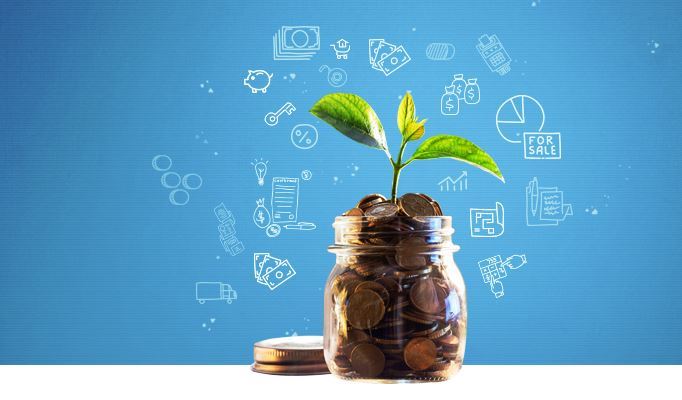
- 11th May 2019
- 23:42 pm
- Ali Akavis
Here's a Java program that reads in the investment amount, annual interest rate, and number of years from the user and displays the future investment value:
import java.util.Scanner;
import java.text.DecimalFormat;
public class FutureInvestmentValue {
public static void main(String[] args) {
// Taking inputs from user
Scanner sc = new Scanner(System.in);
System.out.print("Enter investment amount: ");
double investmentAmount = sc.nextDouble();
System.out.print("Enter annual interest rate (as a percentage): ");
double annualInterestRate = sc.nextDouble();
System.out.print("Enter number of years: ");
int numberOfYears = sc.nextInt();
// Calculating future investment value
double monthlyInterestRate = annualInterestRate / 12 / 100; // Convert annual interest rate to monthly rate
double futureValue = investmentAmount * Math.pow(1 + monthlyInterestRate, numberOfYears * 12);
// Defining DecimalFormat to round off result to 2 decimals
DecimalFormat twoDForm = new DecimalFormat("#.##");
// Displaying the result
System.out.println("Future investment value is
In this program:
- We use a
Scanner
to read inputs from the user for the investment amount, annual interest rate, and number of years. - The future investment value is calculated using the formula
FV = P * (1 + r/12)^n
, whereFV
is the future value,P
is the investment amount,r
is the annual interest rate (converted to a monthly rate), andn
is the number of years. - We use a
DecimalFormat
object to round off the result to two decimal places and then display the future investment value.
About the Author - Seddy
With a fervent dedication to decoding complex datasets and crafting innovative solutions, I am a seasoned programmer proficient in Statistical Data Analysis, Statistics, Statistical Modeling, and programming. My expertise lies at the intersection of technical acumen and analytical prowess, allowing me to engineer dynamic solutions that empower organizations to make data-driven decisions.