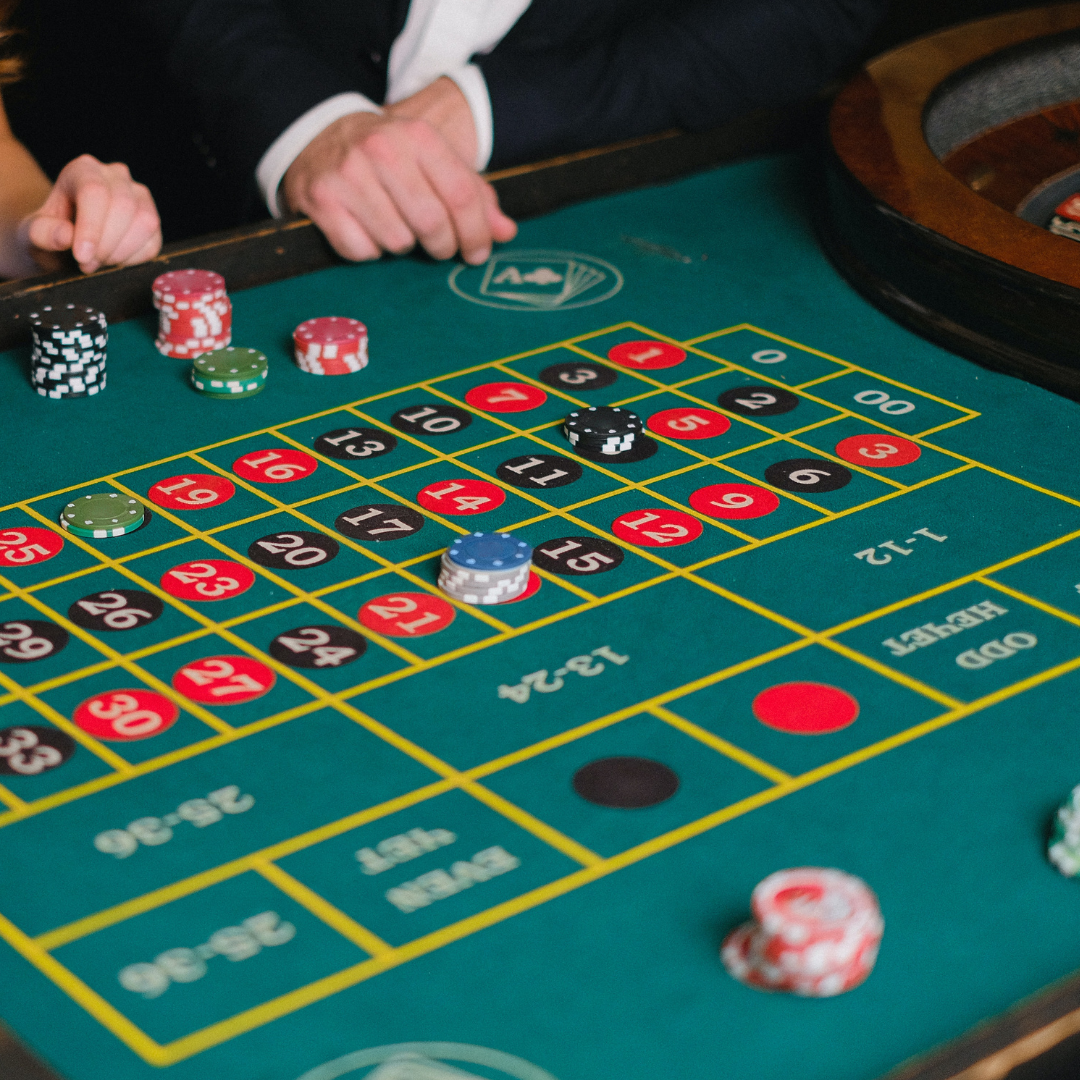
- 7th Apr 2023
- 09:30 am
C# Homework Question - C# Logical Puzzles, Games, and Algorithms: Game of Craps
C# Homework Solution
using System;
class CrapsGame
{
static void Main()
{
Random rand = new Random();
int dice1, dice2, sum, point;
bool win = false;
Console.WriteLine("Welcome to the game of craps!");
Console.WriteLine("Press enter to roll the dice.");
Console.ReadLine();
dice1 = rand.Next(1, 7);
dice2 = rand.Next(1, 7);
sum = dice1 + dice2;
Console.WriteLine("You rolled {0} and {1} for a total of {2}.", dice1, dice2, sum);
if (sum == 7 || sum == 11)
{
Console.WriteLine("You win!");
win = true;
}
else if (sum == 2 || sum == 3 || sum == 12)
{
Console.WriteLine("You lose!");
}
else
{
point = sum;
Console.WriteLine("Your point is {0}.", point);
while (!win)
{
Console.WriteLine("Press enter to roll the dice.");
Console.ReadLine();
dice1 = rand.Next(1, 7);
dice2 = rand.Next(1, 7);
sum = dice1 + dice2;
Console.WriteLine("You rolled {0} and {1} for a total of {2}.", dice1, dice2, sum);
if (sum == 7)
{
Console.WriteLine("You lose!");
break;
}
else if (sum == point)
{
Console.WriteLine("You win!");
break;
}
}
}
}
}
C# Program Explained by Our Programming Homework Helper
- The CrapsGame class contains the Main method, which is the entry point of the program.
- A Random object is created to generate random numbers for the dice rolls.
- The program starts by rolling the dice and calculating the sum of the two dice.
- If the sum is 7 or 11, the player wins. If the sum is 2, 3, or 12, the player loses. Otherwise, the sum becomes the "point" and the player keeps rolling until they either roll a 7 (in which case they lose) or they roll the point again (in which case they win).
- The program uses a while loop to keep rolling until the player wins or loses.
- The break statement is used to exit the loop once the game is over.