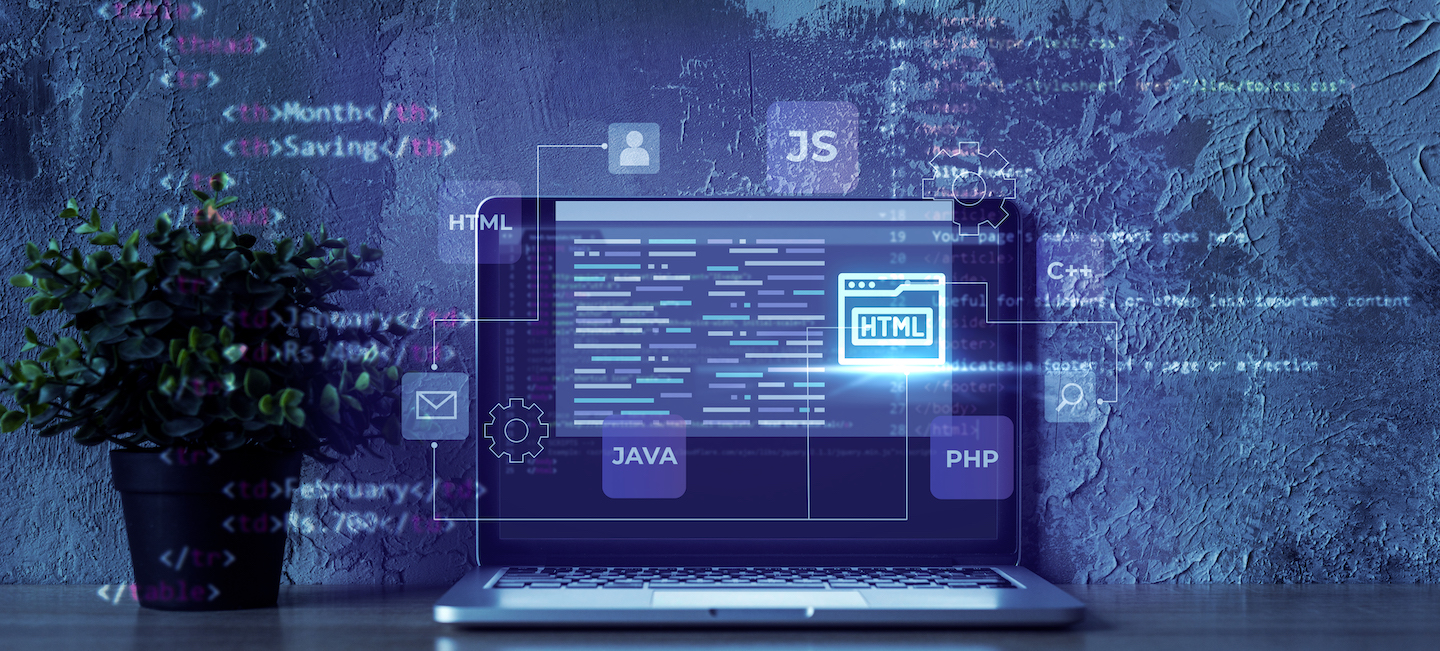
- 19th Dec 2023
- 14:58 pm
- Admin
The Document Object Model (DOM) is an important concept in making websites. It helps arrange the content of a webpage in an organized way, like a tree. It acts as a live connection for scripts, usually in JavaScript, to talk to and modify the content, design, and structure of a webpage.
Think of the DOM as a tool that turns a webpage written in HTML or XML into a tree structure. Each part of the page, like elements, attributes, and text, becomes like an object in this tree. This makes it easier for programmers to work with and change what's on the webpage. The nesting and hierarchy of the HTML or XML code are reflected in the structure, with the document serving as the root of the tree.
Key Features of the Document Object Model in JavaScript
The Document Object Model has the following key features:
- Structure of the Tree:
A web page is represented by the DOM as a tree structure, with nodes representing elements, attributes, and text. The document node serves as the tree's root, from which it branches out to include elements, which can contain more elements, producing a hierarchical structure.
- Dynamic Interaction:
The DOM is really helpful for making web pages interactive. With JavaScript, you can easily reach, change, add, or take away things like elements and content on a webpage. This helps in making web pages that users can interact with and respond to.
- Platform Independence:
Because the DOM provides a platform-independent interface, developers may design scripts that work reliably across multiple browsers. This layer protects developers from browser-specific implementation details, enabling cross-browser compatibility.
- Event Handling:
Scripts may respond to user activities such as clicks, keypresses, or mouse movements thanks to the DOM. This allows for the development of interactive and engaging online apps.
- Styles and Attributes Manipulation:
JavaScript can be used to dynamically change the styles and attributes of HTML components. This functionality is critical for designing dynamic user interfaces, incorporating animations, and changing the visual display of web information in real-time.
- Document Traversal:
The DOM has methods for traversing the document tree, allowing developers to move from one section of the page to another. This navigation ability is essential for quickly identifying and modifying specific parts.
Knowing the DOM is super important for web developers. It helps them create web apps that are lively, responsive, and can interact with users. The DOM forms the base for scripting on the user's side in today's web development. This includes things like changing page content when users do something, handling events, and making elements look different in real-time.
Document Object Model Applications
The Document Object Model (DOM) is a big deal in web development. It gives a neat way to organize a document's content and lets you play with HTML, XML, and XHTML files. Let's see how people use the DOM in programming:
- Dynamic Content Modification:
The DOM enables developers to change the content of a web page dynamically. JavaScript can be used to add, remove, or modify HTML elements, attributes, and text content, allowing the user interface to be updated in response to user actions or external events.
- Interactive User Interfaces:
Developers can use the DOM to construct interactive and responsive user interfaces. Clicks, key presses, and mouse movements can be recognised and handled, allowing for the development of features such as form validation, interactive menus, and dynamic content updates.
- Data Binding:
The DOM makes data binding easier by allowing developers to associate data with HTML components. This relationship between data and the DOM enables the user interface to be automatically updated when the underlying data changes. This is especially handy in frameworks such as React and Angular.
- AJAX (Asynchronous JavaScript and XML) Requests:
AJAX allows web pages to make asynchronous server requests, fetching or transmitting data without reloading the full page. The DOM is essential in processing received data and dynamically modifying page content, resulting in a smoother and more responsive user experience.
- Animation and Effects:
Developers can build animations and visual effects on web pages by combining the DOM with CSS and JavaScript. Elements can be modified over time to change their positions, sizes, and styles, improving the overall user experience.
- Document Traversal:
The DOM has methods for traversing and exploring the document tree. This is required for locating specific elements inside the document structure, retrieving their properties, and performing operations based on their relationships.
The Document Object Model is crucial in web programming because it allows developers to create dynamic, interactive, and responsive web applications by changing the structure and content of HTML documents using client-side scripting.
Structure of The document Object Model in JavaScript
The Document Object Model (DOM) is a tree-like structure that represents the structure of an HTML or XML document. This hierarchical organisation reflects the nesting and relationships between the document's many elements and nodes. Here's a summary of the DOM's basic structure:
- Document Node: At the very top of the DOM tree is the document node. This node stands for the whole content of HTML or XML. It's like the starting point for doing things with the structure and content of the document.
- Element Nodes: Element nodes in a document represent HTML or XML elements. Each HTML tag, such as 'div>', 'p>', or'span>', corresponds to a DOM element node. components can nest inside other components to produce a nested structure.
- Attribute Nodes: Attribute nodes represent HTML or XML element attributes. The "id," "class," and "src" attributes, for example, are represented as nodes in the DOM. The attribute nodes are connected to the element nodes.
- Text Nodes: Text nodes are used to represent text information within HTML or XML elements. A text node in the DOM represents any plain text or spaces within an element, such as the text between 'p>' and '/p>'.
- Comment Nodes: Comment nodes are used to represent HTML or XML comments within a document. Comments are enclosed by '!--' and '-->' and are considered as distinct nodes in the DOM tree.
- Document Object: A specific object that represents the full document is the 'document' object. It includes methods and attributes for interacting with the document, such as adding items, editing content, and managing events.
- Node Relationships: DOM nodes are linked together by parent-child relationships. An element node, for example, is a child of its parent element node and can have child nodes of its own. Sibling nodes have the same parent and are at the same level in the hierarchy.
- Root: The root of the DOM tree is the `document` object. From here, developers can traverse the tree, accessing and manipulating various elements, attributes, and text content.
Example of The Document Object Model in JavaScript
Here's a simplified representation of the DOM structure for a simple HTML document:
```plaintext
- Document (document node)
- Element:
- Element:
- Element:
- Text: "Document Title"
- Element:
- Element:
- Text: "Hello, World!"
- Element:
- Text: "This is a paragraph."
```
The tree in this example begins with the document node, then the '
' element, and so on, showing the hierarchical structure of the HTML page in the DOM.