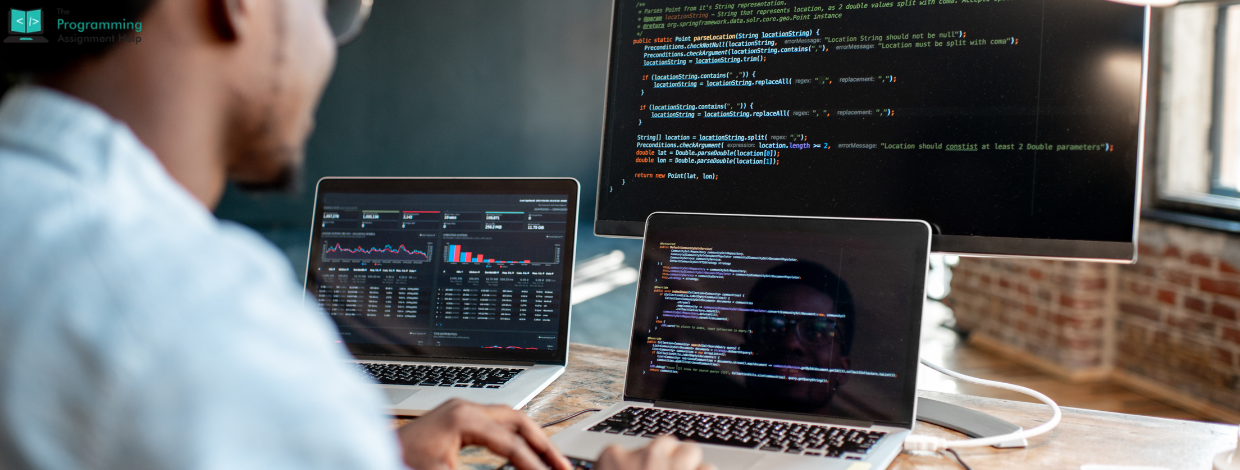
- 25th Sep 2024
- 18:12 pm
- Riana Hen
Iterating through a map in C is an important task, especially when working with key-value pairs. Unlike higher-level languages like Java or Python, C doesn’t come with a built-in map structure. Instead, you need to manually create one using data structures such as arrays, linked lists, or hash tables. This adds a bit more complexity, but it's a valuable skill for efficiently managing data in C.
What is a Map?
A map is a set of key-value pairs, where each key is unique and corresponds to a specific value. Maps are helpful when you need to quickly find or update data using a specific key. In C, you can implement a map using a hash table, which offers fast lookups by distributing data across an array of “buckets” using a hash function.
Iterating Over a Map in C
Iterating over a map in C is a more hands-on process compared to higher-level languages. If you're working with a hash table, here’s how you would typically go about it:
- Accessing the Buckets: In a hash table, data is stored in an array of buckets, and each bucket can contain one or more key-value pairs. To iterate over the map, you start by looping through each bucket in the hash table.
- Handling Collisions: In cases where multiple key-value pairs get stored in the same bucket (due to hash collisions), you’ll need to handle this by navigating through each pair. Within each bucket, you may have to traverse a linked list to access all the key-value pairs stored there. This ensures that you visit and process every key-value pair in the hash table.
- Retrieving Key-Value Pairs: As you go through the hash table, you’ll retrieve and work with each key-value pair. This might include tasks like printing them, doing calculations, or even modifying the values as needed.
Why Is This Important?
Knowing how to iterate over a map is essential for C programmers since many applications depend on efficiently storing and retrieving data. Whether you're working on a simple project or a complex application, effectively managing key-value pairs will help your programs run more smoothly and make your code easier to maintain.
At The Programming Assignment Help, we’re dedicated to helping students grasp essential programming concepts. Learning how to implement and iterate over maps in C is a valuable skill that will improve your coding abilities and allow you to tackle various programming challenges. If you need help with C programming or any other assignments, explore our resources for expert support. Happy coding!