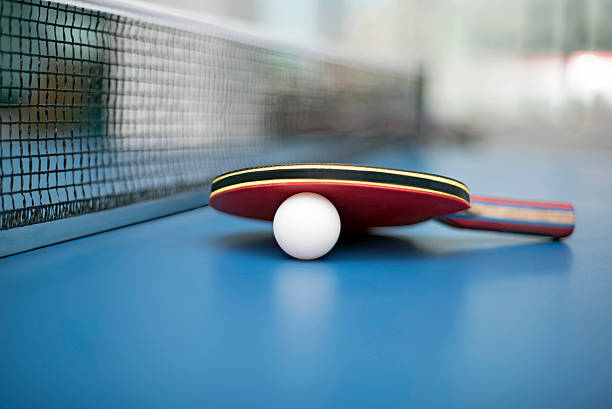
- 15th Jan 2024
- 18:13 pm
- Admin
What is a Pong Game?
Pong is one of the earliest arcade video games, originally released in 1972. It was created by Atari's founder Nolan Bushnell and is considered one of the first video games to achieve widespread popularity
The basic gameplay of Pong involves the following elements:
-
Paddles: There are two paddles, one for each player. The paddles can only move vertically along the sides of the screen.
-
Ball: A ball moves horizontally across the screen, bouncing off the top and bottom walls, as well as the paddles.
-
Scoring: Players score points when the opponent fails to return the ball. The game typically uses a simple scoring system where a point is awarded to the player who successfully makes the opponent miss.
-
Winning: The game continues until a predetermined score is reached. The player who reaches that score first is declared the winner.
Why Students Ask Us to Write Pong Game?
Pong game in Java programming is a very popular assignment that students get in their programming coursework. Students often come to us with queries to write simple games in Java Programming and we gladly help them with it. Here is a basic pong game written in Java by one of our Programmer
Java Code - Pong Game
This version is a two-player Pong game where each player controls a paddle using the keyboard. This is a sample code. Please thoroughly test the game to identify and fix any bugs or errors. Play test with different users to get feedback and make improvements.
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class PongGame extends JFrame implements ActionListener, KeyListener {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private static final int PADDLE_WIDTH = 20;
private static final int PADDLE_HEIGHT = 100;
private static final int BALL_SIZE = 20;
private int paddle1Y = HEIGHT / 2 - PADDLE_HEIGHT / 2;
private int paddle2Y = HEIGHT / 2 - PADDLE_HEIGHT / 2;
private int ballX = WIDTH / 2 - BALL_SIZE / 2;
private int ballY = HEIGHT / 2 - BALL_SIZE / 2;
private int ballSpeedX = 5;
private int ballSpeedY = 5;
public PongGame() {
setTitle("Pong Game");
setSize(WIDTH, HEIGHT);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setResizable(false);
Timer timer = new Timer(10, this);
timer.start();
addKeyListener(this);
setFocusable(true);
setFocusTraversalKeysEnabled(false);
}
private void update() {
// Move paddles and ball
movePaddles();
moveBall();
// Check for collisions
checkPaddleCollision();
checkWallCollision();
// Repaint the screen
repaint();
}
private void movePaddles() {
// Move paddle 1 (left paddle)
if (paddle1Y < 0) {
paddle1Y = 0;
} else if (paddle1Y > HEIGHT - PADDLE_HEIGHT) {
paddle1Y = HEIGHT - PADDLE_HEIGHT;
}
// Move paddle 2 (right paddle)
if (paddle2Y < 0) {
paddle2Y = 0;
} else if (paddle2Y > HEIGHT - PADDLE_HEIGHT) {
paddle2Y = HEIGHT - PADDLE_HEIGHT;
}
}
private void moveBall() {
ballX += ballSpeedX;
ballY += ballSpeedY;
}
private void checkPaddleCollision() {
// Check collision with paddles
if (ballX <= PADDLE_WIDTH && (ballY >= paddle1Y && ballY <= paddle1Y + PADDLE_HEIGHT)) {
ballSpeedX = -ballSpeedX;
}
if (ballX >= WIDTH - PADDLE_WIDTH - BALL_SIZE && (ballY >= paddle2Y && ballY <= paddle2Y + PADDLE_HEIGHT)) {
ballSpeedX = -ballSpeedX;
}
}
private void checkWallCollision() {
// Check collision with top and bottom walls
if (ballY <= 0 || ballY >= HEIGHT - BALL_SIZE) {
ballSpeedY = -ballSpeedY;
}
// Check if the ball passed the paddles (scored)
if (ballX <= 0 || ballX >= WIDTH - BALL_SIZE) {
ballX = WIDTH / 2 - BALL_SIZE / 2;
ballY = HEIGHT / 2 - BALL_SIZE / 2;
ballSpeedX = -ballSpeedX; // Change direction when scored
}
}
@Override
public void paint(Graphics g) {
super.paint(g);
// Draw paddles
g.fillRect(0, paddle1Y, PADDLE_WIDTH, PADDLE_HEIGHT);
g.fillRect(WIDTH - PADDLE_WIDTH, paddle2Y, PADDLE_WIDTH, PADDLE_HEIGHT);
// Draw ball
g.fillOval(ballX, ballY, BALL_SIZE, BALL_SIZE);
}
@Override
public void actionPerformed(ActionEvent e) {
update();
}
@Override
public void keyPressed(KeyEvent e) {
// Move paddle 1 (left paddle)
if (e.getKeyCode() == KeyEvent.VK_W) {
paddle1Y -= 10;
} else if (e.getKeyCode() == KeyEvent.VK_S) {
paddle1Y += 10;
}
// Move paddle 2 (right paddle)
if (e.getKeyCode() == KeyEvent.VK_UP) {
paddle2Y -= 10;
} else if (e.getKeyCode() == KeyEvent.VK_DOWN) {
paddle2Y += 10;
}
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyReleased(KeyEvent e) {
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
new PongGame().setVisible(true);
});
}
}
Blog Author - Janhavi Gupta
Janhavi Gupta is a highly skilled Java programmer with a passion for developing robust and efficient software solutions. With a strong foundation in Java programming, she excels in designing and implementing scalable applications. Janhavi is adept at solving complex coding challenges and has a keen eye for detail. Her expertise extends to various Java frameworks, ensuring the delivery of high-quality, performance-optimized software.