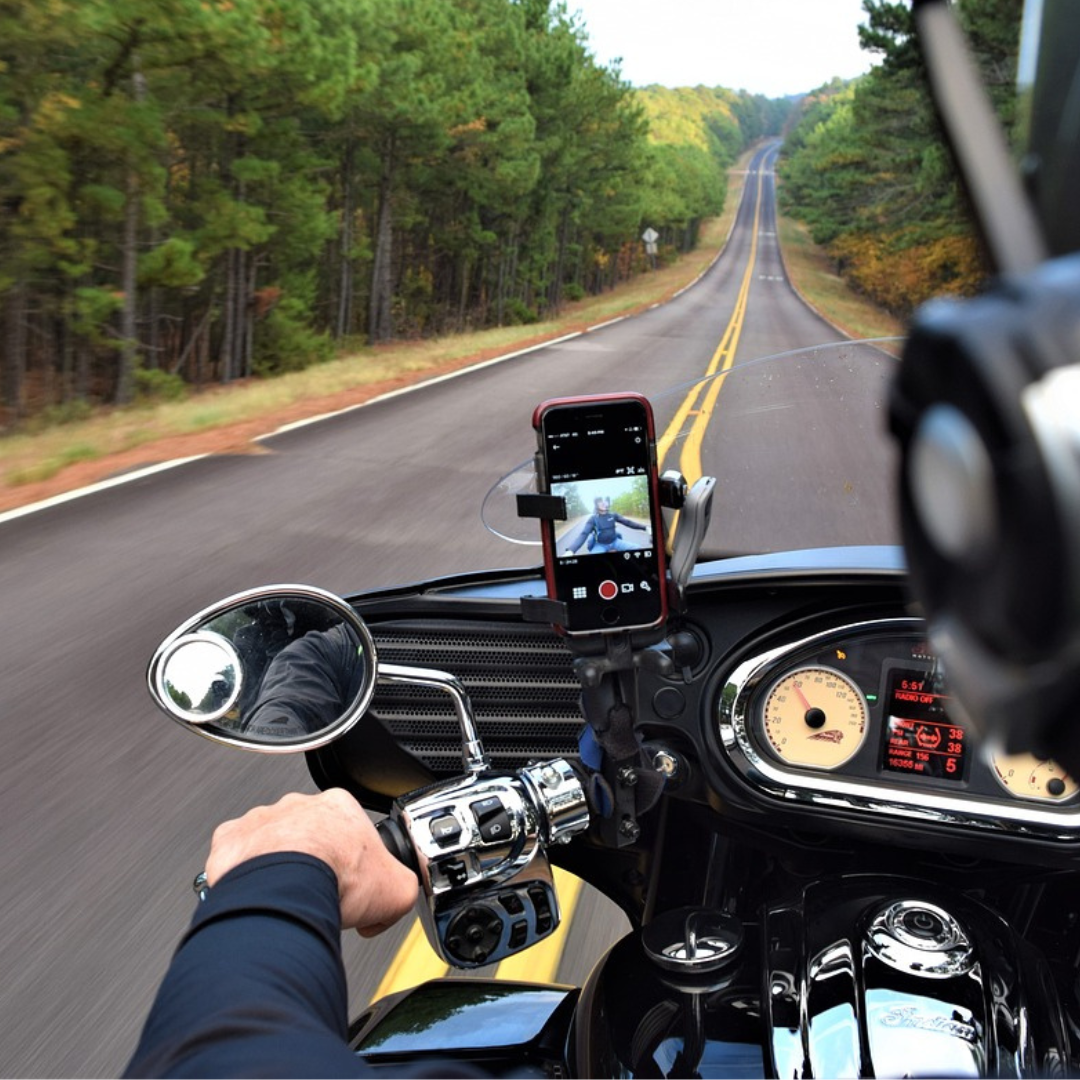
- 27th Mar 2023
- 08:06 am
- Admin
C++ Homework Question & Solution
C++ Distance Calculator (C++ Loops) - The distance a vehicle travels can be calculated as follows: distance = speed * time For example, if a train travels 40 miles per hour for 3 hours, the distance traveled is 120 miles. Write a program that asks the user for the speed of a vehicle and how many hours it has traveled. The program should then use a loop to display the distance the vehicle traveled for each hour of that time.
C++ Homework Solution
#include
using namespace std;
int main()
{
double speed, time, distance;
// ask the user for the speed and time
cout << "Enter the speed of the vehicle (in miles per hour): ";
cin >> speed;
cout << "Enter the time the vehicle traveled (in hours): ";
cin >> time;
// display the distance for each hour of travel
cout << "Hour\tDistance Traveled" << endl;
cout << "------------------------" << endl;
for (int i = 1; i <= time; i++)
{
distance = speed * i;
cout << i << "\t" << distance << " miles" << endl;
}
return 0;
}
In this solution, we first ask the user for the speed of the vehicle (in miles per hour) and the time the vehicle traveled (in hours). We then use a "for" loop to calculate and display the distance the vehicle traveled for each hour of that time. Note that we use a counter variable "i" to keep track of the hours, and the formula distance = speed * time to calculate the distance traveled for each hour. We use "\t" to separate the columns and "\n" to create new lines for better formatting.