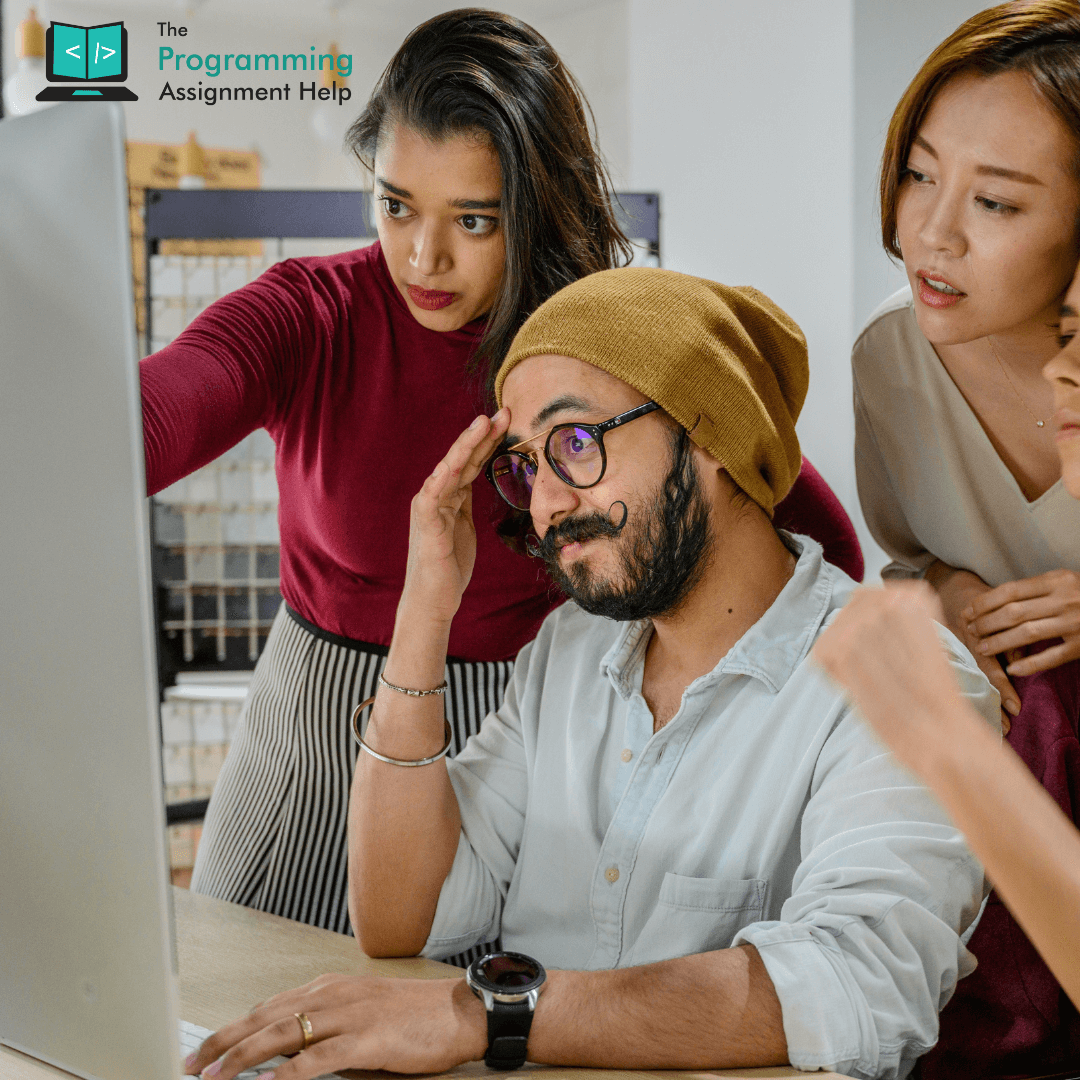
- 9th Jun 2022
- 03:11 am
1.a.
Scope of the variable is the part of the program in which it can be accessed. In Java, variables
can have Class level, Method level and Block level scope. In class level, its variables should be declared
inside class and not inside any function of the class, so they can be used anywhere inside class.
public class Class_level {// member variables int a; char b; }
In Method level, variables are defined inside a method and can’t be accessed outside that method.
public class Method_level { void test() { // local variable int x; } }
In block level, variables are defined inside a pair of flower brackets “{” and “}” in any method and the
variables have scope inside those the brackets.
public class Block_level { for (int i = 0; i < 10; i++) // i is loop variable and has scope inside for loop { System.out.println(i); } // cant access i here, shows error System.out.println(i); }
1.b. Creating a variable is called a variable declaration. To declare a variable, you must specify the data
type and a unique name for it. When you declare a variable, a memory location is set aside for a variable
of that type and the name is associated with that location.For an integer variable 32 bits, for double 64 bits
and for boolean 1 bit of space in memory is allocated. So, declaration gives the type and name of the
variable. Initialization means assigning the value while declaration.