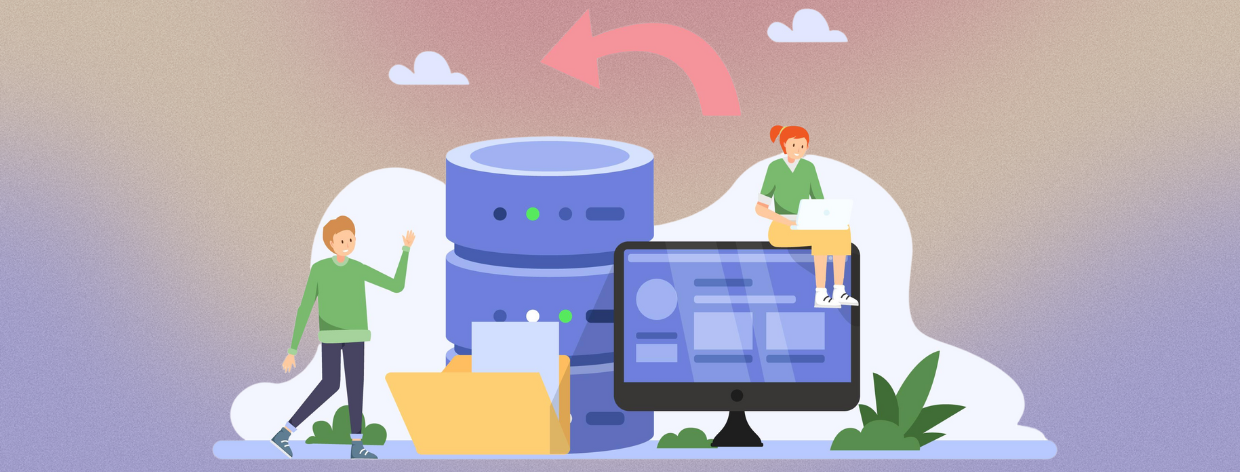
- 16th Feb 2024
- 23:01 pm
- Admin
As software projects evolve, maintaining code structure and integrity becomes vital. This is where modularity emerges, a principle that breaks down code into well-defined, reusable units. In Python, classes serve as the foundational building blocks for creating objects. However, when projects grow complex, managing numerous classes within a single file becomes challenging. This is where the concept of importing classes from other files proves invaluable.
Imagine constructing a grand building. Individual bricks (classes) form the base, but haphazard placement wouldn't create a coherent structure. Organizing these bricks into dedicated sections (files) enhances construction manageability. Similarly, importing classes empowers you to:
- Decompose Large Projects: Break down complex applications into smaller, focused modules. Each module acts as a designated area for specific classes, improving organization and clarity.
- Boost Reusability: Define a class once within a file and import it where needed, eliminating redundancy and simplifying maintenance. Think of building a versatile toolkit where each tool serves a distinct purpose and can be readily reused across different projects.
- Complexity: As projects grow, managing sprawling code becomes intricate. Importing classes compartmentalizes concerns, making code easier to navigate, understand, and update. It's like organizing your toolbox – specific tools remain grouped, readily accessible when required.
- Foster Collaboration: Well-structured code facilitates smoother teamwork. Importing classes promotes better collaboration by clearly defining boundaries and enabling shared code usage. Think of it as dividing tasks among team members, each responsible for their assigned module.
By strategically importing classes, you unlock a powerful approach to organizing and managing Python code. This not only enhances maintainability and reusability but also lays the groundwork for clearer collaboration and efficient project development. Let's now delve deeper into the mechanics of this powerful technique.
What is Import Classes from Another File in Python?
Large Python projects can quickly become unwieldy when numerous classes cram into a single file. Importing classes from other files offers a strategic solution, boosting organization and code quality.
This approach lets you break down big projects into focused modules, each acting as a dedicated home for specific classes. This modularity unlocks several advantages:
- Reuse with Ease: Define a class once, import it wherever needed, cutting down on code duplication and simplifying maintenance. Think of building a versatile toolbox where each tool serves a unique purpose and can be readily used again in different projects.
- Effortless Maintenance: Modular code lets you update and modify specific areas without accidentally affecting the entire project. Imagine dedicated sections within your codebase, easily accessible and manageable even as it grows.
- Teamwork Made Easy: Clear responsibilities through defined modules enable smoother collaboration. Each developer works on their assigned area while understanding how it interacts with others through well-defined class imports.
- Healthy Code: The benefits go beyond the organization. By promoting modularity and reuse, this approach naturally encourages established Python coding practices, leading to cleaner, more maintainable code that's easier to understand, debug, and modify in the long run.
Importing classes in Python is more than just a technical trick; it embodies a core principle of good software design. By embracing this modular approach, you unlock the potential for efficient project management, streamlined updates, and a more enjoyable programming experience for your team.
Importing Classes from Another File in Python: Syntax and Methods
In Python, the ability to import classes from other files plays a crucial role in structuring and managing complex projects. This approach promotes modularity, code reuse, and maintainability, allowing developers to organize their code effectively. Here, we delve into the different syntaxes and methods for importing classes, highlighting their nuances and best practices.
1. Importing by Full Name:
This method offers the most explicit approach, clearly identifying the source of the class:
import module_name
class_instance = module_name.ClassName()
Advantages:
- Clarity: Makes it clear where the class originates, especially for infrequent use or unfamiliar modules.
- No naming conflicts: Reduces the risk of collisions with variables or classes in the current scope.
Disadvantages:
- Verbosity: Can be cumbersome for frequently used classes or long module names.
- Repetitive: Requires typing the module name repeatedly, reducing readability.
Best Use Case:
- Importing classes from third-party modules or modules used less frequently.
2. Importing by Alias:
For frequently used classes or modules, importing with an alias offers a concise alternative:
from module_name import ClassName as AliasName
alias_instance = AliasName()
Advantages:
- Readability: Improves code readability by reducing verbosity.
- Convenience: Makes typing class names faster and more convenient.
Disadvantages:
- Potential for naming conflicts: Overusing aliases can increase the risk of conflicts with other variables or classes.
- Reduced clarity: Might introduce ambiguity for unfamiliar developers unless the alias choice is clear.
Best Use Case:
- Importing frequently used classes from within your project or well-known third-party modules.
3. Using Relative Imports:
When working with multiple modules within a project structure, relative imports become essential. These specify the relative path to the module containing the desired class:
from .subfolder import submodule # Import submodule from current directory
from ..other_module import AnotherClass # Import AnotherClass from parent directory
Advantages:
- Flexibility: Adapts easily to changes in project structure as directories evolve.
- Maintainability: Makes code more maintainable by reflecting the physical location of modules.
Disadvantages:
- Complexity: Can become complex in deeply nested directory structures.
- Circular dependencies: Avoid circular dependencies where modules import each other, leading to potential issues.
Best Use Case:
- Working with multiple modules within a project, especially those organized in a hierarchical structure.
Importing Classes with Examples
The power of importing classes comes alive through practical usage. Let's explore several code examples showcasing different scenarios:
- Simple Class with Basic Attributes and Methods:
Imagine a Point class defined in a geometry.py module:
# geometry.py
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def distance_to_origin(self):
return (self.x**2 + self.y**2)**0.5
# main.py
from geometry import Point
point1 = Point(3, 4)
distance = point1.distance_to_origin()
print(distance) # Output: 5.0
Here, main.py imports the Point class directly using its module name and class name. This approach is suitable for basic classes and infrequent use.
- Class with Inheritance and Polymorphism:
Consider a Shape class with subclasses Circle and Rectangle in shapes.py:
# shapes.py
class Shape:
def __init__(self, color):
self.color = color
def area(self):
raise NotImplementedError
class Circle(Shape):
def __init__(self, color, radius):
super().__init__(color)
self.radius = radius
def area(self):
return 3.14 * self.radius**2
class Rectangle(Shape):
def __init__(self, color, width, height):
super().__init__(color)
self.width = width
self.height = height
def area(self):
return self.width * self.height
# main.py
from shapes import Circle, Rectangle
circle = Circle("blue", 2)
rectangle = Rectangle("red", 5, 3)
print(circle.area()) # Output: 12.56
print(rectangle.area()) # Output: 15
shapes = [circle, rectangle] # Polymorphism!
for shape in shapes:
print(f"Shape color: {shape.color}, area: {shape.area()}")
This example showcases importing specific classes (Circle, Rectangle) and utilizing inheritance for code reuse and polymorphism.
- Handling Circular Imports (with Caution):
Circular imports happen when modules import each other. While generally discouraged, consider this (carefully managed) example:
# module1.py
from module2 import Class2
def function1(arg):
return Class2().method2(arg)
# module2.py
from module1 import function1
def function2(arg):
return function1(arg * 2)
# main.py
from module1 import function1
from module2 import function2
result = function2(5)
print(result) # Output: 20
Here, function1 depends on Class2 from module2, and function2 depends on function1. Though functional, such dependencies can lead to complex execution order and are best avoided in larger projects.
Importance of Clear Structure and Naming:
Throughout these examples, remember the significance of clear module and class names. Descriptive names convey purpose and maintainability. Utilize consistent directory structures and relative imports when applicable. By following these best practices, you empower yourself and your team to work efficiently with the power of importing classes in Python.
Common Challenges and Best Practices
Importing classes in Python unlocks modularity and organization, but pitfalls lurk along the way. Let's explore common mistakes and best practices to ensure smooth sailing in your coding journey.
Challenges and Troubleshooting:
- ModuleNotFoundError: This error arises when Python cannot locate the imported module. Double-check file paths, relative import structures, and ensure correct module names. Remember, Python considers case sensitivity!
- Circular Imports: When modules import each other directly, creating a circular dependency, execution order becomes unpredictable. Refactor code to break these dependencies by introducing intermediary modules or utilizing lazy imports.
- Overloaded Names: Importing multiple classes with the same name from different modules creates naming conflicts. Use aliases or specific module prefixes to clarify which class you're referencing.
Best Practices for Effective Imports:
- Context is Key: Choose the appropriate import method based on context. Use full name import for clarity when unfamiliar or rarely used. Opt for aliases for frequently used classes within your project. Leverage relative imports when working with multiple modules.
- Focus on Modularity: Avoid creating large monolithic files. Break down complex functionalities into smaller, focused modules, each containing related classes. This improves code organization, maintainability, and reusability.
- Clear Documentation: Document module and class usage within your codebase. Utilize docstrings, comments, and clear naming conventions to make your code understandable for yourself and others.
Additional Tips:
- Follow consistent import formatting guidelines within your project. Use tools like linters and code formatters to enforce these conventions.
- Consider leveraging __init__.py files in directories to create packages, further structuring your project hierarchy.
- When dealing with large projects, explore advanced techniques like import hooks and package managers for efficient import management.
Applications and Use Cases of Import Classes from Another File in Python
The ability to import classes in Python extends beyond theoretical concepts; it empowers developers to tackle real-world projects effectively. Here's how:
Reusable Components and Libraries:
- Build adaptable components across projects by defining classes in modules and importing them as needed.
- Create libraries of UI elements, data processing tools, or mathematical functions.
Organizing Large Projects:
- Break down complex projects into focused modules with specific functionalities.
- Manage sprawling codebases easily through modular organization.
- Imagine separate modules for user authentication, database interactions, and templating in a web application.
Separating Concerns and Maintainability:
- Promote separation of concerns for improved code maintainability.
- Isolate functionalities within modules for targeted updates and modifications.
- Work on specific features efficiently without affecting unrelated code sections.
Collaboration and Team Efficiency:
- Facilitate effective teamwork through clear code organization.
- Enable team members to work on assigned modules with defined class imports.
- Streamline development, reduce confusion, and promote shared code ownership.