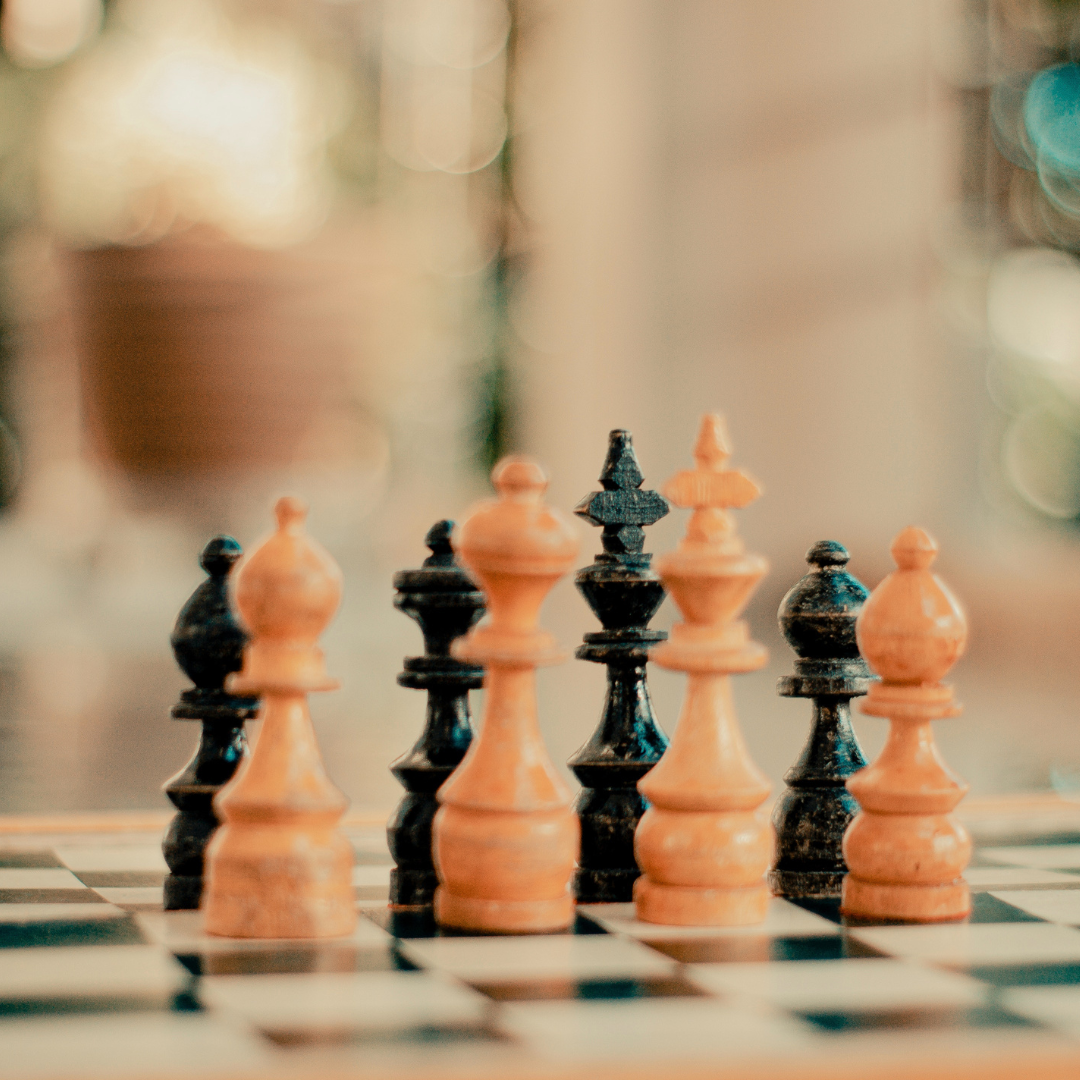
- 9th Apr 2023
- 05:58 am
Get the best C# Homework Help from our qualified programming experts
C# Homework Question ; C# Logical Puzzles, Games, and Algorithms: 8 queens problem
C# Homework Solution:
using System;
class EightQueensProblem
{
static int boardSize = 8;
static int[,] board = new int[boardSize, boardSize];
static bool foundSolution = false;
static void Main()
{
PlaceQueens(0);
if (!foundSolution)
{
Console.WriteLine("No solution found.");
}
}
static void PlaceQueens(int row)
{
if (row == boardSize)
{
PrintBoard();
foundSolution = true;
}
else
{
for (int col = 0; col < boardSize; col++)
{
if (IsSafe(row, col))
{
board[row, col] = 1;
PlaceQueens(row + 1);
board[row, col] = 0;
}
}
}
}
static bool IsSafe(int row, int col)
{
for (int i = 0; i < row; i++)
{
if (board[i, col] == 1)
{
return false;
}
}
for (int i = row, j = col; i >= 0 && j >= 0; i--, j--)
{
if (board[i, j] == 1)
{
return false;
}
}
for (int i = row, j = col; i >= 0 && j < boardSize; i--, j++)
{
if (board[i, j] == 1)
{
return false;
}
}
return true;
}
static void PrintBoard()
{
Console.WriteLine("Solution:");
for (int i = 0; i < boardSize; i++)
{
for (int j = 0; j < boardSize; j++)
{
Console.Write(board[i, j] + " ");
}
Console.WriteLine();
}
Console.WriteLine();
}
}
This program finds a solution to the 8 queens problem by placing queens on a chessboard such that no two queens attack each other. It does this using a recursive algorithm that tries to place a queen on each row, starting from the top row, and backtracking when a safe position cannot be found. The program prints out the first solution it finds, or a message indicating that no solution exists.