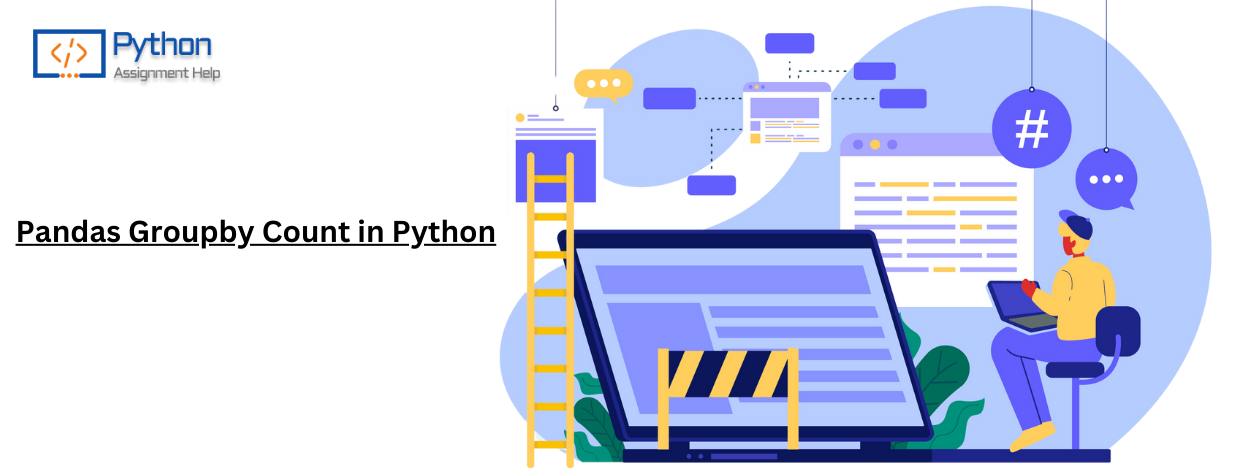
- 13th Feb 2024
- 16:04 pm
- Admin
Data exploration is all about understanding patterns and trends. With Pandas, a popular Python library, you can easily group your data by specific features and unlock valuable insights.
This journey focuses on group by-count, a powerful duo in pandas. groupby organizes your data, and count() tells you how many times things appear within each group. Imagine grouping customer purchases by product category – count() instantly reveals the most popular choices.
Whether you're analyzing website traffic, survey responses, or stock prices, group by-count becomes your secret weapon for uncovering hidden patterns. Get ready to count your way to data mastery!
Basic groupby.count()
Pandas' groupby.count() unlocks efficient group-wise counting, empowering you to analyze data at various granularities. Let's explore its usage through examples.
- Grouping by a Single Column:
df = pd.DataFrame({'product': ['apple', 'banana', 'apple', 'orange', 'banana'],
'price': [1.2, 0.8, 1.5, 2.0, 1.0]})
product_counts = df.groupby('product')['product'].count()
print(product_counts)
This code groups df by the product column and counts occurrences within each group. The output, a Series, displays product names as indices and their respective counts.
Similarly, group by a numerical column:
age_counts = df.groupby('price')['price'].count()
print(age_counts)
This output presents price points as indices and their corresponding counts
Output Format:
The output format (Series or DataFrame) depends on the grouping level. A single column grouping results in a Series, while multi-column grouping creates a DataFrame with hierarchical indexing.
- Excluding Missing Values:
Use dropna() before applying count() to exclude missing values:
product_counts_clean = df.groupby('product')['product'].count().dropna()
print(product_counts_clean)
This ensures missing values do not inflate counts.
- Grouping by Multiple Columns:
For deeper analysis, group by multiple columns:
product_price_counts = df.groupby(['product', 'price'])['product'].count()
print(product_price_counts)
This creates a MultiIndex DataFrame, accessible with appropriate indexing techniques. Access specific groups:
orange_counts = product_price_counts.loc['orange']
print(orange_counts)
- Clear Variable Naming and Labels:
Utilize meaningful variable names (e.g., product_counts instead of x) and informative axis labels to enhance code readability and maintainability.
Remember, this is just a starting point. Explore advanced topics like custom aggregations, weighted counts, and level-wise calculations to unlock the full potential of groupby.count().
Customizing Counts with groupby.count()
While basic counts provide valuable insights, groupby.count() in pandas truly shines when customized for specific needs. Let's delve into these customizations.
- Custom Aggregation Functions with apply():
Want to count unique values within groups? Create a function:
def count_unique(group):
return group['category'].unique().size
df.groupby('product')['category'].apply(count_unique)
This function counts unique categories within each product group. Similarly, calculate percentages within groups or apply custom filtering logic.
- Weighted Counts with the weights Parameter:
Imagine analyzing customer purchases. Assign weights based on transaction amounts:
df['weight'] = df['amount']
weighted_counts = df.groupby('product')['product'].count(weights='weight')
print(weighted_counts)
This prioritizes counts based on purchase values, revealing products generating higher revenue.
- Level-Wise Calculations with agg():
For multi-level groupings, apply different aggregations at different levels:
df = pd.DataFrame({'date': pd.to_datetime(['2023-01-01', '2023-01-02', '2023-01-03', '2023-02-01']),
'city': ['A', 'A', 'B', 'A'],
'value': [10, 20, 30, 40]})
grouped_data = df.groupby(['date', 'city']).agg(total_count=('value', 'count'),
avg_value=('value', 'mean'))
print(grouped_data)
This calculates both total and average values within each date-city group, providing a richer analysis.
These are just a few examples. Explore further customizations like using lambda functions for concise expressions or exploring alternative aggregation methods like size for non-numeric columns. Remember, clear variable names and comments are crucial for understanding your customized counts.
By mastering these techniques, you'll unlock the true power of groupby.count(), tailoring it to your specific data analysis needs and extracting deeper insights from your data.
Advanced Groupby Techniques
Pandas' groupby.count() offers immense flexibility, extending beyond basic counting to handle complex data structures and chained operations. Let's explore these advanced techniques:
- Hierarchical Grouping:
Imagine analyzing sales data with year, month, and day granularity. Hierarchical grouping shines here:
df = pd.DataFrame({'date': pd.to_datetime(['2023-01-01', '2023-01-02', '2023-02-01', '2023-02-03']),
'product': ['apple', 'banana', 'apple', 'orange'],
'sales': [10, 20, 30, 40]})
grouped_counts = df.groupby(['date', pd.Grouper(level='date', freq='M'), pd.Grouper(level='date', freq='D')])['product'].count()
print(grouped_counts)
This groups data by year, month, and day, allowing you to access and visualize trends at different levels (e.g., daily vs. monthly sales).
- Chaining Operations with pipe():
Streamline your code by chaining multiple grouping and aggregation operations using pipe():
counts_by_category = df.groupby('category').pipe(lambda x: x.groupby('product')['product'].count())
print(counts_by_category)
This achieves the same result as previous examples but in a more concise and readable manner.
Advanced Topics:
For even more complex scenarios, consider:
- Resampling: Group data at various time frequencies (e.g., hourly, weekly) for time-series analysis.
- Pivot Tables: Create cross-tabulations for insightful summaries across multiple dimensions.
- Custom Groupers: Define custom grouping criteria tailored to specific data structures.
These topics deserve further exploration, and several resources online provide in-depth tutorials and examples.
Remember:
Adapt these techniques to your specific data analysis needs.
Utilize clear variable names and comments for code maintainability.
Explore these advanced topics once you've mastered the basics.
By delving into these advanced techniques, you'll unlock the full potential of groupby.count(), tackling complex data structures and streamlining your analysis workflow.
Error Handling and Exceptions
- Data Type Mismatches: Ensure grouping columns have compatible data types. Attempting to group by a string column with a numeric count() function will raise a TypeError. Consider converting types beforehand or using appropriate aggregation functions.
- Key Errors: When grouping by non-existent columns, a KeyError occurs. Validate column names before applying groupby.count() to avoid this.
- Memory Errors: When dealing with large datasets, exceeding memory limits can lead to crashes. Utilizing try-except blocks allows graceful handling:
df.groupby('category')['item'].count()
except MemoryError:
print("Memory error occurred. Consider chunking or sampling.")
- Custom Error Messages: Craft informative error messages using except clauses to identify the specific issue (e.g., incorrect data type, missing column) and guide further actions.
Memory Optimization Techniques
- Chunking: Divide large DataFrames into smaller chunks and apply groupby.count() iteratively, reducing memory usage at once. Libraries like dask specialize in this for massive datasets.
- Sampling: Draw a representative sample from your data and apply groupby.count() on the sample. This provides an estimate while significantly reducing memory footprint.
- Data Type Conversion: Convert unnecessary columns to memory-efficient types like int8 for numeric data or category for categorical data.
- Aggregation Function Choice: Functions like size() (count non-null values) can be more memory-efficient than count() in specific scenarios.
Beyond these:
Utilize tools like memory_profiler to identify memory bottlenecks within your code and target optimization efforts effectively.
Consider distributed computing frameworks like Dask, allowing parallel processing on multiple cores for handling truly massive datasets.
Practical Applications and Use Cases of groupby.count()
pandas' groupby.count() function transcends academic exercises, becoming a crucial tool for extracting actionable insights from real-world business data. Let's delve into its diverse applications:
1. Customer Segmentation and Behavior Analysis:
- Group customer purchases by product category and quantify occurrences to reveal buying patterns.
- Analyze product co-purchasing relationships and identify seasonal trends for informed inventory management.
- Calculate unique customer counts per category to understand category reach and target acquisition efforts effectively.
2. Website Traffic Optimization:
- Group website visits by hour and count unique visitors to discover peak usage periods.
- Analyze user behavior across different hours and compare traffic trends between weekdays and weekends for targeted outreach.
- Leverage these insights to optimize website content, resource allocation, and marketing campaigns.
3. Survey Response Analysis and Market Research:
- Group survey demographic responses (age, gender, and location) is an excellent indicator for understanding response distributions by possible bias and estimates the percentage that each group holds.
- Analyze the sentiment trends across demographic groups for deep insights into user opinions and preferences, for product development and marketing strategy.
4. Stock Market Analysis and Risk Assessment:
- Group stock market data by date and calculate daily average prices to visualize trends, identify volatility periods, and compare performance across different stocks.
- By grouping by time frames (weekly, monthly) and calculating total counts or variances, gain insights into long-term trends and assess risk effectively.
Beyond the Fundamentals:
- Anomaly Detection: Identify unusual patterns in data by grouping and comparing counts across different dimensions. Deviations from expected values might indicate errors, fraudulent activity, or emerging trends, enabling proactive countermeasures.
- Feature Engineering: Create new features based on group-wise counts. For example, in customer data, calculating the number of purchases per customer within a specific time frame could predict future purchase behavior, informing targeted promotions.
Remember, the applications of groupby.count() are extensive and limited only by your analytical imagination and business objectives. By combining it with other powerful pandas functionalities, you can unlock a wealth of business intelligence from your data, driving informed decision-making and achieving strategic goals.