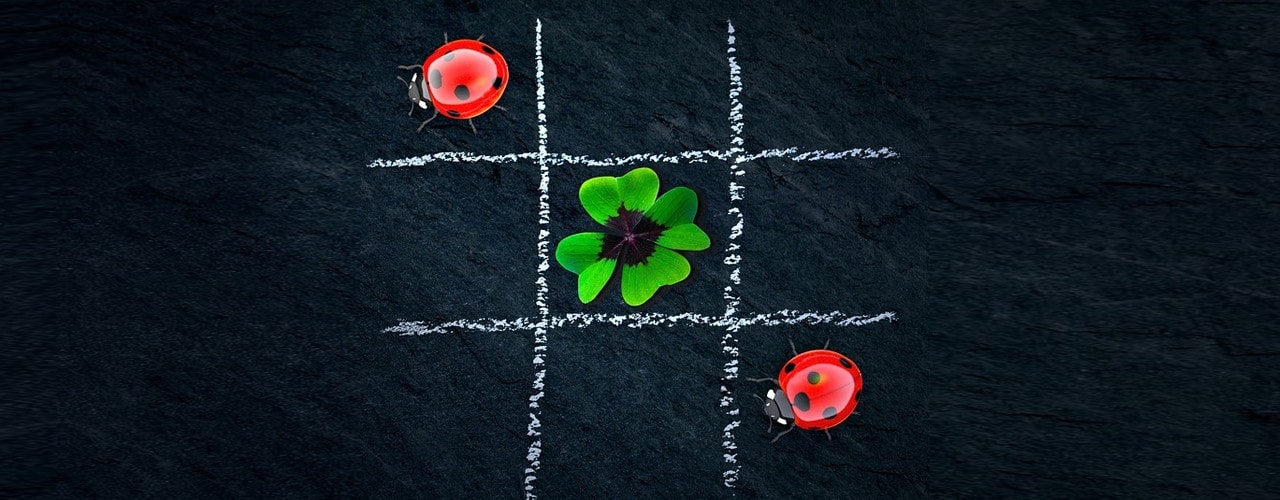
- 27th Feb 2019
- 04:01 am
The provided C++ code is an implementation of a simple command-line Tic-Tac-Toe game using the X Window System for graphics. It allows two players to take turns to play the game on a graphical window.
Please find below the C++ Program for Tic Tak Toe game. If you need help in coding a C++ game then reach out to our customer care.
/* Remember to compile try: g++ tictactoe.cpp -o tictactoe -I/usr/X11R6/include -I/usr/X11R6/include/X11 -L/usr/X11R6/lib -L/usr/X11R6/lib/X11 -lX11 */ /* include the X library headers */ #include < X11 / Xlib.h > #include < X11 / Xutil.h > #include < X11 / Xos.h > #include < stdio.h > #include < stdlib.h > Display * dis; int screen; Window win; GC gc; void init_x(); void close_x(); void redraw(); int checkForResult(int ticToeMatrix[3][3]); int main() { XEvent event; /* the XEvent declaration */ char text[255]; int result; init_x(); XNextEvent(dis, & event); XDrawRectangle(dis, win, gc, 50, 50, 30, 30); XDrawRectangle(dis, win, gc, 80, 50, 30, 30); XDrawRectangle(dis, win, gc, 110, 50, 30, 30); XDrawRectangle(dis, win, gc, 50, 80, 30, 30); XDrawRectangle(dis, win, gc, 80, 80, 30, 30); XDrawRectangle(dis, win, gc, 110, 80, 30, 30); XDrawRectangle(dis, win, gc, 50, 110, 30, 30); XDrawRectangle(dis, win, gc, 80, 110, 30, 30); XDrawRectangle(dis, win, gc, 110, 110, 30, 30); int chance = 0; int numberOfClicks = 0; int ticToeMatrix[3][3] = { 0 }; /* check events forever... */ while (1) { XNextEvent(dis, & event); /* Check for Button Press event */ if (event.type == ButtonPress) { int x = event.xbutton.x, y = event.xbutton.y; int i, j; if (x < 140 && x > 50 && y < 140 && y > 50) { if (x > 110) { x = 122; i = 0; } else if (x > 80) { x = 92; i = 1; } else if (x > 50) { x = 62; i = 2; } if (y > 110) { y = 132; j = 0; } else if (y > 80) { y = 102; j = 1; } else if (y > 50) { y = 72; j = 2; } if (ticToeMatrix[i][j] == 0) { chance == 0 ? strcpy(text, "X") : strcpy(text, "O"); numberOfClicks++; chance = !chance; ticToeMatrix[i][j] = chance + 1; XDrawString(dis, win, gc, x, y, text, strlen(text)); result = checkForResult(ticToeMatrix); if (result == 1) { strcpy(text, "O WINS"); XDrawString(dis, win, gc, 50, 40, text, strlen(text)); break; } else if (result == 2) { strcpy(text, "X WINS"); XDrawString(dis, win, gc, 50, 40, text, strlen(text)); break; } else if (numberOfClicks == 9) { strcpy(text, "DRAW!!!"); XDrawString(dis, win, gc, 50, 40, text, strlen(text)); break; } } } } } for (int i = 0; i < 3; i++) { for (int j = 0; j < 3; j++) { printf(" %d", ticToeMatrix[i][j]); } } XNextEvent(dis, & event); } int checkForResult(int ticToeMatrix[3][3]) { int count = 0; /* check for O to win */ for (int i = 0; i < 3; i++) { count = 0; for (int j = 0; j < 3; j++) { if (ticToeMatrix[i][j] == 1) { count++; } } if (count == 3) { return 1; } } count = 0; for (int i = 0; i < 3; i++) { count = 0; for (int j = 0; j < 3; j++) { if (ticToeMatrix[j][i] == 1) { count++; } } if (count == 3) { return 1; } } count = 0; for (int i = 0; i < 3; i++) { if (ticToeMatrix[i][i] == 1) { count++; } } if (count == 3) { return 1; } count = 0; for (int i = 0; i < 3; i++) { if (ticToeMatrix[i][2 - i] == 1) { count++; } } if (count == 3) { return 1; } /* check for X to win */ count = 0; for (int i = 0; i < 3; i++) { count = 0; for (int j = 0; j < 3; j++) { if (ticToeMatrix[i][j] == 2) { count++; } } if (count == 3) { return 2; } } count = 0; for (int i = 0; i < 3; i++) { count = 0; for (int j = 0; j < 3; j++) { if (ticToeMatrix[j][i] == 2) { count++; } } if (count == 3) { return 2; } } count = 0; for (int i = 0; i < 3; i++) { if (ticToeMatrix[i][i] == 2) { count++; } } if (count == 3) { return 2; } count = 0; for (int i = 0; i < 3; i++) { if (ticToeMatrix[i][2 - i] == 2) { count++; } } if (count == 3) { return 2; } /* for draw*/ return 0; } void init_x() { /* get the colors black and white for initilization */ unsigned long black, white; dis = XOpenDisplay((char * ) 0); screen = DefaultScreen(dis); black = BlackPixel(dis, screen), white = WhitePixel(dis, screen); win = XCreateSimpleWindow(dis, DefaultRootWindow(dis), 0, 0, 300, 300, 5, black, white); XSetStandardProperties(dis, win, "TIC-TAC-TOE", "", None, NULL, 0, NULL); XSelectInput(dis, win, ExposureMask | ButtonPressMask | KeyPressMask); gc = XCreateGC(dis, win, 0, 0); XSetBackground(dis, gc, white); XSetForeground(dis, gc, black); XClearWindow(dis, win); XMapRaised(dis, win); } void close_x() { XFreeGC(dis, gc); XDestroyWindow(dis, win); XCloseDisplay(dis); exit(1); } void redraw() { XClearWindow(dis, win); }
C++ Assignment Help Expert - Tic Tak Toe Code Analysis
Here's a brief overview of how the code works:
- The code includes necessary X11 header files for handling graphics.
- The
init_x()
function initializes the X Window System, creates a window, and sets properties like the window title. - The
close_x()
function closes the X Window System and frees resources when the game is finished. - The
redraw()
function clears the window to redraw the board after each move. - The
checkForResult()
function checks for a winning condition or a draw by examining the Tic-Tac-Toe matrix. - In the
main()
function, the game loop waits for X events (button press) to register player moves. - The game starts with an empty 3x3 Tic-Tac-Toe matrix, and players take turns to click on the board to place their marks ('X' or 'O').
- After each player's move, the
checkForResult()
function is called to check for a winner or draw condition. - The game continues until a winner is found or the board is full, resulting in a draw.
- The result is displayed on the window, and the game loop exits.
Please note that to compile and run this program successfully, you need to have the X Window System and the necessary libraries installed on your system. Additionally, you should run the program on a graphical environment, as it creates a graphical window to display the Tic-Tac-Toe board.
About the Author - Seddy
With a fervent dedication to decoding complex datasets and crafting innovative solutions, I am a seasoned programmer proficient in Statistical Data Analysis, Statistics, Statistical Modeling, and programming. My expertise lies at the intersection of technical acumen and analytical prowess, allowing me to engineer dynamic solutions that empower organizations to make data-driven decisions.