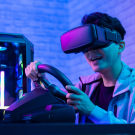
- 4th Aug 2023
- 17:20 pm
- Admin
#include
#include
using namespace std;
const int roadWidth = 10;
const int roadLength = 30;
const int carWidth = 3;
int carPosition = roadWidth / 2;
bool gameover = false;
void drawRoad()
{
for (int i = 0; i < roadWidth; i++)
{
for (int j = 0; j < roadLength; j++)
{
if (i == roadWidth / 2)
cout << "|"; // draw the road divider
else
cout << " ";
}
cout << endl;
}
}
void drawCar()
{
for (int i = 0; i < carWidth; i++)
{
cout << "*";
}
}
void updateGame()
{
if (_kbhit())
{
char ch = _getch();
if (ch == 'a' && carPosition > 1)
carPosition--;
else if (ch == 'd' && carPosition < roadWidth - carWidth - 1)
carPosition++;
else if (ch == 'q')
gameover = true;
}
}
void clearScreen()
{
system("cls");
}
int main()
{
while (!gameover)
{
drawRoad();
drawCar();
updateGame();
clearScreen();
}
cout << "Game Over!";
return 0;
}
This is a basic console-based car game where the player can control the car using 'a' (left) and 'd' (right) keys. The game will end when the player presses 'q'. The car is represented by three asterisks ('*'). The road is a simple two-lane road with a road divider in the middle.
Note: This code assumes that you are using a Windows system with the conio.h header for _kbhit() and _getch() functions. If you are using a different operating system, you may need to use alternative libraries for input handling.