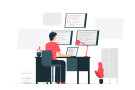
- 16th Nov 2023
- 15:44 pm
- Admin
In Java, Inheritance is a fundamental Object-Oriented Programming (OOP) concept where a new class (subclass or child) inherits attributes and behaviors from an existing class (superclass or parent). It promotes code reuse, allowing the subclass to extend or override functionalities from the superclass, fostering a hierarchical structure.
The keyword "extends" is used to declare inheritance, linking the subclass to its superclass. Java supports single inheritance, meaning a class can inherit from only one superclass. This limitation is mitigated by interfaces, allowing a class to implement multiple interfaces and achieve a form of multiple inheritance.
In addition to promoting code reusability, inheritance facilitates the implementation of polymorphism, enabling objects of the subclass to be treated as instances of the superclass. Method overriding, a key feature of inheritance, empowers subclasses to provide specific implementations for methods defined in the superclass.
Abstract classes and interfaces further extend the versatility of inheritance, offering abstract blueprints and multiple inheritance capabilities. In Java, a subtle yet powerful aspect of inheritance lies in the implicit extension of every class from the Object class, forming the foundation for the entire class hierarchy.
Understanding and effectively implementing inheritance is essential for building modular, scalable, and maintainable Java applications.
Concepts of Inheritance In Java
Here's the key concept of inheritance in Java presented in a tabular format:
- Superclass and Subclass: Definition of existing classes (superclass) and the creation of new classes (subclass) inheriting attributes and methods.
- "is-a" Relationship: Understanding how inheritance models an "is-a" relationship, indicating that a subclass is a specialized form of its superclass.
- Code Reusability: Leveraging inherited fields and methods for efficient and reusable code development.
- Access to Superclass Members: Exploring how subclasses have access to public and protected members of the superclass.
- Method Overriding: Learning the ability of subclasses to provide specific implementations for methods defined in the superclass.
Application Of Inheritance In Java
Applications of Inheritance in Java explained by our experts are:
- Code Reusability: Inherited attributes and methods allow developers to reuse code from existing classes, promoting efficiency.
- Polymorphism: Inheritance supports polymorphism, enabling objects of the subclass to be treated as objects of the superclass.
- Method Overriding: Subclasses can provide specific implementations for methods defined in the superclass, allowing customization.
- Base Class for Frameworks: Superclasses serve as base classes in frameworks, providing foundational functionality for specialized subclasses.
- Software Design Patterns: Inheritance facilitates the implementation of design patterns like Factory, Decorator, and Observer.
- Extending Libraries: Developers extend existing libraries by creating subclasses, and adding functionality while leveraging existing code.
- Organizing Code: Inheritance creates a clear and hierarchical structure in code, making it more organized and readable.
- Modeling Real-world Relationships: Inheritance models "is-a" relationships, representing real-world connections between entities.
- Template Method Pattern: Superclasses define a template with methods, allowing subclasses to override specific steps while maintaining the overall structure.
- Reducing Redundancy: Common functionalities are defined in a superclass, reducing redundancy and promoting maintainability.
In Java, inheritance is a versatile concept, providing a foundation for building scalable, modular, and maintainable software systems. It plays a crucial role in enhancing code organization and fostering reusability.
Challenges Faced By Students In Solving Inheritance In Java
Here are some Challenges Faced by Students in Learning Inheritance in Java:
- Conceptual Grasp: Understanding superclass-subclass relationships and the conceptual nuances of inheritance.
- Method Overriding Complexity: Grasping the intricacies of method overriding, including rules and implementation scenarios.
- Single Inheritance Limitation: Dealing with the limitation of Java supporting single inheritance.
- Hierarchy Design Challenges: Balancing reusability and specialization when designing effective class hierarchies.
- Understanding Object Class: Grasping the implicit inheritance from the Object class and its methods.
- Applying Inheritance in Real-world Scenarios: Effectively applying inheritance to model real-world scenarios.
- Balancing Code Reusability and Complexity: Striking a balance between maximizing code reusability and avoiding unnecessary complexity.
- Maintaining Code Readability: Ensuring that an inherited codebase remains readable and understandable.
- Understanding Access Modifiers: Grasping the impact of access modifiers (public, private, protected) on inherited members.
- Transition from Procedural to OOP Thinking: Shifting from procedural to Object-Oriented thinking, especially for students with prior programming experience.
How The Programming Assignment Help Can Assist In Inheritance In Java Assignment?
Our goal is to provide comprehensive support, addressing challenges at various levels of difficulty, and ensuring that students gain a deep understanding of inheritance in Java for successful assignment completion.
- Expert Guidance: Our team of experienced Java developers offers expert guidance on understanding and implementing inheritance concepts.
- Concept Clarification: We assist in clarifying conceptual challenges, ensuring a solid understanding of superclass-subclass relationships.
- Code Implementation: Our experts help translate inheritance concepts into well-structured Java code, ensuring correctness and efficiency.
- Method Overriding Support: Assistance in navigating the complexities of method overriding, including rules and best practices.
- Hierarchy Design Assistance: Guidance on designing effective class hierarchies, striking a balance between reusability and specialization.
- Real-world Application Guidance: Practical examples and guidance on applying inheritance to model real-world scenarios effectively.
- Code Reusability Strategies: Strategies for maximizing code reusability while managing complexity, and promoting best practices.
- Code Readability Tips: Tips and best practices for maintaining code readability in an inherited codebase.
- Access Modifiers Explanation: Clarification on the impact of access modifiers on inherited members, ensuring a clear understanding.
- Transition Support: Assistance in transitioning from procedural to Object-Oriented thinking, with practical examples and exercises.