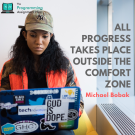
- 27th Oct 2023
- 21:26 pm
What Is Bubble Sort Algorithm?
Bubble Sort is a simple and intuitive sorting algorithm used to arrange elements in a specific order, typically ascending or descending. It works by repeatedly stepping through the list of elements, comparing each pair of adjacent items, and swapping them if they are in the wrong order. This process is akin to the way bubbles rise to the surface in a liquid.
The process involves iterative steps where adjacent elements are compared and swapped. In each iteration, the largest or smallest element gradually moves to its correct position at the list's end. This repetition continues until the entire list is appropriately sorted.
However, Bubble Sort is relatively inefficient for large datasets as it has a time complexity of O(n^2) in the worst and average cases. This makes it less practical for large-scale sorting operations compared to more efficient algorithms like quicksort or merge sort. Despite its inefficiency, Bubble Sort is a fundamental concept often introduced early in programming education to demonstrate sorting techniques and algorithms.
How Does Bubble Sort Work?
Bubble Sort, a straightforward sorting algorithm, works by repeatedly stepping through the elements in a list, comparing adjacent items, and swapping them if they are in the wrong order. The algorithm's name comes from the way smaller elements "bubble" to the top of the list, similar to how bubbles rise to the surface in a liquid.
The process begins by comparing the first two elements and swapping them if necessary. The algorithm then moves to the next pair of elements, comparing and swapping them as needed. This comparison and swapping procedure is repeated for each adjacent pair throughout the list, with larger elements "bubbling up" towards the end.
In each iteration, the largest (or smallest, based on the desired order) element is effectively pushed to the correct position. The process continues until the entire list is sorted, signified by the absence of any more swaps during an iteration.
However, Bubble Sort's simplicity comes at the cost of efficiency. It has a time complexity of O(n^2), making it less efficient for large datasets. Despite this drawback, Bubble Sort serves as a fundamental concept for understanding sorting algorithms and their basic principles.
Let's consider an example of using the Bubble Sort algorithm to sort an array of integers in ascending order:
Original Array:
[5,2,9,3,1]
First Pass: Compare and swap adjacent elements:
[2,5,3,1,9]
Second Pass: Compare and swap adjacent elements:
[2,3,1,5,9]
Third Pass: Compare and swap adjacent elements:
[2,1,3,5,9]
Fourth Pass: Compare and swap adjacent elements:
[1,2,3,5,9]
After four passes, the array is sorted in ascending order using the Bubble Sort algorithm.
Keep in mind that Bubble Sort is not efficient for large datasets, and there are more efficient sorting algorithms for practical applications. However, it serves as a fundamental sorting technique and is often used for educational purposes or small datasets.
Explain The Implementation Of Bubble Sort
Here's a simple implementation of the Bubble Sort algorithm in Python:
Python
def bubble_sort(arr):
n = len(arr)
# Traverse through all elements in the array
for i in range(n):
# Last i elements are already in place, so no need to check them
for j in range(0, n-i-1):
# Swap if the element found is greater than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
# Example usage
if __name__ == "__main__":
arr = [5, 2, 9, 3, 1]
print("Original Array:", arr)
bubble_sort(arr)
print("Sorted Array:", arr)
In this Python implementation, the bubble_sort function takes an array as an argument and sorts it using the Bubble Sort algorithm. It iterates through the array, comparing adjacent elements and swapping them if they are in the wrong order. This process is repeated for each element until the entire array is sorted.
The example usage demonstrates how to sort an array using the bubble_sort function and prints the original and sorted arrays.
Bubble Sort Code Snippet In Python And Other Programming Languages
Bubble Sort is a simple sorting algorithm where adjacent elements in a list are compared and swapped if they're in the wrong order. This process is repeated until the entire list is sorted.
In Python, you can implement Bubble Sort by using nested loops to traverse the list, comparing adjacent elements, and swapping them if needed.
Similarly, in Java and C++, you can use nested loops to traverse the array, comparing adjacent elements and swapping them as required to sort the array.
Bubble Sort is a straightforward algorithm often used for educational purposes or for small datasets due to its simplicity. However, it's not efficient for large datasets, and other sorting algorithms like quicksort or mergesort are preferred for practical applications due to their better performance.
What Are The Advantages of Using Bubble Sort In Your Assignment?
Bubble Sort, despite being a simple and often inefficient sorting algorithm, has a few advantages:
- Ease of Implementation: Bubble Sort is one of the simplest sorting algorithms to understand and implement. It involves straightforward logic based on comparing adjacent elements and swapping them if necessary.
- No Additional Space: Bubble Sort sorts the elements in place, meaning it doesn't require additional memory or space to sort the given array. This is particularly useful when memory is a concern.
- Adaptability: Bubble Sort can be adapted to detect whether the input list is already sorted. If the list is already sorted, it can stop early, making it efficient for nearly sorted lists or lists with only a few elements out of order.
- Useful for Educational Purposes: Bubble Sort serves as a fundamental example of a sorting algorithm. It is often used in introductory computer science courses to illustrate sorting concepts due to its simplicity and easy-to-understand logic.
Despite these advantages, Bubble Sort is not efficient for large datasets and is generally not used in practical applications where more efficient sorting algorithms are preferred for their better time complexity and performance.
What Are The Disadvantages Of Using Bubble Sort?
Bubble Sort, while simple to understand and implement, has several significant disadvantages:
- Inefficiency for Large Datasets: Bubble Sort has a time complexity of O(n^2) in the average and worst cases. This means its performance worsens significantly as the number of elements to be sorted increases. For large datasets, it becomes highly inefficient compared to more optimized sorting algorithms like quicksort or mergesort.
- Poor Performance: Bubble Sort has poor performance characteristics, especially in scenarios where elements are far from their sorted positions. The algorithm requires multiple passes through the array, making it impractical for handling sizable or unsorted datasets.
- Not Suitable for Real-World Applications: Due to its inefficiency, Bubble Sort is seldom used in real-world applications where sorting large amounts of data is common. Faster algorithms like quicksort, mergesort, heapsort, or even built-in sorting functions in programming languages are preferred for efficiency.
- Lack of Practicality: While it's straightforward to implement, Bubble Sort's slow performance and inefficiency make it impractical for any serious application or large-scale data sorting tasks. There are more efficient algorithms available that can sort data much faster.
- Not Adaptive to Changes: Bubble Sort does not adapt well to changes in the dataset. Even if a small portion of the dataset is already sorted, Bubble Sort still goes through all the passes, resulting in unnecessary comparisons and swaps.
In summary, Bubble Sort is primarily used for educational purposes and small datasets due to its simplicity, but its inefficiency and poor performance characteristics limit its practical use in real-world applications.
Various Bubble Sort Applications Described By Our Experts
Application | Description |
Educational Purposes | Often the first sorting algorithm taught in computer science courses, provides a simple introduction to fundamental concepts like loops, conditionals, and array manipulation. Acts as a foundational sorting algorithm before learning more complex alternatives. |
Small Datasets | Suitable for very small datasets or nearly sorted arrays where the simplicity and ease of implementation outweigh the inefficiencies. In such cases, the impact on performance is minimal, making it a feasible choice. |
Teaching Sorting Algorithms | Utilized as a teaching aid to illustrate sorting techniques and fundamental concepts in computer science. Comparing Bubble Sort with more efficient sorting algorithms, it helps in understanding the importance of algorithmic efficiency. |
Algorithm Complexity Analysis | Acts as a reference point for contrasting time complexity against more efficient sorting algorithms. Facilitates a deeper understanding of algorithm analysis and comparison based on time complexity (O(n^2) for Bubble Sort). |
Mock Interviews and Coding Challenges | May be used in mock interviews or coding challenges to evaluate problem-solving skills, understanding of basic algorithms, and the ability to implement a sorting algorithm, even if not the most efficient choice. |
In summary, Bubble Sort is predominantly utilized in educational settings, as a teaching tool, for analyzing algorithm complexity, and occasionally in coding assessments. Its inefficiency limits its usage in practical, large-scale applications.
Why Choose Theprogrammingassignmenthelp.com For Bubble Sort Assignment Help?
If you're grappling with a bubble sort assignment, we at TheProgrammingAssignmentHelp.com are here to rescue you. Wondering why you should consider teaming up with us?
Here are some top reasons:
- We Know Our Stuff: Our squad is a bunch of coding wizards who totally get bubble sort and a bunch of programming languages. We've been around the block and have seen it all, so you can trust us to handle your assignment like pros.
- We Don't Compromise on Quality: Quality is our jam. When you reach out for help with your bubble sort assignment, you'll get solutions that are spot-on, well-organized, and up to the mark. We pay attention to detail and follow your instructions to the T.
- We're on Time, Every Time: We get it—deadlines matter. Your bubble sort assignment will hit your inbox well before the clock ticks down to zero. We're all about making sure you have time to review and ask for tweaks if needed.
- Originality is Key: Your assignment is one of a kind, and we treat it that way. No copy-pasting or shady business here. Our solutions for your bubble sort assignment are crafted fresh, just for you.
- Your Secrets Are Safe: Privacy matters, and we've got your back. Your details and assignment info are safe and sound with us. We don't spill the beans to anyone.
- Affordable Awesomeness: Quality doesn't have to cost an arm and a leg. Our prices are budget-friendly, making sure you can get top-notch help without emptying your wallet.
- We're Always Here: Got questions? Need a hand? We're here 24/7. Reach out, and our awesome support team will sort you out.
Come on over to theProgrammingAssignmentHelp.com, and let's crack that bubble sort assignment together. We're all about helping you nail this!