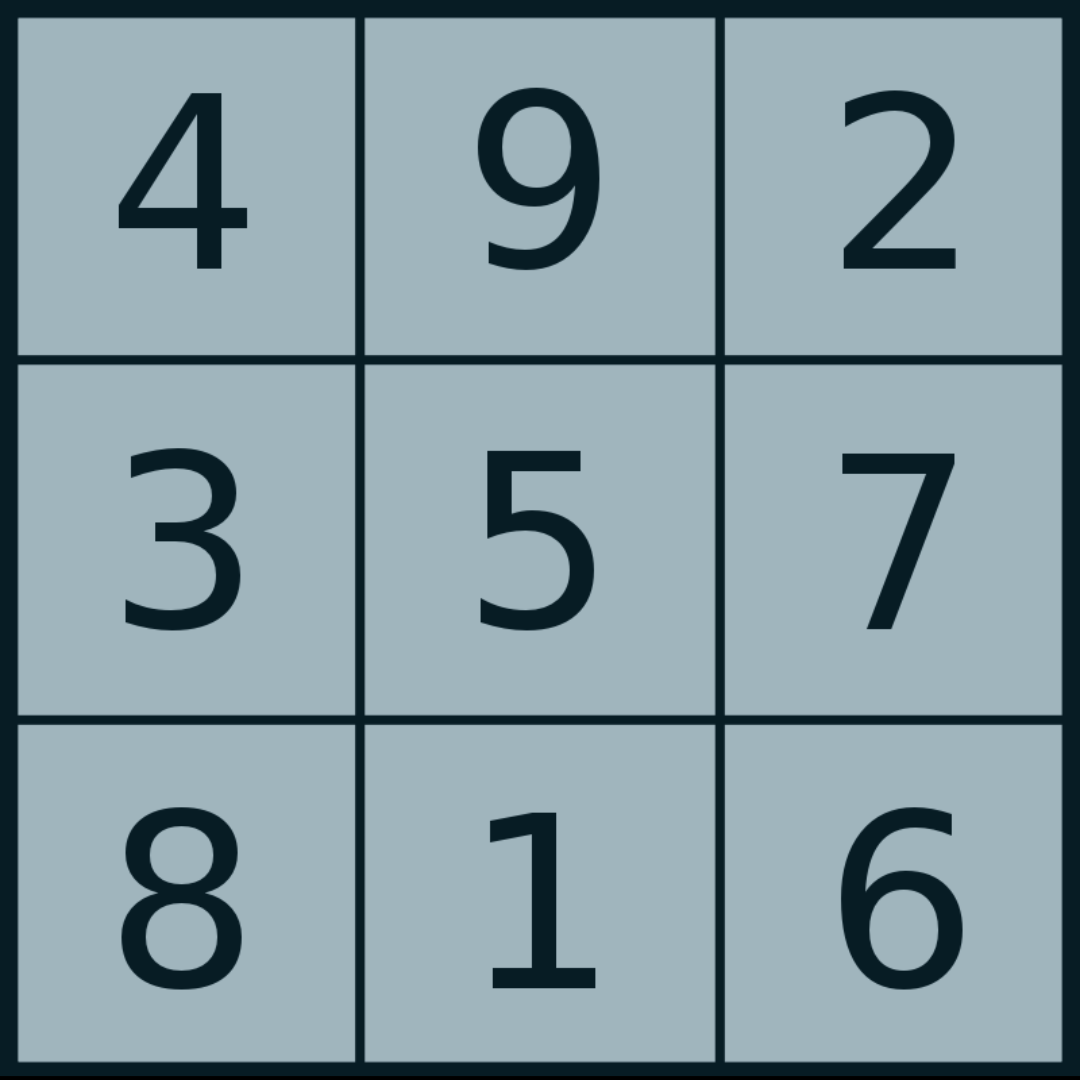
- 5th Apr 2023
- 09:21 am
Python Homework Help
Python Homework Question
Python Logical Puzzles, Games, and Algorithms: Lo Shu Magic Square
Python Homework Solution
The Lo Shu Magic Square is a grid of nine squares, traditionally filled with the numbers 1 through 9. The challenge is to arrange the numbers such that each row, column, and diagonal adds up to the same number (15).
Here's a Python code to check if a given square is a Lo Shu Magic Square:
def is_lo_shu_magic_square(square):
# Check if all rows, columns, and diagonals add up to 15
row1_sum = sum(square[0])
row2_sum = sum(square[1])
row3_sum = sum(square[2])
col1_sum = square[0][0] + square[1][0] + square[2][0]
col2_sum = square[0][1] + square[1][1] + square[2][1]
col3_sum = square[0][2] + square[1][2] + square[2][2]
diag1_sum = square[0][0] + square[1][1] + square[2][2]
diag2_sum = square[0][2] + square[1][1] + square[2][0]
if row1_sum == row2_sum == row3_sum == col1_sum == col2_sum == col3_sum == diag1_sum == diag2_sum == 15:
return True
else:
return False
The function is_lo_shu_magic_square takes a 2-dimensional list representing the square as its input parameter. It checks if all the rows, columns, and diagonals add up to 15, and returns True if it is a Lo Shu Magic Square, and False otherwise.
Here's an example of how to use the function:
# Example square
square = [[4, 9, 2], [3, 5, 7], [8, 1, 6]]
# Check if square is a Lo Shu Magic Square
if is_lo_shu_magic_square(square):
print("The square is a Lo Shu Magic Square!")
else:
print("The square is not a Lo Shu Magic Square.")
Output:
The square is a Lo Shu Magic Square!