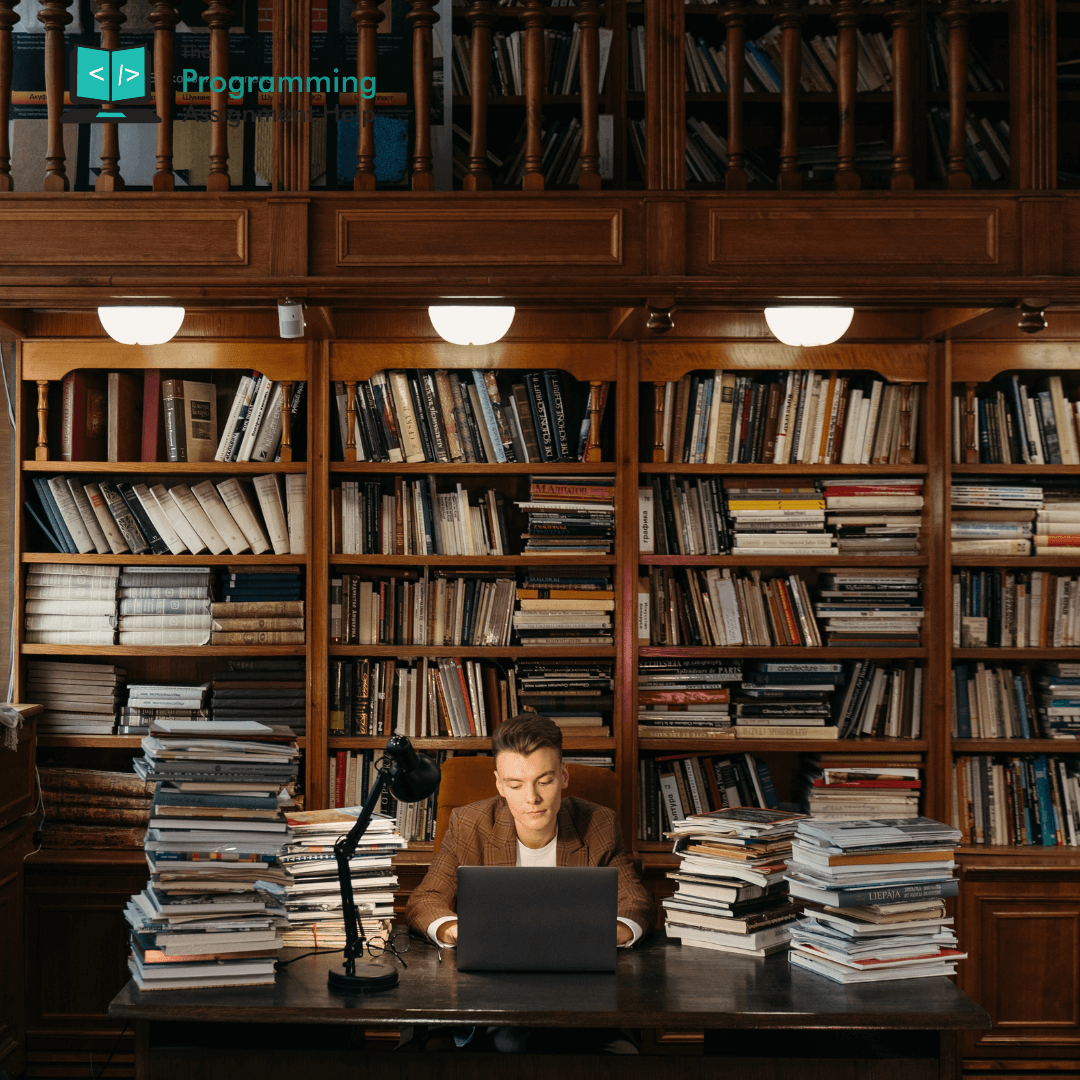
- 30th Jun 2022
- 02:41 am
- Admin
The provided C code is a simple password verification program with a limited number of attempts. It also includes a function important
that takes a string (C-style string) input and copies it into a local buffer buf
. Additionally, there is a function last
that prints "Root access".
C Programming Homework Solution - Written By Student
#include
#include
void last()
{
printf("\nRoot access");
}
void important(const char* input)
{
char buf[10];
strcpy(buf, input);
}
int main()
{
printf("Address of last = %p\n", last);
char systemPassword[8]="12345";
char userPassword[8];
int access=0;
int count=0;
while(count<4)
{
if(count==3)
{
printf("\nSystem Locked");
break;
}
if(count>0)
{
printf("\nYou have %d attemp left",3-count);
}
printf("\nEnter Password : ");
scanf("%s",userPassword);
if(strcmp(systemPassword,userPassword)==0)
{
access++;
}
else
{
printf("\nWrong Credentials");
}
if(access)
{
char *dob;
printf("\nEnter Date of birth for verification : ");
scanf("%s",dob);
important(dob);
}
count++;
}
return 0;
}
C Programming Tutoring - Comments By Our Programming Tutors on the Code
The code has several issues and potential vulnerabilities:
- The userPassword and systemPassword arrays are not properly secured against buffer overflows. A fixed-size buffer can lead to a buffer overflow if the user enters more than the allocated size of characters.
- The dob pointer is uninitialized, and the
important
function uses it without proper memory allocation, causing undefined behavior and possibly crashing the program. - The systemPassword is hardcoded, which is not a secure way to handle passwords.
- The
strcpy
function used in theimportant
function should be avoided, especially when copying data from untrusted sources.
This code should not be used as a real-world password verification mechanism due to its security vulnerabilities. For actual password verification, consider using secure password-handling libraries or encryption mechanisms.
If you need assistance with writing secure password verification code or have any other questions, feel free to ask!