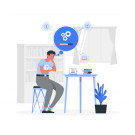
- 2nd Nov 2023
- 21:35 pm
- Admin
Python Memory Leaks can be a challenging issue for developers. In this detailed guide, we delve into the origins of memory leaks, explore the diagnostic tools at your disposal, and discuss effective strategies for addressing these memory management issues. Python developers will acquire a deep understanding of memory leaks, enabling them to leverage the expertise and methods required to create high-performing and robust applications.
What is a Python Memory Leak?
A Python Memory Leak is a situation where the Python interpreter retains references to objects in memory even after they are no longer needed, preventing memory reclamation. These lingering references gradually consume more memory, leading to increased memory usage, program slowdowns, or even crashes.
The primary factor contributing to memory leaks is the persistence of pointers to objects that should have been deallocated, hence impeding Python's automated garbage-collection mechanism. As a result, objects persist in memory unnecessarily, ultimately impairing the application's performance.
Memory leaks can occur due to various factors, including:
- Unintentional circular references: The presence of circular references between objects hinders the process of garbage collection, hence preventing their timely disposal.
- Long-lived references: Objects intended to be short-lived remain in memory, causing memory bloat.
Memory leaks can be especially problematic in long-running applications, such as web servers or daemons, where even small leaks can accumulate over time.
Identifying and resolving memory leaks is essential for maintaining the performance and reliability of Python applications. This may involve techniques like profiling memory usage, visualizing object relationships, and utilizing Python's garbage collection features. By understanding the root causes of memory leaks and implementing proper memory management practices, developers can mitigate this common issue in Python programming.
How Does Python Memory Leak Work?
Python Memory Leaks occur due to inadequate memory management within Python programs. They are a result of objects being created, used, and retaining references, even when those references are no longer required. These lingering references obstruct the Python garbage collector's efforts to identify and recover memory associated with unused objects.
The process of Python Memory Leaks can be broken down into the following steps:
- Object Creation: Python programs continually generate objects during their execution. These objects can encompass variables, data structures, or any dynamically allocated memory.
- Reference Retention: The maintenance of references to these objects is the crucial point. Python monitors the reference count of objects. As long as this count remains above zero, Python considers the object as still in use.
- Garbage Collection Hindrance: Memory leaks manifest when references to objects are unintentionally preserved, preventing the reference count from reaching zero. As a result, these objects linger in memory, despite being no longer required.
- Accumulation: Over time, as more objects are produced and references accumulate, the memory space occupied by unreleased objects accumulates as well. This accumulation leads to escalated memory consumption, causing the program to utilize more resources than necessary.
- Performance Impact: The gradual accumulation of unreleased objects in memory can significantly affect the performance of a Python program. It may lead to potential performance degradation, slowdowns, or even application crashes, particularly in long-running applications.
How to Diagnose Memory Leaks in Python
Diagnosing memory leaks in Python is a critical skill for developers to ensure their applications run efficiently and don't consume unnecessary resources. Several tools and techniques can be employed to identify and resolve memory leaks effectively.
Here's a comprehensive explanation of the diagnostic process for identifying memory leaks in Python:
- Profiling Tools: Python provides various profiling tools, such as cProfile and profile, which help developers measure the execution time of functions and methods. These tools can assist in identifying performance bottlenecks and potential memory leaks.
- Objgraph Module: The objgraph library is a powerful tool for visualizing object relationships. The graphical representation of object reference chains can assist developers in the identification of memory leaks, facilitating the identification of objects that are being maintained needlessly.
- Memory_profiler Module: The memory_profiler module is invaluable for tracking memory usage in Python programs. It can help pinpoint memory-hungry functions and lines of code by providing detailed memory consumption data, aiding in the detection of memory leaks.
- Tracemalloc Module: Python's tracemalloc module enables developers to trace memory allocations and deallocations. By comparing snapshots taken at different points in the code, it's possible to identify objects that are growing in memory over time.
- Garbage Collector Debugging: Python's garbage collector can be fine-tuned to provide debugging information. The GC module allows developers to enable debugging flags to track reference cycles and unreachable objects.
- Manual Inspection: Code inspection and manual tracing of references can also reveal memory leaks. Developers can analyze code to ensure that references are properly released when objects are no longer in use.
- Testing and Benchmarking: Comprehensive testing and benchmarking can help uncover memory leaks by identifying excessive memory consumption during specific operations or workflows.
How to Fix Memory Leaks in Python
Fixing memory leaks in Python is essential to ensure that your applications run efficiently and don't consume excessive resources. Once you've identified memory leaks using the diagnostic techniques mentioned earlier, it's time to take action.
The following are ways to address memory leaks in the Python programming language:
- Use Generators or Iterators: Instead of loading extensive data sets or lists into memory simultaneously, it is advisable to employ generators or iterators to handle data in smaller increments. This approach reduces the memory footprint, as data is retrieved and processed incrementally.
- Use Weak References: In cases where circular references are causing memory leaks, you can use weak references with the weakref module. Weak references allow objects to be garbage collected when there are no strong references to them.
- Optimize Data Structures: Review your data structures and ensure that they are efficiently designed. In some cases, switching to more memory-efficient data structures or implementing custom data structures can mitigate memory leaks.
- Profile and Refactor Code: Profiling tools like cProfile can help identify memory-hungry functions or code segments. Refactor these sections to minimize memory consumption by releasing references promptly.
- Use Context Managers: When working with resources like files or databases, employ context managers (with statements) to ensure that resources are properly released when they are no longer needed.
- Garbage Collection Debugging: Fine-tune the garbage collector settings with Python's GC module to optimize memory management. Enabling debugging flags can help identify and address reference cycles.
- Testing and Benchmarking: Rigorous testing and benchmarking can validate that memory leaks have been successfully addressed and that the application's memory consumption is within acceptable limits.
- Regular Maintenance: Continuously monitor and maintain your codebase to identify and resolve memory leaks as new features are added or the code evolves.