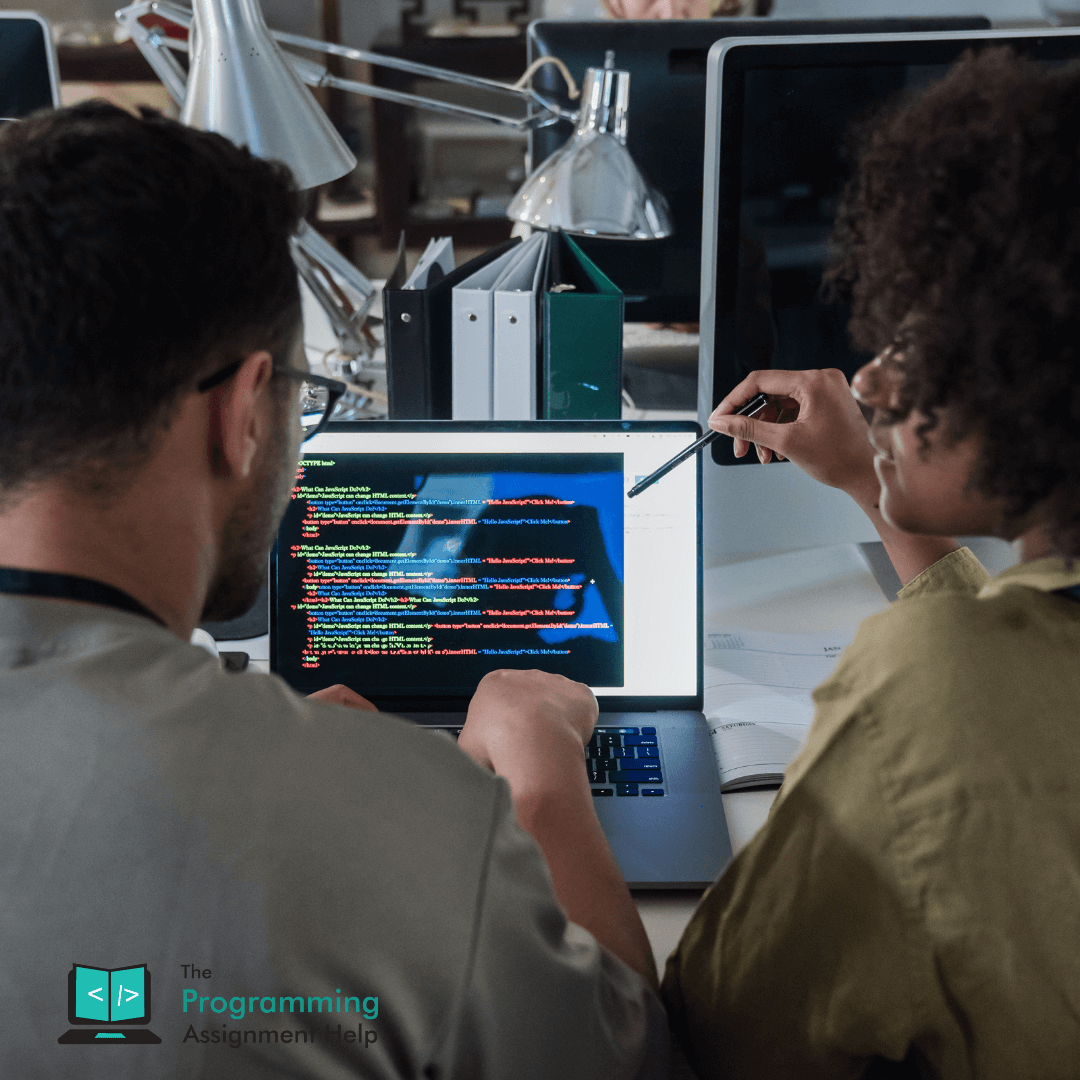
- 18th Aug 2022
- 03:37 am
The provided C code is a program that takes user input in the form of boolean values (TRUE or FALSE) and performs various boolean calculations based on the user's input. The user can enter boolean expressions using TRUE, FALSE, and logical operators such as AND (&), OR (|), and NOT (!).
Here's a brief explanation of how the program works:
-
The program asks the user to input boolean values and boolean expressions in a specific format.
-
The user is expected to enter boolean values as TRUE or FALSE, and boolean expressions using the operators & (AND), | (OR), and ' (NOT). The format is as follows:
a. To input
TRUE AND FALSE
, the user should enter:TRUE FALSE &
b. To inputTRUE OR FALSE
, the user should enter:TRUE FALSE |
c. To inputNOT TRUE
, the user should enter:TRUE'
-
The program parses the user's input to extract individual characters representing the boolean values and operators.
-
The characters representing boolean values (T/t for TRUE and F/f for FALSE) are converted into integer values (1 for TRUE and 0 for FALSE) for condition checks.
-
The program then evaluates the boolean expressions according to the input and prints the output, which can be either
true
orfalse
. -
The program accounts for different cases such as negation (
!
), AND (&
), and OR (|
) to calculate the final boolean value.
Program
#include
#include
int main()
{
printf("\nplease input data in below format........\n");
printf("\nCalculate any type true and false boolean calculation in given bellow --------->\n");
printf("\n1.(TRUE & FALSE) -->Input in --> TRUE FALSE & --->format \n2.(TRUE | FALSE) -->Input in --> TRUE FALSE | --->format\n3.(TRUE !) -->Input in --> TRUE' --->format\n4.( NOT(TRUE AND FALSE) OR FALSE ) -->Input in --> TRUE FALSE & ' FALSE |--->format \n");
char str1[50];
char newString[10][10];
int i,j,ctr;
printf("---------------------------------------\n");
printf(" Please input values : ");
fgets(str1, sizeof str1, stdin);
j=0; ctr=0;
for(i=0;i<=(strlen(str1));i++)
{
// if space or NULL found, assign NULL into newString[ctr]
if(str1[i]==' '||str1[i]=='\0')
{
newString[ctr][j]='\0';
ctr++; //for next word
j=0; //for next word, init index to 0
}
else
{
newString[ctr][j]=str1[i];
j++;
}
}
char a,b,c,d,e,f;
int a1,b1,c1,d1,e1,f1;
for(i=0;i {
for(j=0;j<1;j++)
{
if(i==0){
a=newString[i][j];}
if(i==1){
b=newString[i][j];}
if(i==2){
c=newString[i][j];}
if(i==3){
d=newString[i][j];}
if(i==3){
e=newString[i][j];}
if(i==3){
f=newString[i][j];}
}
}
// make integer from character value for condition checks....
if((a=='t') | (a=='T')){
a1=1;
}
else{
a1=0;
}
if((b=='t') | (b=='T')){
b1=1;
}
else{
b1=0;
}
if((c=='t') | (c=='T')){
c1=1;
}
else{
c1=0;
}
if((d=='t') | (d=='T')){
d1=1;
}
else{
d1=0;
}
if((e=='t') | (e=='T')){
e1=1;
}
else{
e1=0;
}
if((f=='t') | (f=='T')){
f1=1;
}
else{
f1=0;
}
// checks the conditions---------
// !(true & false) | true calculation....................
if(d=='!') {
if ( !(a1 & b1) | e1 ){
printf("Output is-->");
printf("true");
}
else{
printf("Output is-->");
printf("false");
}
}
//true | false condition check...........................
else if(c=='|'){
if ( a1 | b1 ){
printf("Output is-->");
printf("true");
}
else{
printf("Output is-->");
printf("false");
}
}
// Not ! true or false calculation.......................
else if( b=='!'){
if ( !a1 ){
printf("Output is-->");
printf("true");
}
else{
printf("Output is-->");
printf("false");
}
}
//
// (true & false ) condition check........................
else if(c=='&'){
if ( a1 & b1 ){
printf("Output is-->");
printf("true");
}
else{
printf("Output is-->");
printf("false");
}
}
else{
printf("please enter input in given right format in given choice :....");
}
return 0;
}
Please let me know if you have any specific questions or need further clarification on any part of the code! Talk to your C programming Assignment Help Experts.