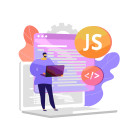
- 29th Nov 2023
- 14:57 pm
- Admin
In the rapidly evolving field of web development, the quest for dynamic and responsive user experiences has propelled the demand for JavaScript Asynchronous Programming. JavaScript, being the language of the web, provides robust mechanisms for handling asynchronous operations, empowering developers to build efficient and non-blocking applications.
What is JavaScript Asynchronous Programming?
JavaScript Asynchronous Programming is a paradigm that facilitates the execution of non-blocking code, enabling concurrent operations without waiting for each to finish before proceeding to the next. Unlike traditional synchronous programming, where tasks are executed sequentially, potentially causing delays, asynchronous programming in JavaScript employs mechanisms such as callbacks, promises, and the async/await syntax.
This approach improves the responsiveness of applications, allowing tasks like data fetching, file operations, or network requests to be initiated without bringing the entire program to a halt. The event loop and concurrency model play pivotal roles in efficiently managing asynchronous tasks.
Callback Functions
Callback functions serve as the foundational building blocks of asynchronous programming in JavaScript. Callback functions in JavaScript are functions passed as arguments to other functions, intended to be executed later when specific events or conditions occur. While callback functions offer a solution for handling asynchronous tasks, nesting them excessively can result in a situation known as "callback hell." This phenomenon makes the code intricate and difficult to maintain.
Let's examine the following example:
getDataFromAPI((result) => {
processData(result, (processedData) => {
displayData(processedData, () => {
// More nested callbacks...
});
});
});
Addressing callback hell can be achieved by leveraging promises, providing a more structured and readable approach to handling asynchronous operations.
Promises in JavaScript
JavaScript incorporated promises to alleviate the challenges linked with callback hell. A promise signifies the eventual completion or failure of an asynchronous operation, enabling developers to attach callbacks to manage the outcomes. Promises have three states: pending, fulfilled, and rejected.
const fetchData = new Promise((resolve, reject) => {
// Asynchronous operation
if (operationSuccessful) {
resolve(result);
} else {
reject(error);
}
});
fetchData
.then((result) => {
// Handle the result
})
.catch((error) => {
// Handle errors
});
Promises enable a more structured and readable way to handle asynchronous operations. They also facilitate chaining, where multiple asynchronous tasks can be executed sequentially.
Async/Await Syntax
ES6 introduced the async/await syntax, further simplifying asynchronous code. Async functions, marked by the async keyword, return promises and allow the use of the await keyword within their body to pause execution until the promise is settled.
async function fetchData() {
try {
const result = await asynchronousOperation();
// Handle the result
} catch (error) {
// Handle errors
}
}
The async/await syntax introduces a code structure that resembles synchronous programming while preserving the advantages of asynchronous operations.
Event Loop and Concurrency Model
The event loop is a crucial concept in understanding how JavaScript handles asynchronous operations. It guarantees the efficient management of non-blocking operations, like I/O operations or timers, without causing the entire application to freeze. The JavaScript concurrency model, centered around the event loop, enables the concurrent execution of multiple tasks, enhancing the language's efficiency.
Handling Multiple Promises Concurrently
JavaScript provides methods like Promise.all() and Promise.race() to handle multiple promises concurrently. Promise.all() waits for all promises to be resolved, while Promise.race() resolves or rejects as soon as one promise settles.
const promise1 = fetch('https://api.example.com/data1');
const promise2 = fetch('https://api.example.com/data2');
Promise.all([promise1, promise2])
.then((results) => {
// Handle results
})
.catch((error) => {
// Handle errors
});
These methods enable efficient management of multiple asynchronous tasks, contributing to improved application performance.
Generators and Asynchronous Iteration
Generators, a feature introduced in ES6, provide an alternative method for managing asynchronous operations. They enable the pausing and resuming of a function's execution, making them well-suited for asynchronous iteration.
function* asynchronousGenerator() {
const result1 = yield asynchronousOperation1();
const result2 = yield asynchronousOperation2();
// Handle results
}
const generator = asynchronousGenerator();
generator.next().value.then((result1) => {
generator.next(result1).value.then((result2) => {
// Handle final result
});
});
Generators provide a unique way to structure asynchronous code, particularly when dealing with asynchronous sequences.
Applications of JavaScript Asynchronous Programming:
JavaScript's asynchronous programming finds crucial applications across various domains, reshaping how tasks are handled in web development.
- Web Development: Asynchronous programming is extensively used in web development to create responsive and dynamic user interfaces. Tasks like fetching data from servers, handling user interactions, and updating the DOM can be performed asynchronously, preventing the user interface from freezing during lengthy operations.
- HTTP Requests: Managing HTTP requests is a common application of asynchronous programming. With AJAX (Asynchronous JavaScript and XML) or modern Fetch API, web applications can make requests to servers without blocking the entire execution, enabling smooth user experiences.
- File Operations: Asynchronous programming is beneficial for handling file operations in web applications. Reading and writing files, especially in scenarios like uploading or downloading, can be executed asynchronously to maintain a seamless user experience.
- Timers and Intervals: JavaScript allows the scheduling of tasks using timers and intervals. Asynchronous programming ensures that scheduled functions run without disrupting the overall flow of the application. This is crucial for animations, real-time updates, and other time-dependent tasks.
Advantages of JavaScript Asynchronous Programming:
Adopting asynchronous programming in JavaScript brings a multitude of benefits, enhancing the performance and responsiveness of web applications.
- Improved Performance: Asynchronous programming prevents blocking operations, enhancing the overall performance of web applications. It ensures that time-consuming tasks don't hinder the responsiveness of the user interface.
- Enhanced User Experience: By handling tasks such as data fetching or file operations asynchronously, the user experience is improved. Applications remain responsive, and users can interact with the interface without delays.
- Concurrent Operations: Asynchronous programming empowers the simultaneous execution of multiple operations, offering notable advantages in efficiently managing numerous tasks and optimizing system resource utilization.
- Efficient Task Scheduling: Timers and intervals, key components of asynchronous programming, facilitate efficient scheduling of tasks. This is crucial for scenarios where certain operations need to be performed at specific intervals.
Disadvantages of JavaScript Asynchronous Programming:
However, incorporating asynchronous programming into JavaScript comes with its own set of challenges.
- Complexity: Asynchronous programming adds intricacies, especially when dealing with callback functions or orchestrating the flow of asynchronous code. This complexity can pose challenges for code comprehension and maintenance.
- Callback Hell (Pyramid of Doom): Excessive nesting of callbacks, commonly known as "Callback Hell" or the "Pyramid of Doom," is a prevalent issue in asynchronous code. This not only diminishes code readability but also presents difficulties in terms of maintenance.
- Error Handling: Managing errors effectively in asynchronous code can be intricate. Robust error handling becomes imperative to prevent issues such as unhandled promise rejections, which can negatively impact the application.
- Debugging: Debugging asynchronous code may be more intricate than debugging synchronous code. Identifying the sequence of asynchronous operations and isolating issues might require additional effort.