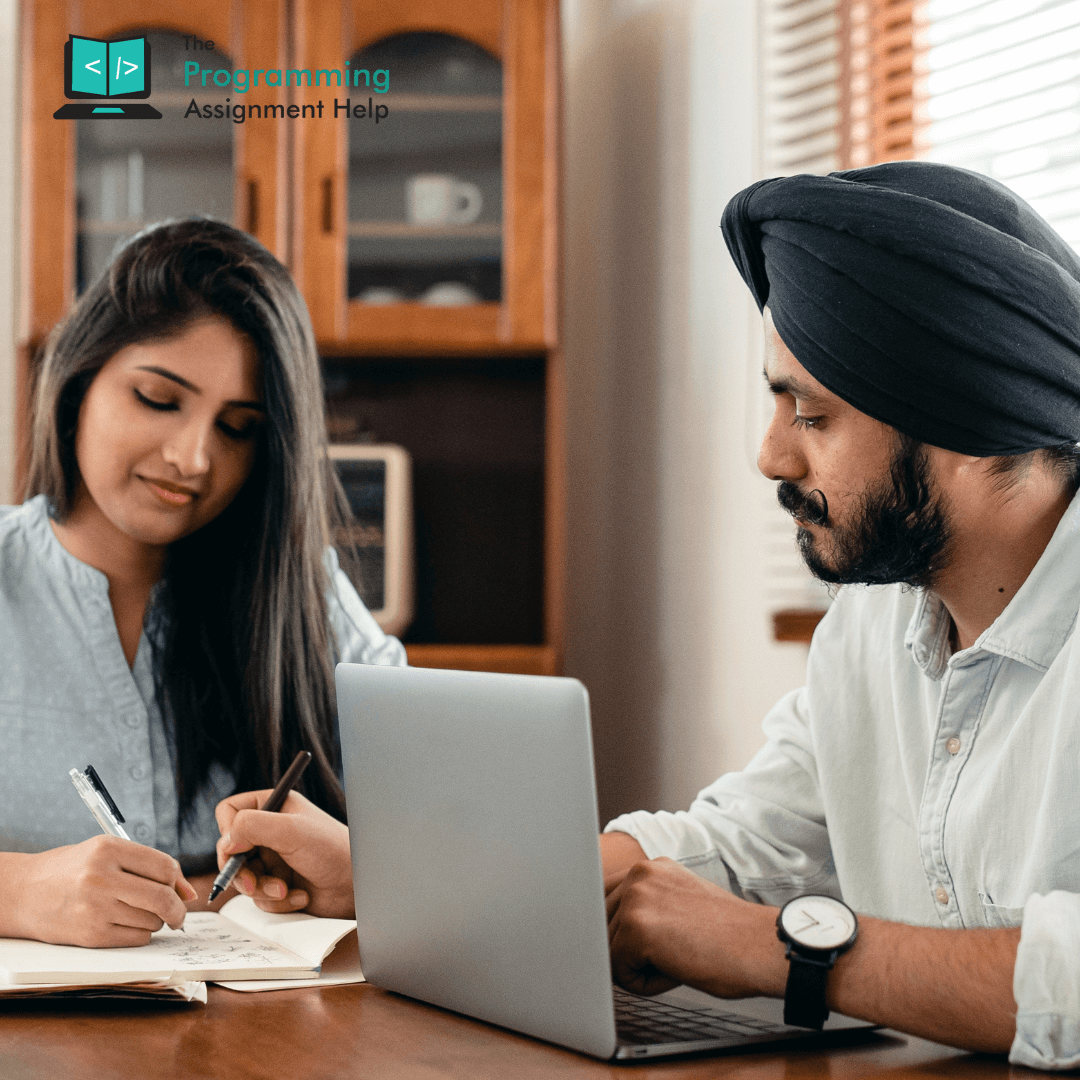
- 27th Jan 2022
- 03:47 am
The provided C++ program defines aBus
class that represents a bus and its driver. The class has a default constructor and a parameterized constructor to initialize the driver's name. It also includes getter and setter methods for the driver's name. Themain
function demonstrates the usage of theBus
class by creating objects of the class and manipulating their driver's names. Program #include using namespace std; class Bus{ public: Bus(){ cout << "Bus Default Constructor is called!" << endl; } Bus(string busDriverName){ //cout << "Bus driver’s name: " << busDriverName << "." << endl; this->name = busDriverName; } void printDriverName(){ cout << "Driver’s name is " << name << endl; } string getDriverName(){ return name; } void setDriverName(string busDriverName){ this->name = busDriverName; } private: string name; }; int main(){ cout << "Hello C++! I love CS52" << endl; Bus bus1; // Creating an object of class Bus. Bus bus2("Hathairat R"); // Creating another object of class Bus. bus2.printDriverName(); bus2.setDriverName("Alex M"); string name = bus2.getDriverName(); bus2.printDriverName(); return 0; }
The output of the program will be:
Hello C++! I love CS52
Bus Default Constructor is called!
Driver’s name is Hathairat R
Driver’s name is Alex M
Note: The using namespace std;
statement is included to avoid using the std::
prefix for standard library objects and functions. It is generally considered fine for small programs like this one, but it's a good practice to avoid it in large projects to prevent naming conflicts.