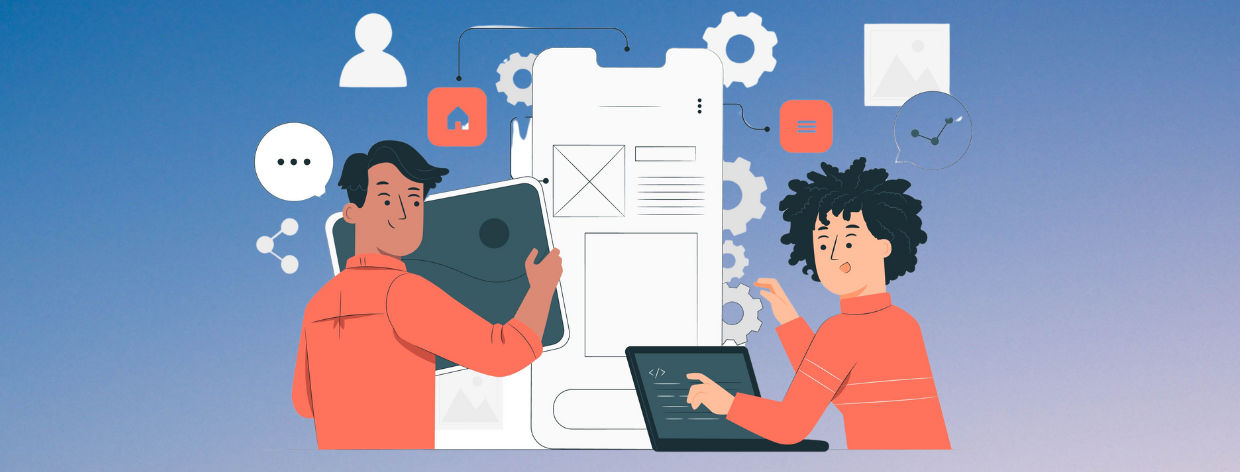
- 4th Mar 2024
- 15:30 pm
- Admin
Data structures, The basic general idea of a data structure is about the capability of authorizing neatly holding or managing different categories of data. Besides, some of the fundamental operations like search, insert, and delete may be realized in varying efficiencies that suit the preference of the data structure and its implementation.
While basic operations often provide a solid foundation, they can encounter limitations in specific scenarios. For example, searching a large linear array might become inefficient due to its sequential nature. Similarly, basic implementations of hash tables might struggle with collisions, reducing performance. Additionally, basic data structures might lack functionalities crucial for specific tasks, such as efficient range queries or maintaining specific ordering within the data.
To address these limitations and unlock the full potential of data structures, advanced techniques come into play. These techniques optimize performance, enhance functionality, and handle dynamic data sets effectively. By delving deeper into these advanced techniques, programmers can leverage the full power of various data structures to tackle complex problems with greater efficiency and flexibility.
Ready to take your understanding of data structures to the next level? Explore advanced techniques and unlock their potential for efficient problem-solving! Our experts offer comprehensive Data Structure Assignment Help and Data Structure Homework Help to guide you through the journey.
Advanced Techniques:
Beyond the fundamental operations of searching, insertion, and deletion, trees offer a rich set of advanced techniques to tackle complex data management challenges. Let's explore two powerful techniques: 1. Self-Balancing Trees and 2. B-Trees.
Self-Balancing Trees:
These trees maintain a balanced structure, ensuring efficient search, insertion, and deletion operations even with dynamic data sets. Common examples include:
- AVL Trees: These trees enforce a strict height balance, limiting the difference in height between any two subtrees to one. Insertion and deletion operations involve rotations to maintain this balance, ensuring a worst-case time complexity of O(log n) for all operations.
class Node:
def __init__(self, data):
self.data = data
self.left = None
self.right = None
class AVLTree:
def __init__(self):
self.root = None
# Implementation of insertion and deletion operations with rotations for balancing
# ...
# Example usage
tree = AVLTree()
tree.insert(10)
tree.insert(5)
tree.insert(15)
- Red-Black Trees: These trees maintain a balance by enforcing specific properties like no node having two consecutive red children. Similar to AVL trees, rotations are performed during insertion and deletion to maintain these properties, guaranteeing a worst-case time complexity of O(log n) for all operations.
B-Trees:
These specialized trees are particularly efficient for storing and managing large datasets on disk. They utilize a multi-way structure, allowing each node to have multiple child nodes. This design optimizes disk access by storing a larger number of keys and child pointers in a single node compared to regular binary trees.
class BTreeNode:
def __init__(self, t): # t is the minimum number of keys per node
self.n = 0
self.keys = [None] * (2 * t - 1)
self.C = [None] * (2 * t) # child pointers
class BTree:
def __init__(self, t):
self.root = None # Root node
self.t = t # Minimum number of keys per node
# Implementation of search, insertion, and deletion operations optimized for disk access
# ...
# Example usage
btree = BTree(3)
btree.insert(10)
btree.insert(20)
btree.insert(5)
print(btree.search(10))
Both self-balancing trees and B-trees offer significant advantages over basic tree implementations. Self-balancing trees ensure efficient search and update operations even with unbalanced data sets, while B-trees optimize storage and retrieval for large datasets residing on disk. These advanced techniques empower programmers to handle complex data structures with greater efficiency and flexibility.
Advanced Libraries and Implementations:
While exploring advanced techniques for data structures deepens your understanding, leveraging optimized implementations available in libraries and frameworks can significantly boost your development efficiency. This section delves into the standard library offerings and popular third-party libraries in various programming languages that provide efficient implementations of data structures, including the previously discussed advanced techniques.
Standard Library Implementations:
C++ Standard Template Library (STL): The C++ STL offers a comprehensive collection of data structures and algorithms, including:
- set and multiset: An ordered set and multiset is, in essence, a self-balancing tree implementation, normally a red-black tree, to ensure a maximum time of O(log n) on the set of operations for search, insertion, and removal.
- map and multimap: Represent kinds of self-balancing trees usually red–black tree in C++, which is an associative container that maps keys to values that offer efficient retrieval and manipulation of pairs with respective keys, and there is a choice between keys with unique mappings and keys with non-unique mappings.
- unordered_set and unordered_map: These are general-purpose implementations are hash-based implementations for fast average-case searching, insertion, and deletion without guaranteed bounds on the time to perform these operations in the worst case.
Python Collections: The Python collections module offers various data structures, including:
- set: A set is used, working in function the same way as C++ set; that is, it implements a self-balanced tree, normally an AVL tree, for efficient operations depending on keys that hold unique values.
- dict: Python's dictionary utilizes a hash table for efficient key-based lookup and modification, similar to C++'s unordered_map.
- collections.defaultdict: A specialized dictionary that allows specifying a default value to be returned if a key is not found, enhancing code readability and reducing the need for explicit key checks.
Third-Party Libraries:
Boost.Container (C++): This library offers additional data structures not included in the STL, including:
- bptree: An implementation of B-trees, enabling efficient storage and retrieval of large datasets on disk.
- flat_set and flat_map: Lock-free implementations of self-balancing trees designed for concurrent access in multithreaded environments.
guava (Java): This open-source library provides various data structures, including:
- com.google.common.collect.ImmutableSet and com.google.common.collect.ImmutableMap: Immutable implementations of sets and maps, offering improved thread-safety and memory efficiency compared to mutable counterparts.
- com.google.common.collect.Multimap: A flexible implementation of multimaps, allowing efficient storage and retrieval of elements associated with the same key.
Choosing the Right Technique:
While exploring advanced data structure techniques unlocks their potential, choosing the right one for a specific scenario is crucial. This section outlines key factors and guidelines to consider when making this decision:
Problem Requirements and Constraints:
- Data Size and Distribution: Understanding the expected data size (small, medium, large) and distribution (uniform, skewed) is crucial. For instance, self-balancing trees might be overkill for small, uniformly distributed datasets where simpler structures suffice.
- Operation Frequency: Identify the operations your application performs most frequently (search, insertion, deletion). Techniques like B-trees excel for frequent searches on large datasets, while self-balancing trees might be suitable for scenarios with frequent insertions and deletions.
- Memory Constraints: If memory usage is a critical concern, consider techniques that optimize memory usage. For example, hash tables generally require less memory compared to self-balancing trees for the same number of elements.
Performance Considerations:
- Time Complexity: Evaluate the time complexity (e.g., O(log n) vs. O(n)) of different techniques for the operations your application performs most frequently. Choose the technique that offers the best time complexity for those operations.
- Space Complexity: Analyze the space complexity (e.g., O(n) vs. O(log n)) of different techniques, considering the available memory resources and the impact on overall application performance.
Trade-offs Between Techniques:
- Self-Balancing Trees vs. Hash Tables: Self-balancing trees offer worst-case guarantees for search, insertion, and deletion, but might require more memory compared to hash tables. Hash tables excel in average-case search but lack worst-case guarantees and might not maintain order.
- Self-Balancing Trees vs. B-Trees: Self-balancing trees are generally more memory-intensive than B-trees but offer efficient in-memory operations. B-trees optimize storage and retrieval for large datasets on disk but might have slightly slower in-memory performance compared to self-balancing trees.
Additional Tips:
- Start with simpler solutions: If possible, consider simpler data structures and techniques first. Introduce advanced techniques only when necessary to address specific performance bottlenecks or handle complex data access patterns.
- Benchmark and compare: For critical scenarios, consider implementing and comparing the performance of different techniques with your actual data and workload to make an informed decision.
Applications in Different Scenarios:
Beyond theoretical concepts, advanced data structure techniques play a crucial role in solving real-world problems across various domains. Let's explore how these techniques are applied in different scenarios:
Algorithm Design and Optimization:
- Self-Balancing Trees in Spell Checkers: Spell checkers often make use of self-balancing trees stored in dictionaries of correctly spelled words (like AVL trees) in order that search and new insertion are to be made in an efficient way, more so if the dictionary extends.
- B-Trees in Database Indexing: Databases frequently utilize B-trees to index data. This optimized storage structure allows for efficient retrieval of specific records based on search criteria, significantly improving query performance, especially for large datasets.
Efficient Data Storage and Retrieval:
- Hash Tables in Caching: Web browsers and applications often leverage hash tables to cache frequently accessed data (e.g., user preferences, website assets). This allows for lightning-fast retrieval of cached data, improving user experience by reducing server load and minimizing network requests.
- Self-Balancing Trees in Contact Management: Contact management applications commonly utilize self-balancing trees to store and manage user contacts. This ensures efficient search and retrieval of contacts by name, even for extensive contact lists, enhancing user experience and efficiency.
System Performance Improvement:
- B-Trees in File Systems: Modern File Systems make good use of B-trees in their File Allocation and Directory structures. It makes the overall storage strategy optimized for better organization and fast retrieval of files, hence increasing the overall File Performance and Speed of accessing data.
- Red-Black Trees in Network Routing: Network routers frequently employ red-black trees to maintain routing tables. This ensures efficient lookup of destination networks, allowing for faster packet forwarding and optimized network performance.
Conclusion:
The journey to mastering data structures is ongoing. Embrace the exploration! Experiment with different techniques, delve into research papers, and tackle coding challenges to solidify your learning and apply your knowledge. By mastering these techniques, you can choose the optimal data structure for your program, optimizing both memory usage and processing speed. Furthermore, understanding advanced analysis methods like amortized analysis empowers you to evaluate long-term efficiency.
About the Author
Ms. Sarah Lopez
Qualification: Master's degree in Computer Science and Artificial Intelligence
Expertise: Skilled in machine learning, programming languages, and software development.
Research Focus: Specializes in applying machine learning techniques to automate software development tasks, including code generation, testing, and debugging.
Practical Experience: Worked with research teams to develop and evaluate machine learning models for automating software development processes, improving efficiency and reducing human error.
Ms. Lopez is a passionate developer with a strong interest in AI, leveraging machine learning to streamline the software development lifecycle.