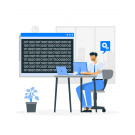
- 16th Nov 2023
- 00:44 am
Polymorphism in Java embodies the idea that a single function or operator can exhibit different behaviors based on the specific object it interacts with. This capability allows the same method to display diverse behaviors depending on the type of object, enhancing code flexibility and promoting reusability. It takes place both during compile-time and runtime, presenting varied methods of resolving methods based on the context. This feature helps ensure dynamic and versatile code execution.
What is Polymorphism in Java?
Polymorphism in Java encompasses the capability of a singular entity, such as a method, operator, or object, to exhibit diverse forms. This characteristic enhances code flexibility by allowing objects from various classes to be uniformly managed via a common interface. Fundamentally, it permits a function or object to perform different actions based on the context.
In Java, polymorphism is typically classified into two primary types: compile-time polymorphism and runtime polymorphism. Compile-time polymorphism involves method overloading, which can be further categorized into function overloading, operator overloading, and templates. Runtime polymorphism is largely enabled through the use of virtual functions. This concept permits the determination of the precise method to take place dynamically at runtime, relying on the specific type of the object being utilized. This capacity to enable methods or functions to display different behaviors makes Java code more versatile and adaptable to various scenarios.
Types of Java Polymorphism
Polymorphism in Java is evident in two fundamental types: compile-time polymorphism and runtime polymorphism.
Compile-time Polymorphism:
- Method Overloading: In Java, method overloading enables the creation of multiple methods within a single class that share the same name but have different parameters. The compiler distinguishes between these methods by considering the number and types of arguments passed.
- Subtypes of Compile-time Polymorphism:
- Function Overloading: Function overloading allows Java classes to include multiple methods with identical names but different parameters. This feature offers increased flexibility in method utilization within the code.
- Operator Overloading: Java doesn't inherently support operator overloading like other languages such as C++. Operators are predefined for specific types in Java.
- Template: Java doesn’t have explicit support for templates like C++ but achieves a similar concept using generics.
Runtime Polymorphism:
- Virtual Functions: Runtime polymorphism, often referred to as method overriding, is attained through inheritance in Java. It allows methods in a child class to replace methods in the parent class, providing distinct implementations. The determination of which method to invoke occurs at runtime and depends on the type of the object being referenced.
Polymorphic Variables
Polymorphic variables in Java are integral to implementing polymorphism. This idea concerns references that can point to different types of objects during runtime. It enables a base class reference to handle objects derived from it, improving the flexibility of managing various object types using the same reference.
For example, consider a fundamental class named "Vehicle" with subclasses like "Car" and "Bike." A polymorphic variable of the "Vehicle" class can be used to reference objects of these derived classes. This enables the invocation of methods on these objects without the compiler requiring prior knowledge of the specific object's type. At runtime, the decision regarding which method to execute depends on the particular class of the object being referenced.
Polymorphic variables significantly contribute to code flexibility by allowing a unified interface to access derived class objects through their base class reference, promoting concise, versatile, and adaptable coding structures in Java.
Example of Polymorphism in Java
- Example 1:
Method Overloading (Compile-time Polymorphism)
class Arithmetic {
int sum(int a, int b) {
return a + b;
}
double sum(double a, double b) {
return a + b;
}
int sum(int a, int b, int c) {
return a + b + c;
}
}
- Example 2:
Runtime Polymorphism (Method Overriding)
class Vehicle {
void accelerate() {
System.out.println("Vehicle is accelerating");
}
}
class Car extends Vehicle {
void accelerate() {
System.out.println("Car is accelerating");
}
}
class Bike extends Vehicle {
void accelerate() {
System.out.println("Bike is accelerating");
}
}
class PolymorphismExample {
public static void main(String[] args) {
Vehicle vehicle = new Car();
vehicle.accelerate(); // Outputs "Car is accelerating"
vehicle = new Bike();
vehicle.accelerate(); // Outputs "Bike is accelerating"
}
}
These illustrations showcase method overloading, where methods share the same name but differ in parameters or data types, and method overriding, which is accomplished by replacing a method from the parent class in a subclass. This display emphasizes the concept of polymorphism in Java.
Advantages of Polymorphism in Java
Polymorphism in Java provides numerous benefits in software creation. Here are a few of the advantages that come with using polymorphism in Java:
- Enhanced Code Reusability: Methods can be shared across different classes, reducing redundancy and simplifying code maintenance.
- Improved Readability: Polymorphism significantly aids in creating cleaner and more readable code, which in turn facilitates easier understanding and maintenance of the codebase.
- Interface Flexibility: It allows a single interface to invoke methods from various classes, offering codebase flexibility and extensibility.
- Dynamic Method Dispatch: Polymorphism enables dynamic method dispatch, determining the runtime behavior of an object based on the actual class instance, rather than the reference type.
- Flexible Design: Provides a foundation for designing more flexible, efficient, and maintainable Java applications.
- Efficient Debugging: It simplifies debugging and error tracking since you can focus on the specific behavior of objects rather than the type of each object.
Disadvantages of Polymorphism in Java
Despite its advantages, polymorphism in Java introduces certain drawbacks. Its extensive use can sometimes complicate code comprehension due to method resolution, resulting in a performance overhead.
- Complexity in Code Understanding: Extensive application of polymorphism might complicate understanding the code, especially within intricate inheritance structures.
- Performance Overhead: Dynamic method resolution may result in a minor performance overhead when compared to static or compile-time binding.
- Potential Confusion: Misuse of polymorphism can result in unexpected behaviors, especially when methods are overridden incorrectly.
- Learning Curve: For newcomers, understanding the subtleties and correct usage of polymorphism may pose a learning curve.
- Debugging Challenges: Debugging polymorphic code, particularly when encountering unexpected behavior, might be intricate, requiring a thorough understanding of class hierarchies and their interactions.