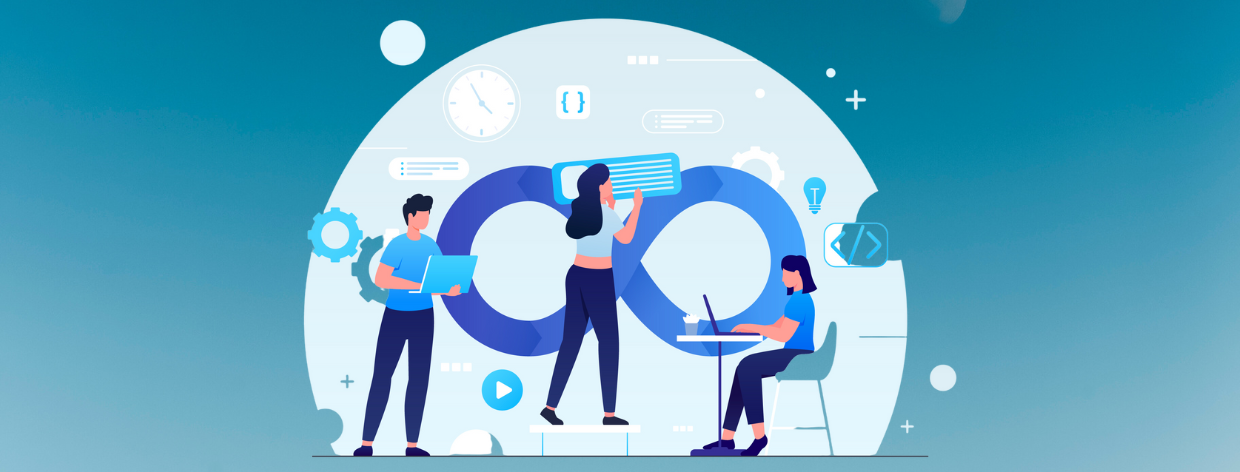
- 1st Mar 2024
- 15:26 pm
- Admin
In the world of programming, loops and conditionals emerge as essential tools for crafting versatile and interactive programs. These sets of fundamental constructs empower you to automate repetitive tasks and make intelligent decisions based on various conditions, ultimately breathing life into your code.
A loop is a control structure where a program carries out an action or a series of statements repeatedly a number of times, or until a certain given condition is fulfilled. For example: Consider the function as carrying the work of printing the values from 1 to 10 in order. Without loops, this would mean ten print statements, which is such a boring and error-prone approach. But using a loop, you can express this concisely in an automated way with the above.
On the other hand, conditionals work as smart devices for decision-making on your program. It allows one to check for conditions and then branch the execution of a set of programs according to that checked condition. It can be just a want to print a different message based on the age of some user. And such a comparison could be carried out only with a conditional statement, which will allow determining age and showing a corresponding message to the user.
Mastering such principles opens the door to be capable of writing code for programs that are not only effective, though but also interactive and adaptive to variation. With our Programming Assignment Help and Programming Homework Help service, we deal in detail with specific kinds of loops and conditionals so as to ensure that you have sufficient knowledge and skills necessary to go ahead and handle programming with confidence yet being in control.
Understanding Loops:
In programming, loops emerge as indispensable tools that define a series of instructions. Loops are necessary when it comes to iterating through collections. It will take you much deeper into loops and their use, helping you to fill any knowledge gaps that may be preventing you from looping effectively and putting together well-structured programs.
Unveiling the Different Loop Types:
- For loop:
The for loop excels at iterating a specific number of times, adhering to the following syntax:
for (initialization; condition; increment/decrement) {
// Code to be executed repeatedly
content_copy
- Initialization: This expression, executed once before the loop commences, is often used to declare and initialize a loop counter variable.
- Condition: This expression is evaluated before each iteration. The loop continues to execute as long as the condition remains true.
- Increment/decrement: This expression, executed after each iteration, typically updates the loop counter variable.
Example: Printing the squares of numbers from 1 to 5:
for (int i = 1; i <= 5; i++) {
std::cout << i * i << " ";
}
- While loop:
The while loop offers flexibility for scenarios with unknown iteration counts, executing a code block as long as a specific condition holds true. Its syntax is:
while (condition) {
// Code to be executed repeatedly
}
Condition: This expression is evaluated before each iteration. The loop continues as long as the condition remains true.
Example: Guessing a random number between 1 and 10:
int guess, randomNumber = rand() % 10 + 1; // Generate random number
std::cout << "Guess a number between 1 and 10: ";
while (std::cin >> guess && guess != randomNumber) {
if (guess < randomNumber) {
std::cout << "Too low! Guess again: ";
} else {
std::cout << "Too high! Guess again: ";
}
}
std::cout << "Congratulations! You guessed the number!" << std::endl;
- Do-while loop:
The do-while loop guarantees at least one execution of the code block, even if the initial condition is false. Its syntax is:
do {
// Code to be executed at least once
} while (condition);
Condition: This expression is evaluated after the code block is executed. The loop continues as long as the condition remains true.
Example: Checking if a user enters a valid input (a positive number):
int number;
do {
std::cout << "Enter a positive number: ";
std::cin >> number;
} while (number <= 0);
std::cout << "Thank you for entering a valid number." << std::endl;
Practical Applications of Loops:
- Iterating over arrays: Efficiently access and process elements of an array one by one, enabling tasks like calculating the sum of all elements or finding the maximum value.
- Calculating complex mathematical sums: Automate the calculation of series or sequences, such as calculating the factorial of a number or implementing various sorting algorithms.
- Generating intricate patterns: Create sequences or repeating elements based on predefined conditions, allowing you to build visually appealing patterns or generate random numbers within specific ranges.
- Simulating real-world scenarios: Model repetitive events or processes, such as simulating the movement of objects in a game or efficiently processing data streams.
Loops will teach you how to automate things and write smaller, neater code while helping you build systems that, through programming, interact with the rest of the world in interesting ways. The following section is an introduction to a concept, conditional, that would be helpful in writing even more potent scripts and be interactive and versatile in nature.
Understanding Conditionals:
Conditionals are used quite frequently in your day-to-day programming life, acting as intelligent gatekeepers that guide the flow of your program based on some criterion. This section explain you to the different kinds of conditional statements and guides on when to use them so that you can write programs that seem to take decisions and be ready for any kind of eventuality.
Unveiling the Conditional Arsenal:
- If statements:
The fundamental building block of conditional statements, the if statement evaluates a single condition and executes a code block if the condition is true. Its syntax is:
if (condition) {
// Code to be executed if the condition is true
}
Condition: This expression is evaluated, and the code block is executed only when the condition evaluates to true.
Example: Checking if a number is even:
int number;
std::cout << "Enter a number: ";
std::cin >> number;
if (number % 2 == 0) {
std::cout << number << " is even." << std::endl;
}
- If-else statements:
The if-else statement extends the functionality of the if statement by offering an alternative code block to be executed if the initial condition is false. Its syntax is:
if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
Condition: This expression is evaluated, and the corresponding code block is executed based on the outcome.
Example: Determining the grade based on a student's score:
int score;
std::cout << "Enter your score (0-100): ";
std::cin >> score;
if (score >= 90) {
std::cout << "Grade: A" << std::endl;
} else if (score >= 80) {
std::cout << "Grade: B" << std::endl;
} else {
std::cout << "Grade: C or below" << std::endl;
}
- Nested conditionals:
Nested conditionals are those in which one conditional statement comes within another. Though powerful, this approach adds complexity and thus room for reduced readability, should their usage not be done mindfully.
Example: Checking if a number is positive, negative, or zero:
int number;
std::cout << "Enter a number: ";
std::cin >> number;
if (number > 0) {
std::cout << number << " is positive." << std::endl;
} else if (number < 0) {
std::cout << number << " is negative." << std::endl;
} else {
std::cout << number << " is zero." << std::endl;
}
Practical Applications of Conditionals:
- Validating user input: Ensure users enter data within acceptable ranges or specific formats, preventing unexpected program behavior.
- Making decisions based on user choices: Offer interactive options to users and tailor the program's behavior based on their selections.
- Controlling program flow based on dynamic conditions: Adapt the program's execution path depending on external factors, such as sensor readings or network connectivity.
- Implementing branching logic: Create intricate decision-making structures for complex algorithms or game mechanics.
By mastering conditionals, you unlock the potential to create programs that are not only efficient but also interactive and capable of adapting to different scenarios. The next section delves into essential practices and tips for effectively utilizing loops and conditionals in your programming endeavors.
How to Master Loops and Conditionals?
Since loops and conditionals are basic ingredients of programming, one should have crystal clear thoughts by now regarding these concepts. However, identifying best practices, common pitfalls, and strategies for effective debugging will take your skills one step further. This section makes you aware of them.
- Choosing the Right Tool for the Job: It is important to choose the correct type of loop when you find a task in which you wish to repeat everything. Here are some factors that you should consider:
- Definite vs. Indefinite Iteration: If the exact number of iterations to be performed inside the loop is known, then generally, we should use a for loop. However, when the desired number of iterations is not known prior, at that time, either a while loop or a do-while loop is used.
- Readability and Maintainability: While, under some conditions, both for loops and while loops give similar kinds of results, clearer code is formed by use of a for loop if the number of iterations of the loop is well-defined.
- Specific Iteration Needs: Do-while loops make sure that the code block in the loop is executed at least once, even when the initial condition is false. This can be quite handy for those cases where at least one iteration is mandatory.
By carefully considering these factors, you can choose the loop type that best suits the specific requirements of your program, enhancing both efficiency and code readability.
Exiting Early: Utilizing the break Statement
Loops provide the power of repetition, but it's critical to have mechanisms for early termination when necessary. The break statement allows you to prematurely exit a loop under certain conditions. Its syntax is:
for (...) {
// Code...
if (condition) {
break; // Exit the loop
}
// Code...
}
Using break judiciously can improve code efficiency and avoid unnecessary iterations, especially when certain conditions signal the completion of the task within the loop. However, overuse of break can make code harder to follow – strive for well-defined loop conditions that generally lead to natural loop completion.
Keeping Your Code Crystal Clear:
As your programs become more intricate, ensuring code readability becomes paramount. When crafting conditionals, prioritize clarity and maintainability with these practices:
- Meaningful Variable Names: Employ descriptive variable names that reflect their purpose and context. Avoid single-letter or cryptic names that obscure their meaning.
- Proper Indentation: Consistent indentation visually represents code blocks and the nesting of conditionals, making the flow of logic easier to comprehend.
- Comments: Use comments for explaining complex logic or code sections that are non-obvious when it is necessary to make the code clear for yourself and others.
With these practices, you can create conditionals that are functional, correct, and readable by someone else after a long time.
Common Pitfalls and Debugging Strategies
Even the most seasoned programmers encounter mistakes when working with loops and conditionals. Here are some common pitfalls to watch out for:
- Infinite Loops: Unintended infinite loops occur when the loop condition never becomes false, leading to the loop's indefinite execution. Ensure your loop condition eventually evaluates to false to allow the loop to terminate.
- Incorrect Loop Conditions: Mistakes in the loop condition can lead to the loop not iterating as intended or skipping certain elements. Carefully review your loop condition and verify its logic against your desired outcome.
- Logic Errors within Conditionals: Errors in the conditional expressions or the code within the conditional blocks can produce incorrect program behavior. Utilize debugging tools and print statements to trace variable values and identify the source of the error.
Debugging these issues requires a systematic approach. Here are some helpful tips:
- Utilize Print Statements: Add print statements at different points of time within the loop or conditional block to ascertain the value these variables may have in that particular point.
- Leverage Debugging Tools: Most integrated development environments (IDEs) have debugging tools that enable one to step through execution one line at a time, inspect variable values, and set necessary breakpoints. Use these features to try and pin down both where your mistake may be occurring and what type of mistake it might be.
- Break Down Complex Problems: Take complex problems that seem impossible and divide them into many small and quite possible parts. This action will not only make debugging much easier but also help you focus on sections of code where the problem may lie.
Knowing these typical mistakes, and using a proper debugging approach, will allow you to follow a systematic way to identify and rectify your code so that it makes the code right and efficient.
Conclusion:
Loops and conditionals are the essential building blocks of any programming language. By mastering these constructs, you gain the power to automate repetitive tasks, make choices within your programs, and manipulate program flow. This empowers you to write efficient and dynamic code that can handle a wide range of problems. Embrace the journey of learning! By dedicating time to exercise and exploration, you will soon discover yourself wielding this powerful equipment with self-assurance and creativity.
About the Author
Dr. Michael Hernandez
Qualification: Ph.D. in Cybersecurity and Software Engineering
Expertise: Renowned for expertise in secure software development and vulnerability analysis.
Research Focus: Specializes in developing secure software practices and tools to identify and mitigate vulnerabilities in software applications.
Practical Experience: Collaborated with organizations to implement secure coding practices, conduct vulnerability assessments, and develop security solutions for various software systems.
Dr. Hernandez is a leading expert in cybersecurity and software security, and his work has significantly contributed to protecting software applications