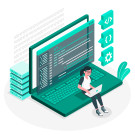
- 14th Nov 2023
- 15:40 pm
Breadth-First Search (BFS) stands as a fundamental algorithm utilized for traversing and searching through tree or graph data structures. This algorithm explores a graph systematically by visiting its neighbors before moving on to the next level of nodes. It operates in a way where all vertices at the current level are visited before proceeding to the next level in a hierarchical manner.
What is BFS?
Breadth-First Search (BFS) is a fundamental graph traversal algorithm that systematically explores nodes or vertices. It achieves this by thoroughly searching through all vertices at a specific depth level before advancing to deeper levels within a tree or a graph. Unlike depth-first approaches, BFS prioritizes examining nodes level by level, starting at the initial vertex and expanding into its immediate neighbors before proceeding further. The algorithm operates on the principle of breadth-first expansion, allowing it to methodically cover all nodes at a specific level before advancing to deeper levels.
BFS begins with a designated starting vertex, where it systematically explores the immediate neighbors of that vertex. As it progresses, it moves level by level, visiting all vertices at the current level before delving into deeper levels. This approach ensures that BFS moves through the graph or tree in a layered, hierarchical manner, offering a broader view of the structure before diving deeper into its intricacies.
BFS Algorithm
Breadth-First Search (BFS) stands as a fundamental graph traversal algorithm, systematically exploring the vertices or nodes within a graph in a level-by-level fashion. This exploration method operates by visiting all the immediate neighbors of the starting vertex, then moves to their neighbors before proceeding further. The methodology harnesses a queue data structure to control and manage the order of nodes as they undergo processing. Starting at the source node, BFS expands outward to cover nodes layer by layer, ensuring a methodical examination of the graph. This approach allows for a comprehensive examination of all nodes within the same level before delving deeper into the graph structure.
BFS is commonly used in various fields like pathfinding, network analysis, and component connections within a graph due to its orderly exploration of vertices. Its level-wise traversal approach aids in detecting the shortest path and exploring connected components, making it an essential algorithm in graph theory and problem-solving.
def bfs(graph, start):
visited = set()
queue = [start]
visited.add(start)
while queue:
node = queue.pop(0)
print(node, end=" ")
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
BFS Pseudocode
The Breadth-First Search (BFS) algorithm can be outlined in a pseudocode format to illustrate its step-by-step operation. The pseudocode typically involves initializing a queue to track nodes and marking the initial node as visited. It begins by enqueuing the source node, then explores its neighbors. As it processes nodes, BFS marks them as visited to avoid redundant iterations. The procedure continues until the queue is empty, systematically exploring all adjacent nodes in the graph. The pseudocode conveys the logic and operation of the BFS algorithm, ensuring an organized and level-wise exploration of the graph's nodes.
BFS Implementation in Python, Java, and C/C++
BFS implementation in Python, Java, and C/C++ is relatively similar in concept but varies in syntax and language-specific elements. All three languages can accomplish BFS by using queue data structures, collections, or arrays to track visited nodes and manage the order of traversal. The Python implementation might make use of in-built data structures like lists or deque from the collections module. Java and C/C++ typically involve the utilization of classes or libraries to handle queues or arrays efficiently for the same BFS approach. While the specific syntax may differ, the core BFS strategy remains consistent across Python, Java, and C/C++ when it comes to graph or tree traversal.
- Python BFS Implementation:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
node = queue.popleft()
print(node, end=' ')
for neighbor in graph[node]:
if neighbor not in visited:
queue.append(neighbor)
visited.add(neighbor)
- Java BFS Implementation:
import java.util.*;
public class BFS {
public void bfs(Map> graph, int start) {
Set visited = new HashSet<>();
Queue queue = new LinkedList<>();
queue.add(start);
visited.add(start);
while (!queue.isEmpty()) {
int node = queue.poll();
System.out.print(node + " ");
for (int neighbor : graph.get(node)) {
if (!visited.contains(neighbor)) {
queue.add(neighbor);
visited.add(neighbor);
}
}
}
}
}
- C++ BFS Implementation:
#include
#include
#include
#include
#include
void bfs(std::unordered_map>& graph, int start) {
std::unordered_set visited;
std::queue q;
q.push(start);
visited.insert(start);
while (!q.empty()) {
int node = q.front();
std::cout << node << " ";
q.pop();
for (int neighbor : graph[node]) {
if (visited.find(neighbor) == visited.end()) {
q.push(neighbor);
visited.insert(neighbor);
}
}
}
}
Time Complexity of BFS Implementation in Python
The time complexity of BFS is often denoted as O(V + E). V stands for the number of vertices, while E indicates the number of edges present in the graph.
- Time Complexity:
The time complexity for Breadth-First Search (BFS) in Python is usually described as O(V + E). Here, V represents the count of vertices, and E denotes the quantity of edges in the graph. BFS methodically explores through all the vertices and examines each edge just once. For each vertex, it visits all its connected vertices. In an adjacency list representation, where every vertex is linked to an average of 'k' vertices, the time complexity can be articulated as O(V + kV), ultimately simplifying to O(V) in graphs with sparser connections.
- Space Complexity:
Regarding space complexity, BFS utilizes a queue data structure to store the nodes it plans to visit. The required space grows in proportion to the number of vertices in the graph. Therefore, the space complexity of BFS stands at O(V), where V represents the count of vertices. The queue data structure holds the nodes to be visited, and as the algorithm navigates through each vertex and its linked vertices, the queue's memory usage directly corresponds to the number of vertices present in the graph.
Application of BFS
BFS finds extensive applications in various areas, including:
- Shortest Path Finding: Utilized in graph theory to find the shortest path from a source node to a destination node.
- Network Analysis: Used to discover the shortest route between nodes in a network.
- Social Networking: Implemented for friend suggestions or to determine mutual connections.
- Web Crawling: Employed by search engines to explore and index web pages.
BFS is a fundamental algorithm that serves as a base for more complex traversal and searching algorithms. Its structured exploration of graphs and trees makes it a valuable tool in various domains.