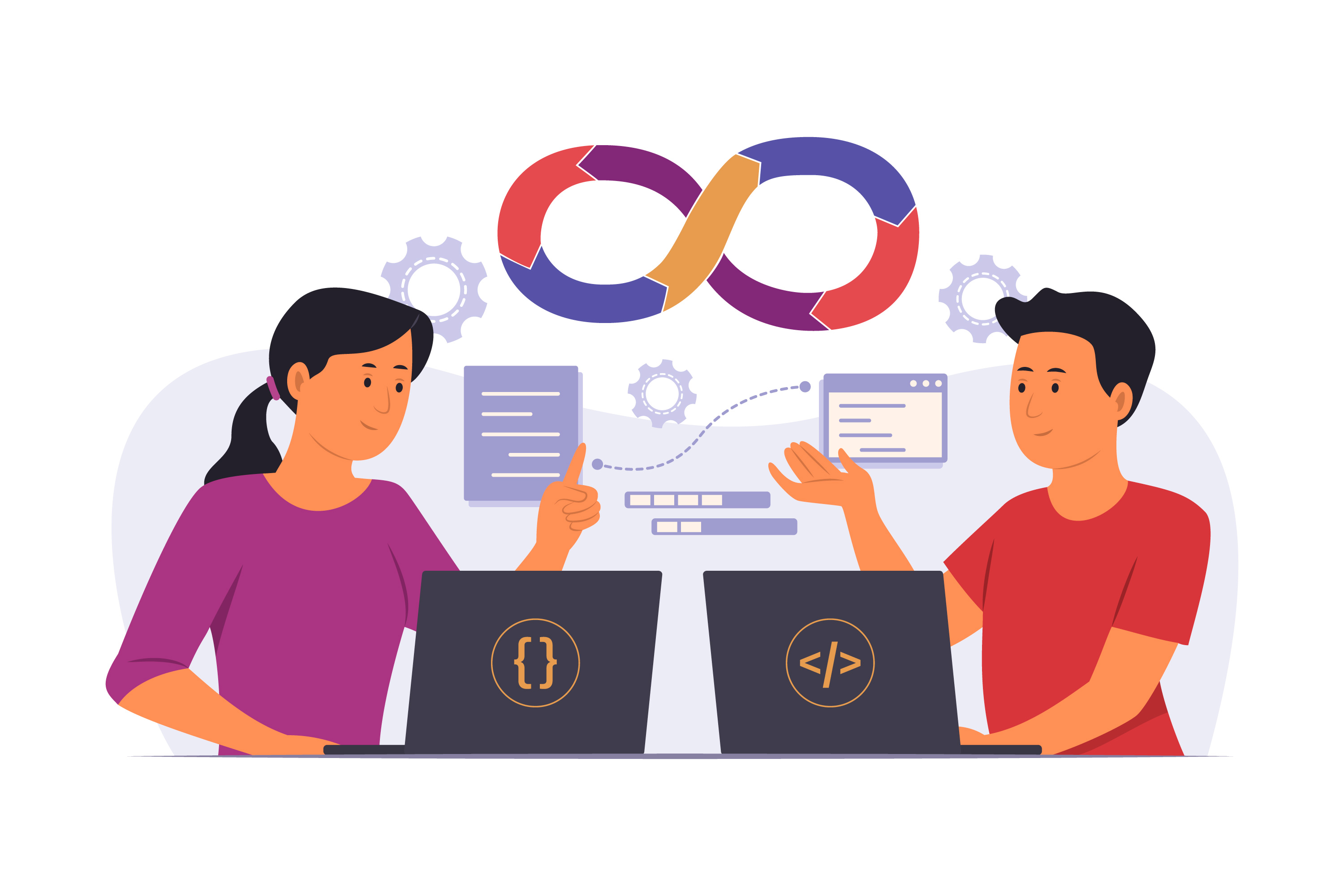
- 8th Feb 2024
- 23:23 pm
- Admin
Efficient data handling is vital in Python, and understanding how to represent and manage missing values is essential. While other languages grapple with uncertain "null" concepts, Python offers a clear solution: "None." We will guide you to confidently utilize "None" and effectively manage missing data in your Python projects.
Understand "None" as:
- Absence of Value: Consider it a reserved seat in your data table, explicitly indicating the lack of information.
- Missing Data Flag: It acts as a signal, highlighting where data is absent, preventing errors and ensuring code clarity.
- Distinct from Emptiness: Unlike other values like zero or an empty string, "None" specifically denotes the absence of any data.
Employ "None" for:
- Representing Missing Information: Whether in datasets, function arguments, or uninitialized variables, "None" accurately signals missing data points.
- Setting Default Values: When information might be unavailable, "None" serves as a safe placeholder, preventing unexpected issues.
- Terminating Loops and Recursion: Utilize "None" to gracefully conclude processes lacking required data.
Utilize "None" Checks:
- Employ if is None:: This efficient expression allows your code to react appropriately to missing data, ensuring smooth program execution.
- Avoid Confusing Comparisons: Remember, "None" is unique. Comparing it to other values like zero or empty strings can lead to unexpected results.
What is none in Python?
In Python, understanding "None" is critical for handling missing data effectively. Unlike other languages with ambiguous "null" concepts, "None" stands as a clear representation of the absence of a value. It's not just an empty string or zero; it's a distinct object signifying nothingness.
Understanding Its Nature:
- Keyword, not a value: "None" is a special keyword, not a regular data type. This means it behaves uniquely and shouldn't be confused with other values.
- Object, not emptiness: Though it denotes "no value," "None" is still an object, meaning it occupies memory and interacts with other objects through defined methods.
- Falsehood in disguise: When evaluated in conditional statements, "None" acts as "False," allowing you to handle its absence directly.
Distinguishing from Emptiness:
- Non-existent vs. empty: Remember, "None" implies no information exists, while empty sequences (strings, lists, dictionaries) have allocated space but no content.
- Comparisons matter: Don't assume "None" equals emptiness! "" != None and [] != None are both true. Use appropriate checks like is None for clarity.
Using "None" in Action:
- Assigning absence: my_variable = None explicitly indicates a data point hasn't been assigned or doesn't exist.
- Checking for emptiness: Conditional statements like if some_value is None: allow code to react specifically to missing data.
- Default values: When optional function arguments might be absent, setting them to "None" prevents errors and allows flexibility.
When to Use "None"
Understanding when to utilize "None" is essential for adept data management in Python. But let's explore the specific scenarios where this indicator of absence truly excels:
- Representing Missing Data: When datasets contain absent values, "None" acts as a clear flag, preventing ambiguity and ensuring data integrity.
- Default Value Representative: Optional function arguments often require safe placeholders. "None" gracefully fills this role, avoiding unexpected behavior when data might be unavailable.
- Uninitialized Variable Marker: While initializing variables, "None" signifies they haven't been assigned a value yet, maintaining code clarity and preventing errors.
- Optional Argument Indicator: When functions accept optional arguments, "None" serves as a signal that no value was provided, allowing for flexible function design.
- Loop and Recursion Termination Marker: In iterative processes, "None" can gracefully signal termination when specific conditions indicate no further processing is needed, acting as a termination marker.
Working with "None"
Understanding how to effectively check for and handle "None" is crucial.
Conditional Checks:
- if is None:: This concise expression directly verifies if a variable holds "None," facilitating efficient handling of missing values.
- if not None:: This inverts the check, allowing code to execute only if a value is present.
Checking Techniques:
- is None: The most efficient and recommended method for direct comparison against "None".
- isinstance(obj, type(None)): More versatile, but less efficient, this confirms if an object belongs to the same type as "None".
- Custom functions (is_null(obj)): Can offer flexibility, but might introduce overhead compared to simpler methods.