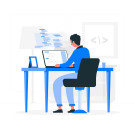
- 14th Nov 2023
- 20:55 pm
- Admin
Access modifiers in Java regulate the visibility of classes, variables, methods, and constructors, determining their accessibility within the program. These modifiers are crucial in upholding security, encapsulation, and controlling access to elements, ensuring a structured and secure coding environment. They determine the level to which different elements can be accessed or modified, thereby influencing the overall structure and security measures within the program.
What are Access Modifiers in Java?
Access modifiers in Java are pivotal in deciding the accessibility and visibility of classes, methods, and variables within a program. They define the scope of access and provide a level of control over how these elements can be interacted with and utilized. Access modifiers in Java enable developers to control the visibility of classes, methods, and variables, thereby enhancing security and encapsulation within the codebase.
The access modifiers in Java Default, Private, Protected, and Public designate various levels of accessibility. Default access limits access to the same package, while Private confines access to the class in which it is declared. The "protected" access modifier grants access to classes within the same package and their subclasses. Conversely, "public" offers unrestricted access from any part of the program.
By employing these access levels, developers can maintain a structured and secure codebase, ensuring that only permitted components are accessible, thus adhering to the principles of information hiding and fostering a more organized and secure software structure.
Types of Access Modifiers in Java
Access modifiers in Java provide specific levels of control over the visibility and accessibility of classes, methods, and variables within a program. They determine the boundaries for interaction with these program elements, thereby ensuring a secure and well-organized codebase. These modifiers are a fundamental aspect of the Java language, granting developers the power to regulate the visibility of their classes, methods, and variables.
- Default Access Modifier:
Default access applies to elements within the same package. When no other modifier is specified, the default access level is set.
class DefaultExample {
void display() {
System.out.println("This is the Default Access Modifier");
}
}
- Private Access Modifier:
Restricts access to the class where it is declared, ensuring that the element is only accessible within the class itself.
class PrivateExample {
private void display() {
System.out.println("This is the Private Access Modifier");
}
}
- Protected Access Modifier:
Extending accessibility to classes within the same package and their subclasses, even if they are present in different packages.
class Parent {
protected void display() {
System.out.println("This is the Protected Access Modifier");
}
}
class Child extends Parent {
void show() {
display(); // Accessing protected method from the parent class
}
}
- Public Access Modifier:
Enables unrestricted access to the element from any part of the program, ensuring accessibility without limitations.
public class PublicExample {
public void display() {
System.out.println("This is the Public Access Modifier");
}
}
Algorithm to Use Access Modifier in Java
Following this systematic algorithm empowers developers to precisely manage the visibility and accessibility of their code elements, thereby promoting structured, secure, and efficient software design in Java.
When utilizing access modifiers in Java, the process typically follows a straightforward algorithm:
- Understand Access Requirements: Identify the necessary visibility level for classes, methods, or variables to ensure proper data encapsulation and security.
- Apply the Suitable Modifier: Choose the access modifier (Default, Private, Protected, or Public) that aligns with the desired level of accessibility.
- Set the Modifier Accordingly: Apply the chosen modifier to the class, method, or variable to establish the intended level of access control.
- Test and Verify: Verify that the accessibility operates as intended. Ensure that the selected access level permits or restricts access to the designated components, avoiding unexpected behaviors or security loopholes.
Java Access Modifiers with Method Overriding
In Java, method overriding involving access modifiers mandates certain rules to maintain consistency between overridden and overriding methods. When a subclass provides a specific implementation for a method already defined in its superclass, these access rules ensure uniformity in visibility:
- Preservation of Access Levels: When overriding a method in Java, the access level of the overriding method must not be more restrictive than that of the overridden method in the superclass. It can widen the accessibility but cannot narrow it.
- Access Widening: For instance, if the superclass method is public, the overriding method in the subclass cannot be private or default. It can be public or protected, broadening the accessibility.
- Inherited Access Levels: Overridden methods uphold the access level of the superclass methods, preserving a cohesive class relationship without compromising the inherited access level.
Advantages of Access Modifiers in Java
Access modifiers in Java bring several advantages that greatly contribute to the structure, security, and integrity of a program. Access modifiers in Java provide several advantages in programming:
- Data Encapsulation: Access modifiers facilitate encapsulation by allowing the control of data visibility, enhancing security by hiding sensitive data and methods from external access.
- Enhanced Security: By restricting access to classes, methods, and variables, access modifiers prevent unauthorized tampering and protect critical functionalities.
- Controlled Access: They enable fine-grained control over data, limiting exposure to only what is necessary, contributing to a more organized and manageable codebase.
- Maintainability: Access modifiers promote code maintenance by providing a structured approach to managing class elements, allowing modifications to be made with minimal impact on other code segments.
- Code Reusability: Controlled access to methods and variables enables safe reuse, reducing redundancy and promoting efficient coding practices.
Disadvantages of Access Modifiers in Java
While access modifiers in Java have numerous advantages, they also come with specific limitations that developers must take into account. Although access modifiers in Java provide various benefits, they also come with certain limitations:
- Increased Complexity: Using multiple access modifiers can lead to complex code structures, making code comprehension and maintenance more challenging.
- Potential Misuse: Improper use of access modifiers may inadvertently restrict intended access, leading to unexpected or unintended behavior within the program.
- Limitation on Flexibility: Over-restrictive access may hinder the flexibility of classes or methods, limiting their use and reusability in different contexts.
- Complexity in Inheritance: In certain cases, restrictive access levels might complicate inheritance hierarchies, requiring careful planning to maintain the intended behavior.
About The Author - Janhavi Gupta
Seasoned programmer with 6 years of experience in designing, developing, and deploying software solutions. Strong problem-solving skills coupled with the ability to collaborate effectively in multidisciplinary teams. Continuously learning and adapting to new technologies and methodologies to drive innovation and deliver high-quality, user-centric solutions.