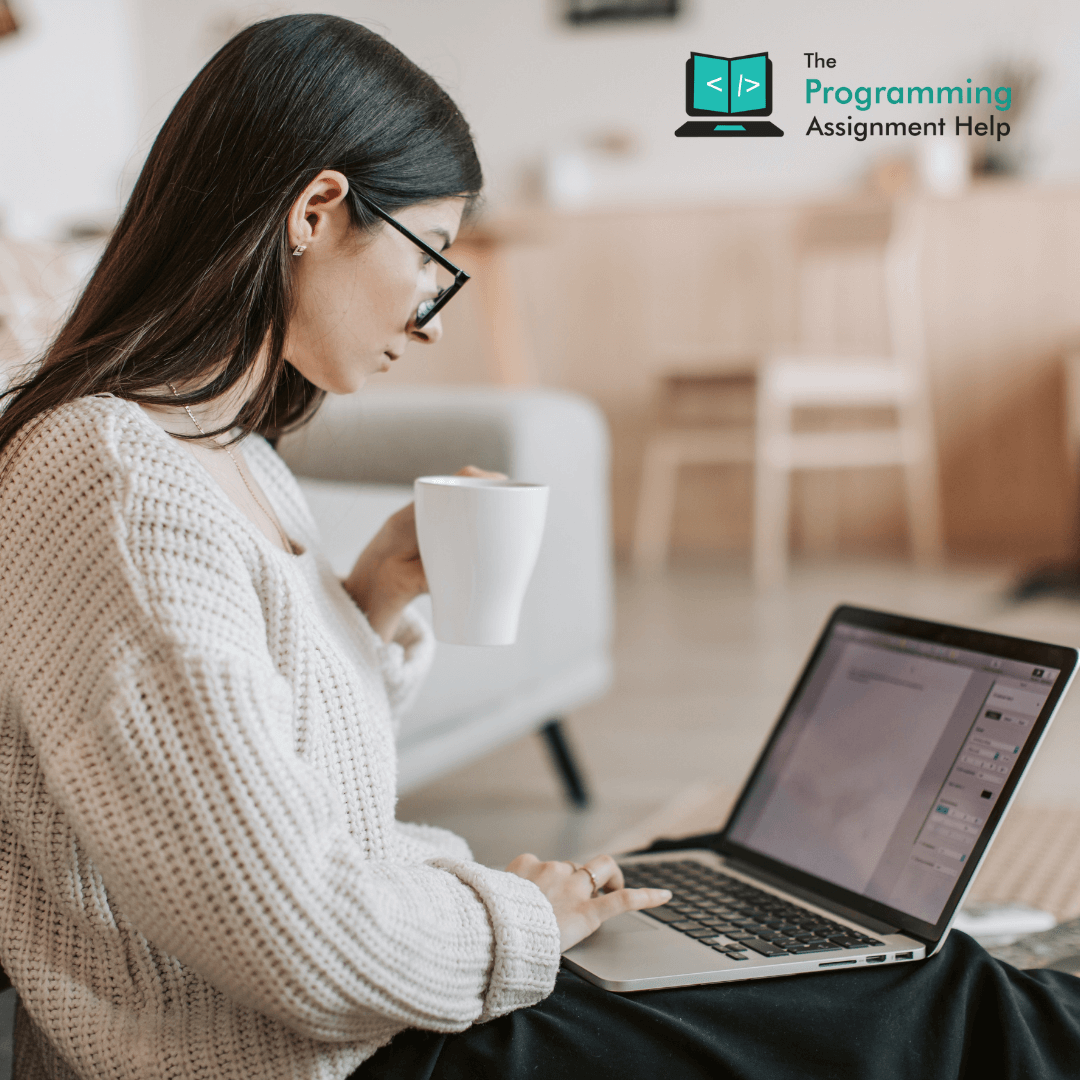
- 19th Oct 2021
- 03:06 am
- Adan Salman
Main Code:
#include #include #include #include "LinkedList.h" using namespace std; string removeSpace(string str) { int count = 0; for (int i=0; i if (str[i] == ' '){ count++; } else { break; } } return str.substr(count,str.length()-count); } string upperCase(string str) { for (int i=0; i str[i] = toupper(str[i]); } return str; } //void createAndInsert(); int main() { int size = 50; LinkedList library[size]; // Library of branch size = 50 int choice = 0; while (choice != 9) { // Display MENU cout << "\n1. Create a branch and insert its books.\n"; cout << "2. Give an author name (first, last) and a branch name, to CHECKOUT that book from the branch.\n"; cout << "3. Give an author name (first, last), a title and a branch name, to RETURN that book to the branch.\n"; cout << "4. Give an author name (first, last), a title and a branch name, to FIND the number of copies of that book that are available in that branch.\n"; cout << "5. Give a branch name, to PRINT the author name (first, last) and title of all books contained in that branch.\n"; cout << "6. Give a branch name and an author name, to FIND the number of books by that author contained in the branch.\n"; cout << "7. Give an author name (first, last), to FIND the number of books by that author contained in all the branches of the library system.\n"; cout << "8. Give an author name (first, last), to PRINT in lexicographical order the titles of all books by that author contained in all the branches of the library system.\n"; cout << "9. Exit the program.\n"; cout << "\nWhat is your menu choice?\n"; cin >> choice; switch(choice) { case 1: { string branch_name; string first_name, last_name, book_title; cout << "\nWhat is the name of the branch?" << endl; cin >> branch_name; branch_name = upperCase(branch_name); int found = -1; for(int i=0; i // if library does not contains user enetered branch name // set the branch name and save the location of the branch if (library[i].get_branch_name().empty()){ found = i; library[i].set_branch_name(branch_name); break; } else { // if library contains user entered branch name // save the loation of branch name if (branch_name == library[i].get_branch_name()){ found = i; break; } } } int cond = 1; // prompt and add author and book title till user enter NONE NONE NONE // breaking do while loop condition , cond = 0 do { cout << "\nWhat is author and title of book?" << endl; cin >> first_name >> last_name; getline(cin, book_title); book_title = removeSpace(book_title); first_name = upperCase(first_name); last_name = upperCase(last_name); book_title = upperCase(book_title); string authorName = first_name + " " + last_name; if (!(first_name == "NONE" && last_name == "NONE" && book_title == "NONE")){ library[found].insert(authorName,book_title,1); } else { cond = 0; // loop breaking variable } } while(cond != 0); cout << "\nThank you, I created branch " << branch_name << endl; break; } case 2: { string first_name, last_name, data, book_title, branch_name; cout << "\nGive author, title and branch from which to checkout the book:\n"; cin >> first_name >> last_name; getline(cin, data); data = removeSpace(data); int pos = 0; // loop to extract book title and branch name by finding the position of last space in the string for (int i=data.length()-1; i>0; i--){ if (data[i] == ' '){ pos = i+1; break; } } branch_name = data.substr(pos,data.length()-pos); branch_name = upperCase(branch_name); book_title = data.substr(0,pos-1); book_title = upperCase(book_title); pos = -1; for (int i=0; i if (library[i].get_branch_name().empty()){ break; } else { if (library[i].get_branch_name() == branch_name){ pos = i; break; } } } if (pos != -1){ string authorName = upperCase(first_name) + " " + upperCase(last_name); int found = library[pos].checkout(authorName,book_title); if (found == 0){ cout << "\nSorry! This book is not available at branch " << branch_name << endl; } else { cout << "\nThank you! The book " << book_title << " by " << authorName << " has been checked out from " << branch_name << endl; } } else { cout << "\nSorry! There is no branch " << branch_name << endl; } break; } case 3: { string first_name, last_name, data, branch_name, book_title; cout << "\nGive author, title and branch to which book is to be returned:\n"; cin >> first_name >> last_name; getline(cin, data); data = removeSpace(data); int pos = 0; // loop to extract book title and branch name by finding the position of last space in the string for (int i=data.length()-1; i>0; i--){ if (data[i] == ' '){ pos = i+1; break; } } branch_name = data.substr(pos,data.length()-pos); branch_name = upperCase(branch_name); book_title = data.substr(0,pos-1); book_title = upperCase(book_title); pos = -1; for (int i=0; i if (library[i].get_branch_name().empty()){ break; } else { if (library[i].get_branch_name() == branch_name){ pos = i; break; } } } string authorName = upperCase(first_name) + " " + upperCase(last_name); if (pos != -1){ library[pos].insert(authorName,book_title,1); cout << "\nThank you! The book " << book_title << " by " << authorName << " has been returned to branch " << branch_name << endl; } else { cout << "\nSorry! There is no branch " << branch_name << endl; } break; } case 4: { string first_name, last_name, data, branch_name, book_title; cout << "\nGive an author name (first, last), a title and a branch name, to FIND the number of copies of that book that are available in that branch (returns zero if there are none).\n"; cin >> first_name >> last_name; getline(cin, data); data = removeSpace(data); int pos = 0; // loop to extract book title and branch name by finding the position of last space in the string for (int i=data.length()-1; i>0; i--){ if (data[i] == ' '){ pos = i+1; break; } } branch_name = data.substr(pos,data.length()-pos); branch_name = upperCase(branch_name); book_title = data.substr(0,pos-1); book_title = upperCase(book_title); pos = -1; for (int i=0; i if (library[i].get_branch_name().empty()){ break; } else { if (library[i].get_branch_name() == branch_name){ pos = i; break; } } } string authorName = upperCase(first_name) + " " + upperCase(last_name); if (pos != -1){ int no_copies = library[pos].get_num_copies(authorName,book_title); cout << "\nThere are " << no_copies << " books in the library branch " << branch_name << " by " << authorName << " titled " << book_title << endl; } else { cout << "\nSorry! There is no branch " << branch_name << endl; } break; } case 5: { string branch_name; cout << "\nGive a branch name, to PRINT the author name (first, last) and title of all books contained in that branch.\n"; cin >> branch_name; branch_name = upperCase(branch_name); int pos = -1; for (int i=0; i if (library[i].get_branch_name().empty()){ break; } else { if (library[i].get_branch_name() == branch_name){ pos = i; break; } } } if (pos != -1){ cout << "\nThe books in " << branch_name << " are:\n"; library[pos].print(); } else { cout << "\nSorry! There is no branch " << branch_name << endl; } break; } case 6: { string first_name, last_name, branch_name; cout << "\nGive a branch name and author name to print number of books.\n"; cin >> branch_name >> first_name >> last_name; branch_name = upperCase(branch_name); int pos = -1; for (int i=0; i if (library[i].get_branch_name().empty()){ break; } else { if (library[i].get_branch_name() == branch_name){ pos = i; break; } } } string authorName = upperCase(first_name) + " " + upperCase(last_name); if (pos != -1){ int no_books = library[pos].get_num_books(authorName); cout << "\nThe number of books by " << authorName << " is " << no_books << endl; } else { cout << "\nSorry! There is no branch " << branch_name << endl; } break; } case 7: { string first_name, last_name; cout << "\nGive an author name (first, last), to FIND the number of books by that author contained in all the branches of the library system.\n"; cin >> first_name >> last_name; string authorName = upperCase(first_name) + " " + upperCase(last_name); int i=0, result = 0; while (!(library[i].get_branch_name().empty())) { result += library[i].get_num_books(authorName); i++; } cout << "\nThere are " << result << " books in the library system by " << authorName << endl; break; } case 8: { string first_name, last_name; cout << "\nGive an author name (first, last), to FIND title of books by that author contained in all the branches of the library system.\n"; cin >> first_name >> last_name; string authorName = upperCase(first_name) + " " + upperCase(last_name); LinkedList tempNode[1]; // to store the book title in lexicographically order int i=0; while(!(library[i].get_branch_name().empty())) { book *bk = library[i].get_title_info(authorName); // gets all the book information corresponding to library at given branch //insert all the book information corresonding to the author to the tempNode while(bk != NULL) { tempNode[0].insert(bk->author_name, bk->book_title, bk->num_copies); bk = bk->next; } i++; } cout << "\nThe books by " << authorName << " are:\n"; tempNode[0].print_title(); break; } case 9: { cout << "\nThank you and goodbye.\n\n"; break; } } } return 0; } *******************************************
Linked List:
#include using namespace std; struct book { string author_name; string book_title; int num_copies; book *next; }; class LinkedList { private: string branch_name; book *head; public: LinkedList(); void set_branch_name(string bname); string get_branch_name(); void insert(string authorName, string bookTitle, int noc); int checkout(string authorName, string bookTitle); int get_num_copies(string authorName, string bookTitle); int get_num_books(string authorName); book* get_title_info(string authorName); void print_title(); void print(); }
The main code is menu-driven and offers several options to interact with the library system, such as creating a branch, inserting books, checking out books, returning books, finding the number of copies of a book, printing books in a branch, finding the number of books by an author in a branch, finding the number of books by an author in the entire library system, and printing book titles by an author in lexicographical order.
The linked list class (LinkedList
) appears to have the following member functions:
LinkedList()
: Constructor to initialize a linked list.set_branch_name(string bname)
: Sets the name of the branch.get_branch_name()
: Returns the name of the branch.insert(string authorName, string bookTitle, int noc)
: Inserts a new book into the linked list with the given author name, book title, and number of copies.checkout(string authorName, string bookTitle)
: Handles the book checkout process by reducing the number of available copies for a specific book.get_num_copies(string authorName, string bookTitle)
: Returns the number of available copies of a specific book in the branch.get_num_books(string authorName)
: Returns the total number of books by a specific author in the branch.get_title_info(string authorName)
: Returns a linked list containing all book information (titles and number of copies) by a specific author in the branch.print_title()
: Prints the titles of all books in the branch.print()
: Prints the author names and titles of all books in the branch.
To understand the entire functionality and logic of the program, you would need to analyze both the LinkedList
class and the main()
function together. The program appears to be designed for managing a library system with multiple branches and books.
If you have any specific questions or need further assistance with understanding or modifying the code, feel free to ask!