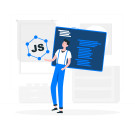
- 29th Nov 2023
- 15:38 pm
JavaScript, renowned for its versatility, supports a diverse range of data types crucial for effective programming. These types can be broadly categorized into primitive and non-primitive data types. The primitive types, such as numbers, strings, booleans, null, undefined, symbols, and bigints, provide fundamental building blocks. Conversely, non-primitive types, exemplified by objects, present a more intricate and flexible structure. Grasping and utilizing these types is essential for unlocking the complete potential of JavaScript, enabling developers to craft dynamic and feature-rich applications effortlessly.
Primitive Data Types
JavaScript, as a dynamically typed language, boasts several primitive data types that serve as the foundational elements in coding. These fundamental types are crucial for developers, offering a range of capabilities essential for effective coding.
- Number: JavaScript handles numeric data seamlessly, supporting both integer and floating-point values. This flexibility allows developers to perform arithmetic operations effortlessly. Numbers in JavaScript are of the IEEE 754 floating-point format, and operations involving them may lead to precision issues.
let myNumber = 42;
- String: Strings represent textual data and are essential for manipulating and displaying information. JavaScript provides robust string-handling capabilities, enabling text processing and manipulation. Strings can be enclosed in single (') or double (") quotes.
let myString = 'Hello, World!';
- Boolean: Booleans are binary data types, representing either true or false. They play a pivotal role in control flow and decision-making within JavaScript programs. Boolean values result from logical operations or comparisons.
let isTrue = true;
- Null: The null type indicates the absence of a value. While often used intentionally, developers must be cautious about potential null references to avoid unintended consequences. It is an assignment value that represents no value or no object as its value.
let myNull = null;
- Undefined: Similar to null, undefined signifies the absence of a defined value. It often serves as the default value for variables that have been declared but not initialized. Variables without an assigned value automatically contain undefined.
let myUndefined;
- Symbol: Introduced in ECMAScript 6, symbols are unique and immutable data types, commonly used to create private object properties and avoid naming conflicts. Symbols are created using the Symbol() constructor.
const mySymbol = Symbol('description');
- BigInt: BigInt allows JavaScript to represent and perform operations on integers of arbitrary precision. BigInts prove especially valuable when handling very large numbers. To create BigInts, append "n" to the end of an integer literal or use the BigInt() constructor.
const bigNumber = 9007199254740991n;
Non-Primitive Data Types
Beyond primitive types, JavaScript programming also incorporates non-primitive data types, adding versatility to the language. Non-primitive types are mutable and include objects, which serve as containers for key-value pairs and other complex data structures. Understanding these types enhances developers' ability to build sophisticated applications with dynamic functionality.
- Object: In JavaScript, the Object type stands out as a versatile non-primitive data type, empowering developers to construct and manipulate intricate structures by amalgamating diverse key-value pairs. Objects play a fundamental role in enhancing JavaScript's flexibility and providing support for sophisticated data structures.
let myObject = { key: 'value', anotherKey: 42 };
Type Coercion in JavaScript:
JavaScript, being a dynamically-typed language, allows for implicit type conversion, making it flexible but sometimes prone to unexpected behaviors. Developers often use techniques like the “typeof” operator and other methods to check variable types and ensure proper data manipulation and flow in their code.
let num = 42;
let str = '3';
console.log(num + str); // Implicit coercion: "423"
console.log(num + Number(str)); // Explicit coercion: 45
Type Checking in JavaScript:
In JavaScript, type checking plays a crucial role in maintaining code integrity and preventing unexpected errors. Developers commonly use the “typeof” operator, “instanceof” operator, and other techniques to check the types of variables and ensure consistent and reliable behavior across their applications. These mechanisms contribute to code robustness by allowing developers to anticipate and handle different data types effectively.
let example = 'Hello';
console.log(typeof example); // Output: "string"
console.log(example instanceof String); // Output: false
Type Conversion in JavaScript:
Type conversion, or type coercion, in JavaScript involves transforming a value from one data type to another. This process can occur explicitly when developers intentionally convert values using functions like String(), Number(), or Boolean(). Additionally, type conversion can happen implicitly when JavaScript automatically converts values in certain situations. Understanding and managing type conversions is crucial for writing reliable and predictable JavaScript code.
let numString = '42';
let num = Number(numString); // Explicit conversion
console.log(num + 3); // Output: 45
Dynamic Typing in JavaScript:
JavaScript is a dynamically typed language, allowing variables to hold values of any data type. Unlike statically typed languages where variable types are declared explicitly, JavaScript's dynamic typing offers flexibility but requires careful consideration to prevent unexpected behavior. The interpreter determines the variable type during runtime, enabling more adaptable and concise code.
let dynamicVariable = 42;
dynamicVariable = 'Now a string';