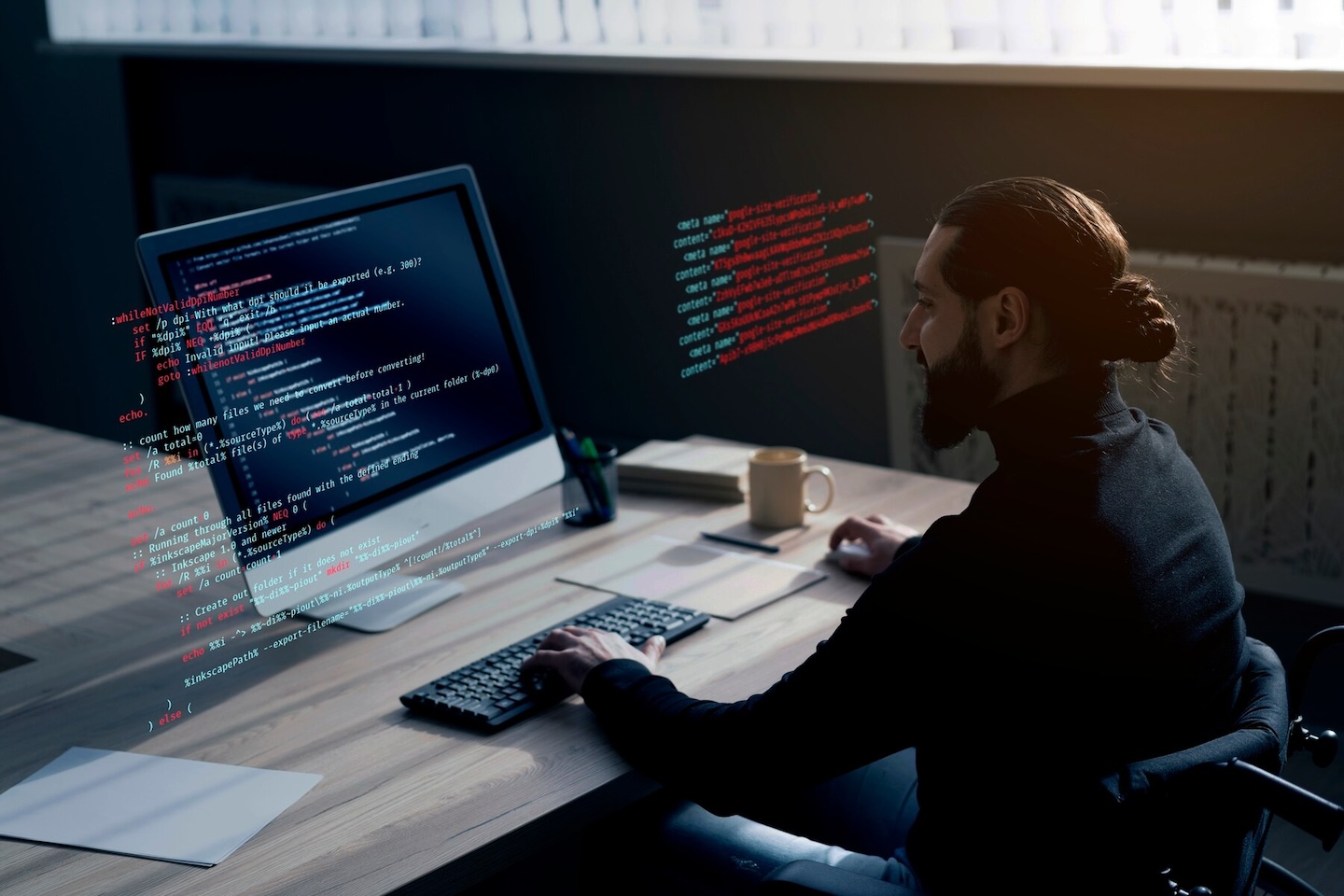
- 13th Dec 2023
- 00:33 am
- Admin
Virtual functions are required in C++ to provide polymorphism, a fundamental concept in object-oriented programming. A virtual function is a base class member function that has the virtual keyword attached to it. It allows derived classes to have their implementation, allowing for runtime dynamic or late binding. In a class, a virtual function creates a dynamic link between the function call and the function definition. This means that the correct version of the function is determined at runtime based on the object's actual type rather than the type of the object's pointer or reference. This feature is critical for creating runtime polymorphism, which enables more flexible and extendable programming.
Derived classes that override a virtual function give their own specialised implementation while abiding to the substitutability principle. This allows a programme to interact with objects from multiple derived classes over a common interface, promoting code reuse and modularity.
- Declaration in Base Class: Using the 'virtual' keyword, virtual functions are declared in the base class. This tells the compiler that the function is overridable in derived classes.
- Overriding in Derived Classes: The virtual function in derived classes can be overridden with the 'override' keyword. This assures that the derived class's function has the same signature as the base class's virtual function.
- Dynamic Binding: Dynamic binding is the most important property of virtual functions. When a virtual function is invoked using a base class pointer or reference, the actual function to be performed is selected at runtime based on the kind of object pointed to by the pointer or reference.
- Late Binding: Dynamic binding is sometimes known as late binding since the decision to invoke a function is decided during programme execution rather than at compile time.
- Default Implementation: A virtual function in the base class might have a default implementation. If a derived class does not override the virtual function, it will inherit the base class's implementation.
The usage of virtual functions enables polymorphism to be implemented, allowing a program to interact with objects of different derived classes via a common interface (the base class). It is a key idea in C++ object-oriented programming for enabling runtime polymorphism.
C++ Virtual Function Applications
- Polymorphism:
The fundamental and most important application of virtual functions is polymorphism. Polymorphism allows objects from various derived classes to be considered as objects from the same base class, resulting in a unified interface. Because a base class pointer can be used to communicate with objects of many derived classes, this encourages flexibility and simplifies programming.
- Dynamic Binding:
Virtual functions enable dynamic binding, allowing the actual function to be called at runtime to be determined based on the type of the object. In contrast, in static binding, the decision is taken at compile time. Dynamic binding is critical for object-oriented programmes to achieve runtime flexibility.
- Function Overriding:
Virtual functions support function overriding, allowing derived classes to provide their own implementations of the base class's functions. This is required for behaviour customisation and specialisation in derived classes while retaining a common interface.
- Abstract Classes and Interfaces:
Abstract classes that serve as interfaces are created using virtual functions. Pure virtual functions in abstract classes define a set of functions that must be implemented by any class derived from them. This makes it mandatory for derived classes to perform certain functionality.
- Default Implementations:
Default implementations are allowed for virtual functions in the base class. If a derived class does not override a virtual function, it inherits the base class's default implementation. This enables for a standardised behaviour that may be tailored as needed.
Advantages of Virtual Function
Virtual functions in C++ provide various benefits that help to increase the flexibility and extensibility of object-oriented code. Here are five significant benefits:
- Polymorphism: Polymorphism is enabled by virtual functions, which allow objects of various derived classes to be considered as objects of the shared base class. By providing a single interface for interacting with objects of various types, this enhances code reusability and flexibility.
- Dynamic Binding: A fundamental advantage of virtual functions is their ability to facilitate dynamic binding (late binding). The decision concerning which function to call is deferred until runtime, allowing the code to be flexible and adaptable.
- Override and Customisation: Virtual functions in derived classes can be overridden, allowing each derived class to give its own unique implementation. This allows for behaviour customisation, allowing you to enhance the functionality of base classes without altering current code.
- Common Interface: Virtual functions define a shared interface for a collection of related classes. This similarity makes it easier to use diverse classes inside the same framework, improving code organisation and readability.
- Run-Time Polymorphism: The runtime polymorphism achieved by virtual functions enables the selection of the appropriate function during programme execution based on the actual type of the object. This is especially useful in circumstances where the type of object is unknown until runtime.
- Abstract Classes and Pure Virtual Functions: Using pure virtual functions (functions with no implementation) in the base class, virtual functions can be utilised to construct abstract classes. Abstract classes cannot be instantiated, allowing derived classes to implement a clear interface.
The combination of these benefits makes virtual functions an effective tool for writing modular, extensible, and maintainable C++ programs. They contribute to essential object-oriented programming ideas such as encapsulation, inheritance, and polymorphism.
Limitations of Virtual Functions
While virtual functions provide major benefits, they are not without limitations and considerations. Here are a few of the most significant constraints of virtual functions in C++:
- Performance Overhead: Because of the additional degree of indirection necessary for dynamic dispatch, virtual functions might create a performance overhead. When opposed to static binding, the process of finding the proper function to call at runtime incurs a minor runtime penalty.
- Memory Overhead: A virtual function table (vtable) is present in every object that has at least one virtual function. In resource-constrained contexts, this table adds a little bit of memory overhead to each item, which can be an issue.
- Constructor Inflexibility: Constructors cannot be virtual in C++. Because of this constraint, the virtual function mechanism does not function as planned during building. The type of the object being built is always the class being directly instantiated, not the most derived type.
- Limited Use in Templates: When used with template classes, virtual functions are less effective. Templates rely on static polymorphism, but virtual functions include dynamic polymorphism. Combining the two can be difficult and may result in less optimal code.
- Complexity and Maintenance: Excessive use of virtual functions, particularly in big codebases, can result in increased complexity. Understanding and maintaining the links between base and derived classes, as well as their virtual functions, can be difficult, reducing code readability.
It is critical to balance the benefits and drawbacks of virtual functions based on the application's specific requirements. While virtual functions are a valuable tool for attaining polymorphism and code flexibility, developers should be aware of the potential impact they may have on performance, memory, and code complexity.
Example of C++ Virtual functions
C++ program that demonstrates the use of virtual functions
```
#include
// Base class
class Shape {
public:
// Virtual function for calculating area (to be overridden)
virtual double calculateArea() const {
return 0.0;
}
// Non-virtual function (not intended to be overridden)
void displayShapeType() const {
std::cout << "This is a generic shape." << std::endl;
}
};
// Derived class 1
class Circle : public Shape {
private:
double radius;
public:
// Constructor
Circle(double r) : radius(r) {}
// Overridden virtual function to calculate the area of a circle
double calculateArea() const override {
return 3.1415 * radius * radius;
}
// Additional function specific to Circle
void displayCircleInfo() const {
std::cout << "Circle with radius " << radius << std::endl;
}
};
// Derived class 2
class Rectangle : public Shape {
private:
double length;
double width;
public:
// Constructor
Rectangle(double l, double w) : length(l), width(w) {}
// Overridden virtual function to calculate the area of a rectangle
double calculateArea() const override {
return length * width;
}
// Additional function specific to Rectangle
void displayRectangleInfo() const {
std::cout << "Rectangle with length " << length << " and width " << width << std::endl;
}
};
int main() {
// Creating objects of the derived classes
Circle myCircle(5.0);
Rectangle myRectangle(4.0, 6.0);
// Using base class pointer to achieve polymorphism
Shape* shape1 = &myCircle;
Shape* shape2 = &myRectangle;
// Calling virtual function to calculate area
std::cout << "Area of shape 1: " << shape1->calculateArea() << std::endl;
std::cout << "Area of shape 2: " << shape2->calculateArea() << std::endl;
// Additional functions specific to derived classes
static_cast(shape1)->displayCircleInfo();
static_cast(shape2)->displayRectangleInfo();
return 0;
}
```
Explanation:
- The 'Shape' class has a virtual function 'calculateArea()' that derived classes can override. It also includes a non-virtual function called 'displayShapeType()'.
- The derived classes 'Circle' and 'Rectangle' inherit publically from 'Shape' and implement the 'calculateArea()' function.
- Objects of the derived classes ('myCircle' and'myRectangle') are generated in the 'main' method.
- Polymorphism is demonstrated by using base class pointers ('Shape*') to point to objects of derived classes.
- The 'calculateArea()' function is invoked via the base class pointer, and the derived classes' individual implementations are run based on the actual type of the objects.
- To demonstrate that polymorphism permits calling functions other than those defined in the base class, additional functions particular to each derived class ('displayCircleInfo()' and 'displayRectangleInfo()') are called.
This program demonstrates how to use virtual functions to achieve polymorphism, allowing for a uniform interface to interact with objects from many derived classes.