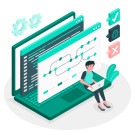
- 15th Nov 2023
- 21:51 pm
The Map interface in Java represents a collection of key-value pairs, with each key paired with a matching value. This data structure enables efficient value retrieval based on their related keys. The Map interface does not support duplicate keys and only one null key with multiple null values.
Key Features Map in Java
The Map interface's key features are as follows:
- Key-Value Association: Each key in the Map is assigned a value. Keys act as unique identifiers and are used to get the values associated with them.
- Implementation Training: Java supports several Map implementations, including HashMap, TreeMap, LinkedHashMap, and ConcurrentHashMap, each having unique attributes and use cases.
- Methods and Operations: Map interface methods such as put(key, value), get(key), remove(key), containsKey(key), and size() enable insertion, retrieval, deletion, and key existence testing.
It allows you to iterate over entries by utilizing the keySet(), entrySet(), or values() methods.
Because of its excellent O(1) average-case performance for common operations, the HashMap implementation is frequently used for general-purpose key-value storage. TreeMap keeps items in sorted order, but LinkedHashMap keeps insertion order.
Map interfaces are widely used in applications such as databases, caching systems, and whenever key-based data retrieval or association is required. They provide a versatile and efficient method of storing and managing key-value pairs in Java.
Characteristics of Map interface in Java
The Map interface in Java has numerous distinguishing features:
- Key-Value Pairs:
Maps are made up of key-value pairs, with each key corresponding to a certain value.
The linked value is accessed via the key, which must be unique inside the Map. Multiple keys, however, can map to the same value.
- Key Uniqueness:
Each key in a Map is unique; duplicate keys are not permitted. If an existing key is added, the prior mapping is replaced with the new one.
- Null Key and Multiple Null Values:
A Map can have a single null key.
It may store numerous null values and correlate duplicate null values with distinct keys.
- Variety of Implementations:
Java provides a number of Map interface implementations, each with its own set of attributes, performance, and behaviors.
HashMap, TreeMap, LinkedHashMap, and ConcurrentHashMap are examples of popular implementations.
- Iterating Over Entries:
Using methods like entrySet(), keySet(), or values(), map interfaces allow iteration over entries, keys, or values.
- Efficient Data Retrieval:
Maps allow for the quick retrieval of values based on their keys, making them a more efficient way to store and access key-based data.
- Flexibility and Use Cases:
Maps are commonly employed in scenarios requiring associative arrays, key-value pairs, or dictionary-like structures, such as databases, caching mechanisms, or applications requiring quick data retrieval based on keys.
Understanding these qualities aids in the selection of the suitable Map implementation depending on individual use cases, performance constraints, and data store and retrieval functionalities in Java programs.
Important methods in Java Map
Below are some important methods in the Java Map interface:
- put(K key, V value):
Inserts a key/value pair into the map. If the key already exists, the previously associated value is replaced with the new value.
Returns the key's previous value or null if the key was not previously existent.
- get(Object key):
- Returns the value associated with the given key. If the key is not found in the map, it returns null.
- remove(Object key):
Removes the key and its corresponding value from the map.
Returns the value associated with the deleted key, or null if the key was missing.
- containsKey(Object key):
Determines if the map includes the supplied key. If the key exists, returns true; otherwise, returns false.
- keySet():
Returns a Set view of all the keys contained in the map. This set allows iterating through the keys.
- entrySet():
Returns a Set view of the key-value mappings in the map. Each entry is represented as a Map.Entry containing both the key and its associated value.
- values():
Returns a collection view of all the values present in the map. This collection allows iterating through the values.
These methods are essential for maintaining and altering data within a Map, allowing for key insertion, retrieval, and removal, as well as offering views of keys, entries, and values for iterating over the map's content.
Types of Maps in Java
In Java, various Map implementations are available, each designed with specific characteristics and purposes. Here are some types of Maps:
- HashMap:
HashMap is a Java implementation of the Map interface that stores key-value pairs in a hash table. It allows for quick access and retrieval operations, with an average-case complexity of O(1) for typical operations like put and get. HashMap supports one null key and numerous null values, does not keep insertion order, and is unsynchronized, making it inappropriate for concurrent settings. Because of its efficiency and speed in discovering and manipulating items, it is commonly used for general-purpose key-value storage, making it excellent for applications requiring quick retrieval of data based on keys.
- TreeMap:
TreeMap is a Java implementation of the Map interface that stores data in a Red-Black tree. It keeps elements in sorted order based on keys, allowing for quick retrieval of sorted elements. TreeMap does not support null keys, but it does support multiple null values. It provides range views for key intervals and is especially handy when a sorted collection of key-value pairs is required. Its ordered nature makes it useful for applications that need data retrieval in sorted order. However, because to the added sorting complexity, its operations are somewhat slower than HashMap.
- LinkedHashMap:
In Java, LinkedHashMap is a Map interface implementation that extends HashMap. It preserves the order of insertion, allowing for predictable iteration order in accordance with the insertion sequence. Each item keeps a doubly linked list, which ensures that the order in which elements were put is kept. LinkedHashMap, like HashMap, allows for one null key and numerous null values. It's useful when iteration order is critical, since it ensures elements are accessible in the same order they were put. This approach combines the rapid access of a hash table with the predictable iteration order, making it appropriate for applications where insertion order must be maintained.
- ConcurrentHashMap:
ConcurrentHashMap in Java is a synchronized and thread-safe Map interface implementation built for concurrent access. It enables numerous threads to conduct read and write operations concurrently without blocking, resulting in high concurrency. ConcurrentHashMap does this by segmenting the map and enabling various threads to access separate portions concurrently. It avoids locking the entire map for read operations, which improves efficiency and performance in concurrent situations. It allows some changes to happen without synchronization, increasing throughput and making it an excellent choice for applications that require thread safety and performance in cases where many threads are altering the map at the same time.
Each Map implementation has unique features and benefits, allowing developers to select the best one depending on needs like as efficiency, concurrency, ordering, or particular use cases requiring enums. Understanding the features and behavior of different Map implementations is critical for picking the best-fit Map for a particular job.
Here are various types of maps in Java along with brief explanations in a tabular format:
Map Type | Explanation |
HashMap | Implements the Map interface using a hash table, offering quick retrieval and insertion. Allows one null key and multiple null values. |
TreeMap | Implements the NavigableMap interface using a Red-Black tree, maintaining elements in a sorted order. Useful for range-based queries and sorted key-value pairs. |
LinkedHashMap | Extends HashMap with an additional feature of maintaining the insertion order, useful for iteration in the order in which entries were added. |
ConcurrentHashMap | Implements a thread-safe map using a hash table, providing concurrency without the need for external synchronization. |
EnumMap | Specifically designed to use enum type as keys, offering a highly efficient map implementation when keys are enum constants. |
WeakHashMap | Implements a hash table using weak keys, allowing keys that are only weakly referenced, useful in scenarios where cached entries are no longer needed. |
These different map implementations in Java serve specific purposes and have unique features catering to various requirements. Choosing the right map type is crucial based on factors such as performance, iteration order, concurrency, and the nature of keys to be used within the map.