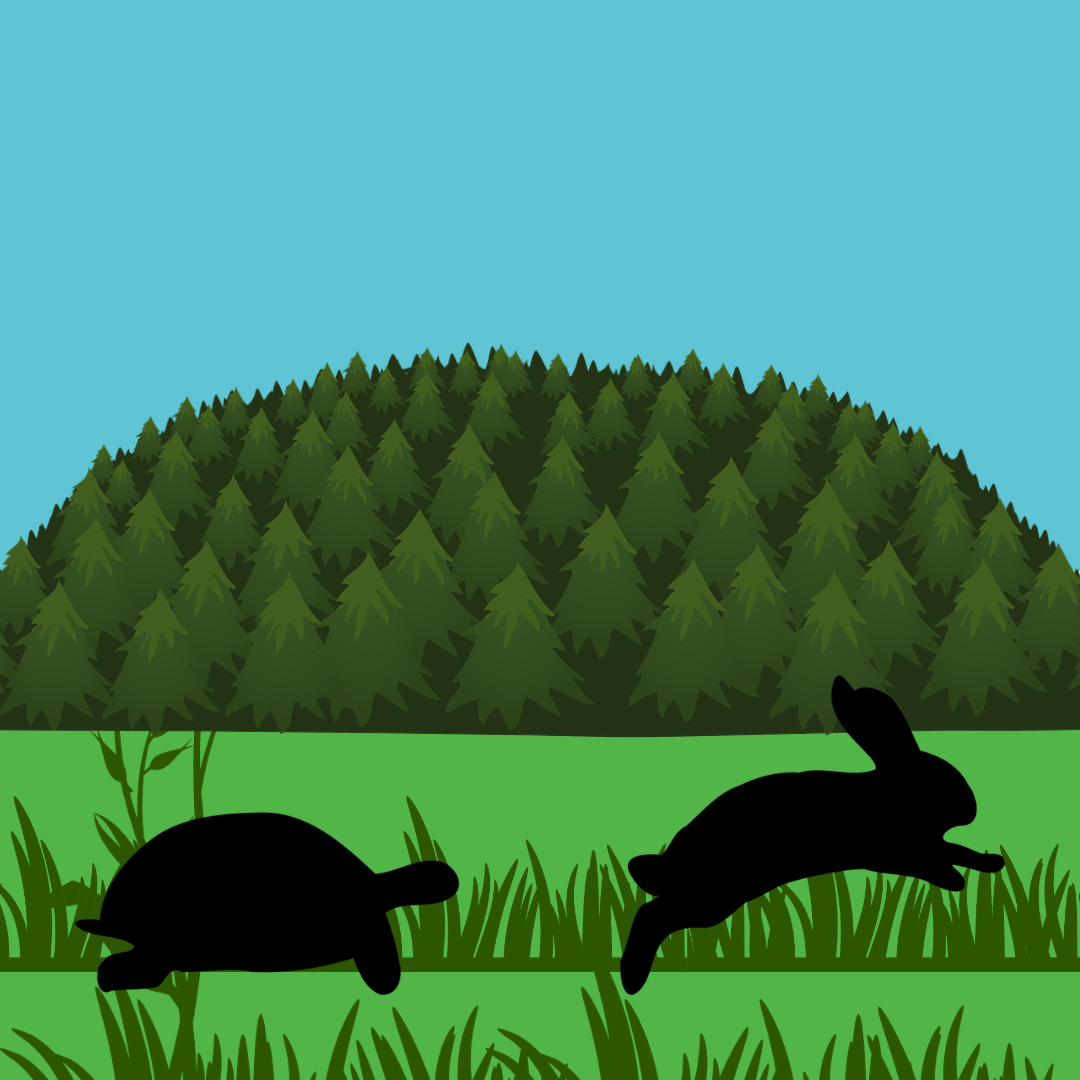
- 9th Apr 2023
- 05:44 am
- Admin
C# Homework Help
C# Homework Question
C# Logical Puzzles, Games, and Algorithms: Tortoise vs Hare
C# Homework Solution
using System;
class Program
{
static void Main(string[] args)
{
// Set up the race track
int finishLine = 100;
int tortoisePos = 1;
int harePos = 1;
Random rand = new Random();
// Main game loop
while (tortoisePos < finishLine && harePos < finishLine)
{
// Tortoise moves
int tortoiseMove = rand.Next(1, 11);
if (tortoiseMove >= 1 && tortoiseMove <= 5)
{
tortoisePos += 3;
}
else if (tortoiseMove >= 6 && tortoiseMove <= 7)
{
tortoisePos -= 6;
}
else
{
tortoisePos += 1;
}
// Hare moves
int hareMove = rand.Next(1, 11);
if (hareMove >= 1 && hareMove <= 2)
{
harePos += 0;
}
else if (hareMove >= 3 && hareMove <= 4)
{
harePos += 9;
}
else if (hareMove == 5)
{
harePos -= 12;
}
else if (hareMove >= 6 && hareMove <= 8)
{
harePos += 1;
}
else
{
harePos -= 2;
}
// Display current positions
Console.WriteLine("Tortoise position: " + tortoisePos);
Console.WriteLine("Hare position: " + harePos);
}
// Determine the winner
if (tortoisePos >= finishLine)
{
Console.WriteLine("Tortoise wins!");
}
else
{
Console.WriteLine("Hare wins!");
}
Console.ReadLine();
}
}
This program sets up a race track with a finish line at position 100, and then simulates the Tortoise vs Hare race using a while loop. The tortoise and hare move randomly based on probabilities defined by the Tortoise vs Hare algorithm. After each move, the program displays the current positions of the tortoise and hare. Once one of them crosses the finish line, the program determines the winner and displays the result.