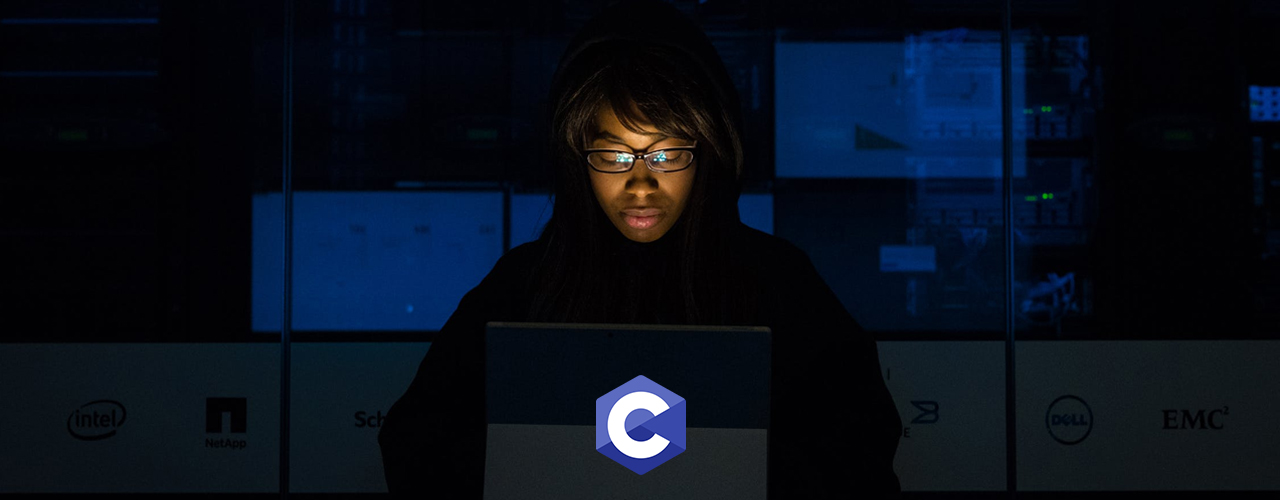
- 27th Feb 2019
- 01:00 am
C Program Assignment Help - Write a Program to solve a challenge in timber management
Timber Regrowth :
A challenge in timber management is to determine how many acres to leave uncut after harvesting in a forest so that the harvested area is reforested in a certain period of time. It is assumed that reforestation take place at a known constant rate, r¸ per year, depending on climate and soil conditions. A reforestation equation expresses this growth as a function of the amount of timber standing and the reforestation rate. i 1 i i A ArA + = + where i represents the year, 0 after harvesting is completed, and 1, 2, 3 represents the number of years after harvesting (thus A0 represents the acres uncut after harvesting is completed, and A1, A2, A3, …represents the reforested acres after years 1, 2, 3, …). r is the reforestation rate expressed as a fraction (for example 0.05 means that the forested acres are increases by 5% at the end of a year). You are responsible for developing a software tool that supports analysis of reforestation. Given (from the user)
- The number of total acres,
- A minimum value for acres uncut,
- A maximum value for acres uncut,
- Reforestation rate,
Show a table in the console that increments the values of uncut acres (from the given minimum to the maximum values) and for each of these values the total number of years it takes to totally restore the forest. The table shall contain 20 rows. The following shows a sample interaction with the user.
C Program :
#include < stdio.h > #include < math.h > struct FOREST { char name[20]; double acres; double min; double max; double rate; }; intcalculateTableData(double area, doubler, doublemaxarea) { int p = 0; int s = 0; doublear = area; while (s == 0) { if (ar >= maxarea) { s = 1; } else { ar = ar * (1 + r); p = p + 1; } } return p; } voidgetForestInput(char * n[], double * t, double * mi, double * ma, double * ra) { int i = 0; printf("Forest name : "); scanf(" %[^\n]s", & * n); printf("\n"); while (i == 0) { printf("Total acres : "); scanf("%lf", & * t); printf("\n"); if ( * t <= 0) { printf("Please enter a positive value!!\n"); } else { i = 1; } } i = 0; while (i == 0) { printf("Acres Uncut Minimum :"); scanf("%lf", & * mi); printf("\n"); if ( * mi <= 0) { printf("Please enter a positive value!!\n"); } else if ( * t < * mi) { printf("Minimum area should be less than total area!!\n"); } else { i = 1; } } i = 0; while (i == 0) { printf("Acres Uncut Maximum :"); scanf("%lf", & * ma); printf("\n"); if ( * ma <= 0) { printf("Please enter a positive value!!\n"); } else if ( * mi > * ma) { printf("Maximum area should be more than minimum!!\n"); } else if ( * t < * ma) { printf("Maximum area should be less than total area!!\n"); } else { i = 1; } } i = 0; while (i == 0) { printf("Reforestation rate :"); scanf("%lf", & * ra); printf("\n"); if ( * ra < 0) { printf("Please enter a positive value!!\n"); } else if ( * ra == 0 || * ra >= 1) { printf("Please enter a value between 0.0 and 1.0!!\n"); } else { i = 1; } } } voiddisplayTable(struct FOREST f) { printf("Forest : %s\n Uncut Area : min = %.2f, max = %.2f\n Reforestation Rate %.2f\n", f.name, f.min, f.max, f.rate); doubleincr = (f.max - f.min) / 19; int k = 0; printf("Uncut Area Start Num Years For Total Reforestation\n"); while (k == 0) { if (f.max - f.min > 0.001) { printf("%10.2f %30d\n", f.min, calculateTableData(f.min, f.rate, f.acres)); f.min = f.min + incr; } else { printf("%10.2f %30d\n", f.max, calculateTableData(f.max, f.rate, f.acres)); k = 1; } } } int main() { int i = 0; char opt = 'n'; int p = 0; struct FOREST f; while (i == 0) { if (opt == 'y') { i = 1; } else { getForestInput( & f.name, & f.acres, & f.min, & f.max, & f.rate); displayTable(f); printf("Do you want to quit?(y/n)"); scanf(" %c", & opt); } } return 0; }
If you want to learn C programming, then reach out to our experts.
About the Author - Seddy
With a fervent dedication to decoding complex datasets and crafting innovative solutions, I am a seasoned programmer proficient in Statistical Data Analysis, Statistics, Statistical Modeling, and programming. My expertise lies at the intersection of technical acumen and analytical prowess, allowing me to engineer dynamic solutions that empower organizations to make data-driven decisions.