current loop iteration.
For loop
For loops iterate over a sequence like lists, tuples, strings etc.
The range() function
The range() can be used to generate the sequence for a for loop to iterate over.
For else statement
A for loop can have an optional else block that executes if the loop completes normally.
Ending a Program with sys.exit()
You can terminate a Python program early with sys.exit().
FAQs
- What is the difference between if, elif, and else in Python?
‘if’ is used to check a condition, elif (short for "else if") is used to check additional conditions if the previous if or elif condition was false, and else is used to provide a default action when no previous conditions are true.
- How can you handle exceptions and errors in control flow?
Exception handling in Python allows you to handle errors easily. You can use try, except, finally, and raise to manage exceptions in your code.