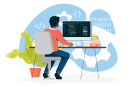
- 16th Nov 2023
- 23:36 pm
C++ Pointers
Pointers in C++ programming are variables that store memory addresses, enabling access to other variables indirectly. They are crucial for memory management, allowing dynamic allocation and deallocation. Each pointer occupies memory space equal to the size of the memory address.
Understanding pointers requires comprehension of the relationship between memory addresses and the pointers. By storing the address of a variable, pointers facilitate operations on the underlying data at that specific memory location. Syntax-wise, pointers are declared by adding an asterisk (*) after the data type.
#include
using namespace std;
int main() {
int num = 10;
int *ptr = #
cout << "Value of num: " << num << endl;
cout << "Address of num: " << &num << endl;
cout << "Value at address (dereferencing): " << *ptr << endl;
return 0;
}
Pointer Operations and Usage
- Dereferencing Pointers
Dereferencing, accomplished with the * operator, allows access to the value at the address a pointer holds. For instance, if a pointer 'ptr' points to a variable 'x', *ptr yields the value of 'x'.
- Pointer Arithmetic
Pointers can be incremented and decremented to navigate through memory addresses. For example, adding 1 to an integer pointer increases it by the size of the integer data type.
#include
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4};
int *ptr = arr;
for (int i = 0; i < 4; ++i) {
cout << "Element " << i + 1 << ": " << *ptr << endl;
ptr++;
}
return 0;
}
- Dynamic Memory Allocation
The 'new' operator dynamically allocates memory during runtime, providing the programmer control over memory usage. Conversely, 'delete' deallocates memory previously allocated using 'new', preventing memory leaks and managing the program's memory efficiently.
#include
using namespace std;
int main() {
int* dynamicPtr = new int;
*dynamicPtr = 25;
cout << "Value stored in dynamicPtr: " << *dynamicPtr << endl;
delete dynamicPtr;
return 0;
}
- Null Pointers
Null pointers, initialized with a value of 0 or nullptr, denote that the pointer does not point to a valid memory location. They are often used to avoid errors when a valid memory address isn't available.
#include
using namespace std;
int main() {
int* nullPtr = nullptr;
if (nullPtr == nullptr) {
cout << "The pointer is NULL" << endl;
} else {
cout << "The pointer is not NULL" << endl;
}
return 0;
}
- Pointers and Arrays
Pointers and arrays are closely related in C/C++. Arrays are essentially pointers to the first element of the array. Thus, incrementing a pointer to an array would point to the subsequent element in the array.
C++ References
In C++, references provide an alias for an existing variable, offering an alternative way to access data. Once assigned, a reference remains associated with the same variable throughout its lifecycle, cannot be null, and must be initialized during declaration.
The syntax for references involves adding an ampersand (&) after the data type, e.g., 'int& ref = x;' creates a reference 'ref' that refers to the variable 'x'. References offer a more intuitive and direct notation compared to pointers, streamlining code readability and aiding in expressing clear, direct relationships between variables.
#include
using namespace std;
int main() {
int a = 10;
int &ref = a;
cout << "Value of a: " << a << endl;
cout << "Value using reference: " << ref << endl;
ref = 20;
cout << "New value of a: " << a << endl;
return 0;
}
Definition and Syntax of C++ References
References in C++ act as alternative names for variables and are initialized by placing an ampersand (&) after the data type, creating an alias for an existing variable. For instance, 'int& ref = x;' associates a reference 'ref' with the variable 'x'.
Once set, a reference cannot be reassigned to point to another variable. Their syntactic clarity and direct representation make references a practical and straightforward tool in C++ programming.
- References vs. Pointers
References and pointers, while both used to access data indirectly in C++, exhibit distinct characteristics. References serve as an alias to a variable, whereas pointers store memory addresses. Unlike pointers, references cannot be null and must be initialized during declaration. They cannot be re-assigned to refer to a different variable. In contrast, pointers can be reassigned to point to different memory addresses.
Additionally, references provide a more intuitive and user-friendly notation compared to pointers, simplifying code and making relationships between variables more evident. Each has its unique applications and best practices in C++ programming.
#include
using namespace std;
int main() {
int num = 10;
int* ptr = #
int& ref = num;
cout << "Value of num: " << num << endl;
cout << "Value through pointer: " << *ptr << endl;
cout << "Value through reference: " << ref << endl;
*ptr = 20;
ref = 30;
cout << "New value of num: " << num << endl;
return 0;
}
Pointers and References in Functions
Pointers and references play significant roles in C++ functions, particularly in altering data within functions or enhancing performance by avoiding unnecessary copies.
- Passing by Reference:
When passing arguments by reference to functions, any modification within the function directly affects the original variable, streamlining data changes and eliminating the need for additional memory allocation.
#include
using namespace std;
void modifyReference(int &ref) {
ref = 100;
}
int main() {
int x = 50;
modifyReference(x);
cout << "Updated value of x: " << x << endl;
return 0;
}
- Passing by Pointer:
Passing arguments by pointers provides similar functionalities to references, allowing direct access and modification to the original variable. It facilitates more control over data manipulations within functions.
#include
using namespace std;
void modifyPointer(int *ptr) {
*ptr = 1000;
}
int main() {
int y = 500;
int *pointer = &y;
modifyPointer(pointer);
cout << "Updated value of y: " << y << endl;
return 0;
}
- Returning Pointers and References:
Functions can return pointers or references, allowing the calling function to access or modify the returned values, streamlining the handling of data structures and memory. These methodologies facilitate efficient data manipulation in C++ programs.
#include
using namespace std;
int* createArray() {
int* arr = new int[5]{1, 2, 3, 4, 5};
return arr;
}
int main() {
int* newArray = createArray();
for (int i = 0; i < 5; ++i) {
cout << "Element " << i << ": " << newArray[i] << endl;
}
delete[] newArray;
return 0;
}
Blog Author Profile - Radhika Joshi
Radhika Joshi is a seasoned programming expert with a profound academic background in Computer Science and Machine Learning. Her dedication to the field has been fueled by her relentless pursuit of knowledge and her commitment to pushing the boundaries of technology. PhD in Computer Science from a prestigious university in the United States. Her doctoral research focused on cutting-edge advancements in advanced machine learning algorithms and techniques.